How to Get Class in jQuery
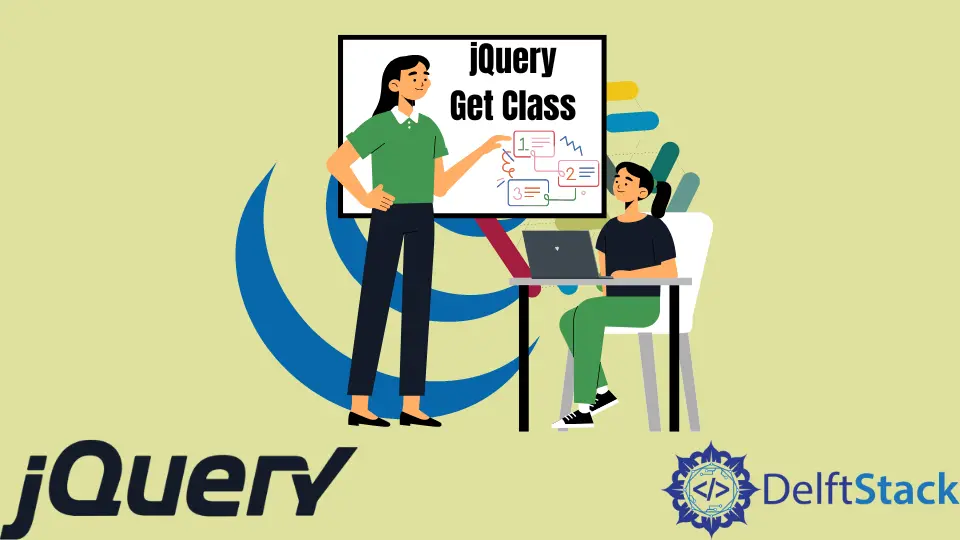
This tutorial demonstrates how to get the class name in jQuery.
jQuery Get Class
The attr()
method can get the class name in jQuery. Besides this method, the hasClass()
method can be used to check if the particular element has a particular class name.
Let’s describe and show examples for both methods.
The attr()
method is used to get the value of HTML element attributes. We can use the class name in the attr()
method as a parameter to get the name of the class.
The syntax to get the class name using the attr
method is below.
attr('class')
Let’s try an example of this method.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Get Class Name</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
var Name = $("#DELFTSTACK").attr("class");
alert(Name);
});
});
</script>
</head>
<body>
<div id="DELFTSTACK" class="Delfstack">Click the button to get the class name for this element.</div>
<button type="button">Click Here</button>
</body>
</html>
The code above will alert the class name of the given element. See output:
Let’s check if the class has the name we got from the attr()
method. For this purpose, we can use the hasClass()
method.
See example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Get Class Name</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
var Name = $("#DELFTSTACK").attr("class");
if ($( "#DELFTSTACK" ).hasClass( Name )){
alert(Name);
}
});
});
</script>
</head>
<body>
<div id="DELFTSTACK" class="Delfstack">Click the button to get the class name for this element.</div>
<button type="button">Click Here</button>
</body>
</html>
The code above will have similar output because the attr
method will return us the correct class name, and the hasClass
will return true
. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook