jQuery Dialog
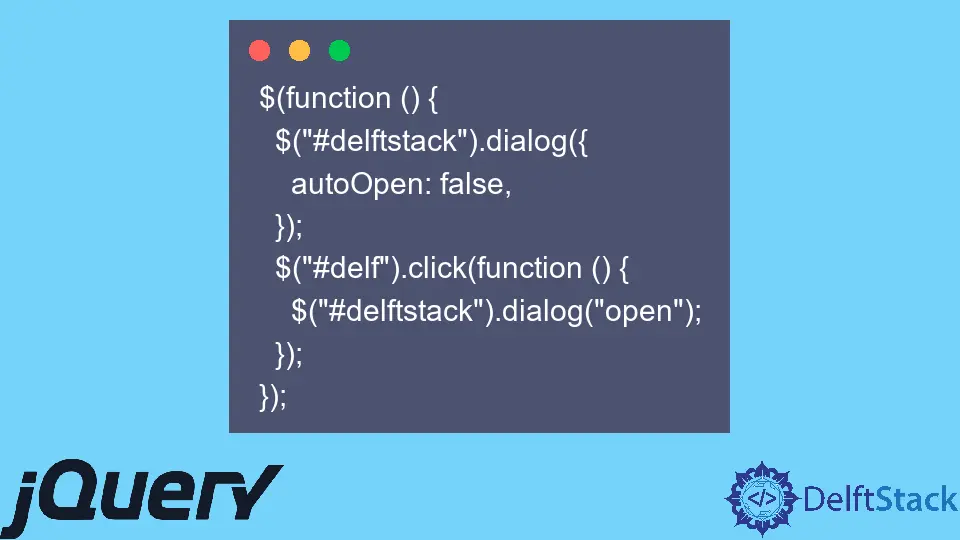
In today’s post, we’ll learn about dialog
and its various options in jQuery.
Dialog in jQuery
The basic dialog box is an overlay placed inside the viewport and shielded from page content (e.g., select
items) showing through an iframe. It has a title
bar and content area and may be moved, resized, and closed with the x
icon by default.
A scroll bar automatically appears if the content length exceeds the maximum height.
We can add options like a bottom button bar and a semi-transparent modal overlay layer. Some options that can be used with dialog
are below.
-
autoOpen
- a Boolean value; if this value is set totrue
, the dialog will be automatically opened on initialization. Iffalse
, the dialog remains hidden until theopen()
method is called. -
closeOnEscape
- a Boolean value that indicates whether to close the dialog box when it focuses and the user presses the Esc key. -
closeText
- a string value that specifies the text for the close button. When using a default theme, the closing text is visibly hidden. -
draggable
- a Boolean value; if set totrue
, the dialog can be dragged from thetitle
bar. This requires the jQuery UIdraggable
widget to be imported. -
modal
- a Boolean value. If set totrue
, the dialog has modal behavior.Other page elements are deactivated, i.e., you cannot interact. Modal dialogs create an overlay under the dialog; however, above different elements on the web page.
You can find more information in the documentation for dialog
.
Let’s understand it with the following example.
<script src="https://code.jquery.com/ui/1.13.1/jquery-ui.js"></script>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.1/themes/base/jquery-ui.css">
The above two lines need to be imported before implementing dialog. It’s a third-party library that provides dialog functionality.
If it’s not imported, it will throw an error dialog is not a function
.
<button id="delf">Open Dialog</button>
<div id="delftstack" title="Welcome to Delftstack">
Welcome to Delftstack
</div>
$(function() {
$('#delftstack').dialog({
autoOpen: false,
});
$('#delf').click(function() {
$('#delftstack').dialog('open');
});
});
Within the above example, we have defined the button which will open a dialog. By default, a dialog will be closed.
Once you click the button, the dialog will trigger the open
method, visible on the screen.
Attempt to run the above code snippet in any browser that supports jQuery; it will display this result.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn