How to Check if a String Contains a Substring in jQuery
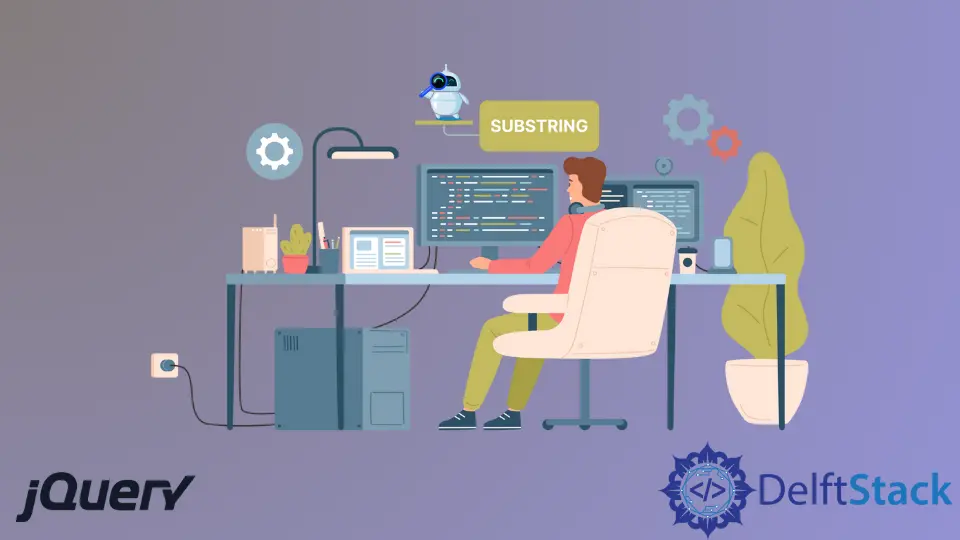
The contains()
method in jQuery can check whether a DOM element contains another DOM element. This tutorial demonstrates how to use the contains()
method in jQuery.
Use the contains()
Method to Check if a String Contains a Substring in jQuery
The jQuery contains()
method checks if a string contains a substring or a character. The syntax for this method is:
jQuery.contains( container, contained )
Where:
- the
container
is the DOM element that contains another DOM element, and - the
contained
in the sub-element.
The method returns a Boolean value.
The syntax for contains()
can also be written as:
container:contains(contained)
Let’s try an example:
<!DOCTYPE html>
<html>
<head>
<title> jQuery Contains Method </title>
<script src="https://code.jquery.com/jquery-3.4.1.js"></script>
<script>
$(document).ready(function(){
$("p:contains(is)").css("background-color", "lightgreen");
});
</script>
</head>
<body>
<h1>Welcome to Delfstack</h1>
<p>DelftStack is a resource for everyone interested in programming,.</p>
<p>embedded software, and electronics. It covers the programming languages like</p>
<p> Python, C/C++, C#, and so on in this website's first development stage</p>
</body>
</html>
The code above will check if the string in the paragraph element contains a substring or a character and change the background color of that paragraph. See output:
This method can also tell us if a certain HTML element contains another element. See the example below:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JQuery contains() method</title>
<script src= "https://code.jquery.com/jquery-3.4.1.js"> </script>
</head>
<body>
<h3>JQuery contains() method</h3>
<b id="delftstack"></b>
<script>
$("#delftstack").append( "Does 'document.body' contains 'document.documentElement'? <br>"
+ $.contains(document.documentElement, document.body));
</script>
</body>
</html>
The code above will check if the document body contains some elements and if it contains, the method will return true. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook