How to Format Date and Time Using DateTimePicker in jQuery
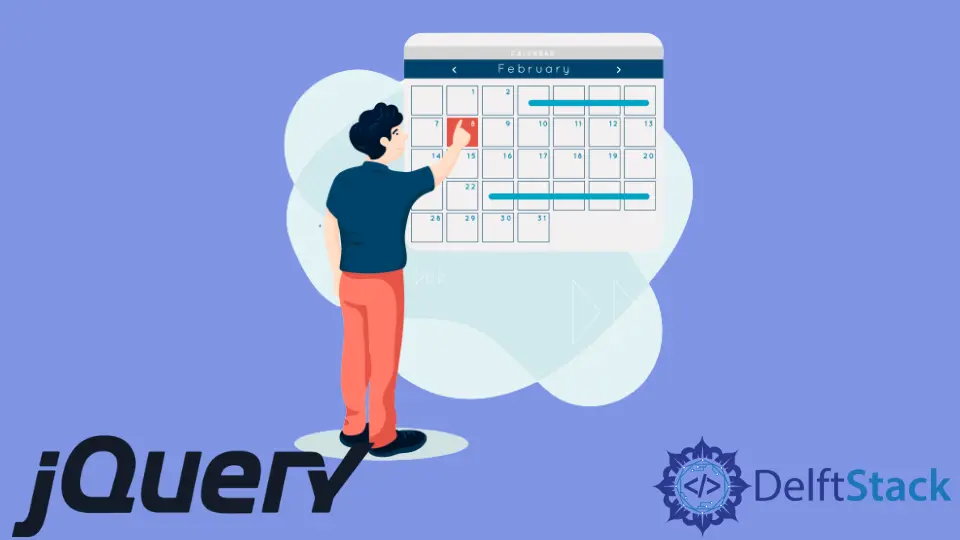
If you want to pick dates only, you should use a jQuery plugin called DatePicker. But sometimes, you must select both dates and times, so you must use the jQuery plugin called DateTimePicker.
This article will use DateTimePicker to format the date.
Format Date and Time Using DateTimePicker in jQuery
The DateTimePicker provides the ability to write a date and time value that will appear in the datetimepicker
control.
To use DateTimePicker, first, you need to download the jQuery plugin. You can download it from here.
After downloading, you have to extract those files.
You do not have to use all the files. You must only use the jquery.js
, jquery.datetimepicker.full.min.js
, and jquery.datetimepicker.min.css
files.
Remember that you have to place these files in the same directory. You can get jquery.js
in the main folder and the remaining files in the build
directory.
To format dates in the jQuery DateTimePicker, change the format property inside the item sent to the datetimepicker
function.
For example, we could write the following. To run this code, you need to save the file as an HTML file and run it in the browser.
<!DOCTYPE html>
<html>
<head>
<link
rel="stylesheet"
type="text/css"
href="jquery.datetimepicker.min.css"
/>
<script src="jquery.js"></script>
<script src="jquery.datetimepicker.full.min.js"></script>
</head>
<body>
<input id="datetimepicker" type="text" />
<script>
$("#datetimepicker").datetimepicker({
format: "d/m/Y H:m:s",
});
</script>
</body>
</html>
Remember: The file jquery.js
should be included above the jquery.datetimepicker.full.min.js
.
We have the input
element for the date and time picker, including the jQuery script, link tags, and the jQuery datetimepicker
. Then, we call datetimepicker
with an entity to modify the format property to d/m/Y
so that the better fit appears in the input in DD-MM-YYYY
format.
Here, d
represents the date, m
represents the month, and Y
represents the year. Likewise, H
stands for the hour, m
for the minutes, and s
stands for the seconds.
Output:
Here, the date-time format is in d/m/Y
and H:m:s
. You can change the input format by passing your date and time formats.
In summary, to format dates using the jQuery DateTimePicker, change the format property in the object sent into the datetimepicker
function.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn