Date Format in jQuery
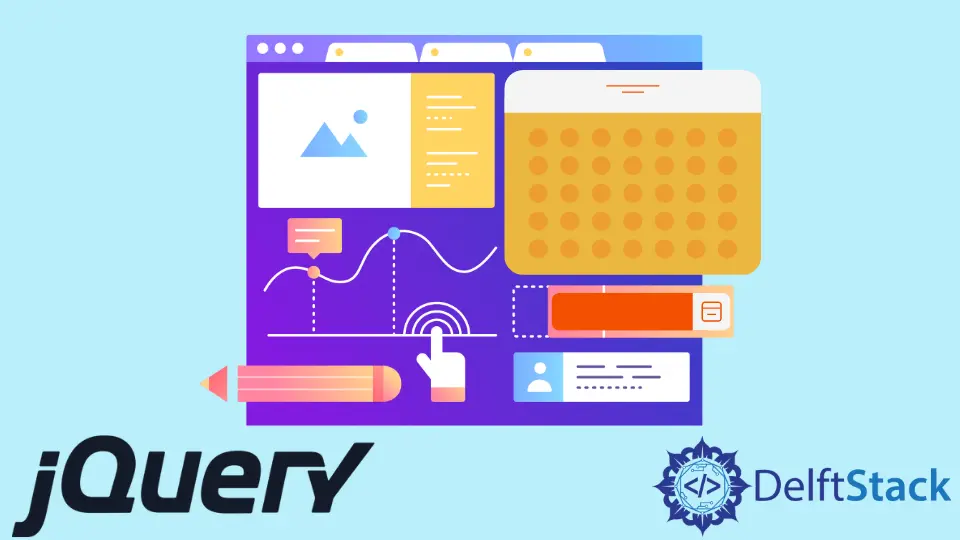
JavaScript date
objects represent a single time factor in a platform-neutral format. Date items contain numbers representing milliseconds since January 1, 1970, UTC.
A new Date()
is known as it returns a new Date item. The Date()
is referred to as it returns a string illustration of the present-day date and time.
In this quick tutorial, we’ll learn about how to format dates in jQuery.
Date Format in jQuery
jQuery provides various plugin which is used to format dates. The most popular ones are moment and dateFormat
. We will use both the plugins and format the date in our example in this tutorial.
The jQuery-dateFormat
is a jQuery Plugin for dates to format the outputs using JavaScript. The size of the library is less than 5kb; hence jquery-dateFormat
is the smallest date format library available.
The jQuery-moment
is a lightweight JavaScript date library for parsing, validating, manipulating, and formatting dates. Instead of extending the Date object, Moment.js
builds a wrapper for it.
Call the moment()
getter function to reference the container object. The Moment prototype is exposed via the moment.fn
property so that you can add your functions to it.
Example Code:
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.29.3/moment.min.js" integrity="sha512-x/vqovXY/Q4b+rNjgiheBsA/vbWA3IVvsS8lkQSX1gQ4ggSJx38oI2vREZXpTzhAv6tNUaX81E7QBBzkpDQayA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-dateFormat/1.0/jquery.dateFormat.min.js"></script>
console.log("using dateFormat", $.format.date("2021-12-18 12:12:19.546", "dd/MM/yyyy"))
console.log("using moment", moment("2021-12-18 12:12:19.546").format("DD-MM-yyyy"))
We’ve imported the min.js
file for moment.js
and dateFormat
within the above example. Once the import has finished, you can use the functions of both the library and format the date according to the requested formats.
Run the above code snippet in any browser that supports jQuery.
Output:
"using dateFormat", "18/12/2021"
"using moment", "18-12-2021"
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn