How to Animate Scrolling in jQuery
- Understanding jQuery’s Animate Method
- Basic jQuery Scroll Animation
- Smooth Horizontal Scrolling
- Adding Easing Effects
- Creating Scroll Spy Navigation
- Conclusion
- FAQ
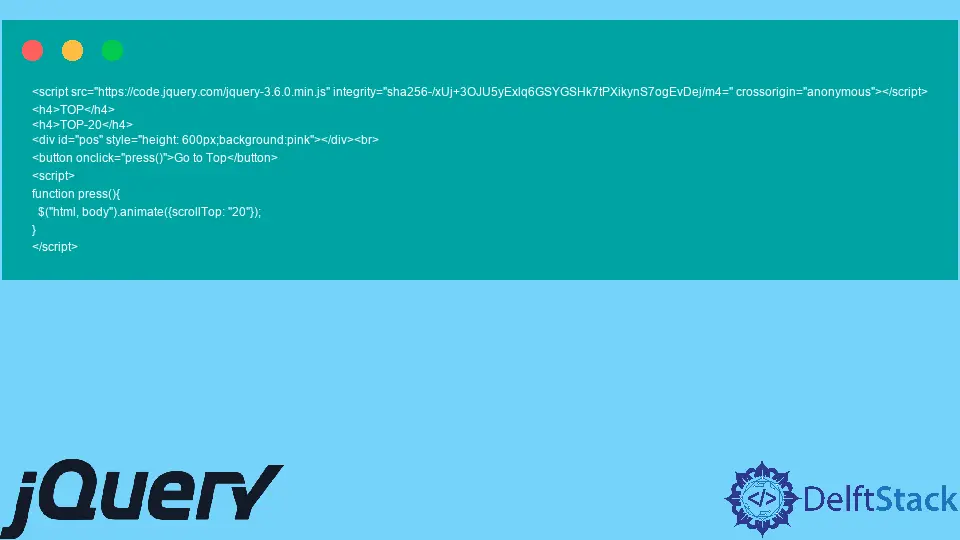
Animating scrolling is a powerful feature that enhances user experience on websites. With jQuery’s animate()
method, you can smoothly scroll to specific vertical or horizontal positions with just a few lines of code. This technique is not only simple to implement but also adds a professional touch to your web pages. Whether you’re creating a single-page application or a long-scroll website, mastering scrolling animation can help keep your visitors engaged.
In this article, we’ll explore how to animate scrolling using jQuery, providing clear code examples and explanations to ensure you can easily incorporate this feature into your projects.
Understanding jQuery’s Animate Method
Before diving into the code, it’s essential to understand what the animate()
method does. This method allows you to create custom animations for CSS properties of selected elements. When applied to scrolling, it can smoothly transition the viewport to a designated position on the page. The beauty of jQuery is in its simplicity and versatility, making it an excellent choice for developers of all skill levels.
Basic jQuery Scroll Animation
To get started with scroll animation, you’ll need to include jQuery in your project. You can either download it or link it directly from a CDN. Once jQuery is set up, you can use the following code snippet to create a basic scroll animation.
$(document).ready(function() {
$('a.scroll-link').click(function(event) {
event.preventDefault();
$('html, body').animate({
scrollTop: $(this.hash).offset().top
}, 800);
});
});
In this example, we first wait for the document to be fully loaded. The click event is then attached to any anchor tag with the class scroll-link
. When one of these links is clicked, the default action is prevented, and jQuery’s animate()
method is invoked. The scrollTop
property is set to the vertical offset of the target element (identified by the hash in the link). The duration of the animation is set to 800 milliseconds, which strikes a good balance between speed and smoothness.
The complete HTML code is below.
<!DOCTYPE html>
<html>
<head>
<title>Vertical Scroll Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<style>
body {
margin: 0;
padding: 0;
}
.section {
height: 600px; /* Example section height, adjust as needed */
width: 100%;
background-color: #f0f0f0;
border-bottom: 1px solid #ccc;
text-align: center;
line-height: 600px;
font-size: 24px;
}
</style>
</head>
<body>
<a href="#section1" class="scroll-link">Go to Section 1</a>
<a href="#section2" class="scroll-link">Go to Section 2</a>
<a href="#section3" class="scroll-link">Go to Section 3</a>
<div id="section1" class="section">Section 1</div>
<div id="section2" class="section">Section 2</div>
<div id="section3" class="section">Section 3</div>
<script>
$(document).ready(function() {
$('a.scroll-link').click(function(event) {
event.preventDefault();
$('html, body').animate({
scrollTop: $(this.hash).offset().top
}, 800);
});
});
</script>
</body>
</html>
Smooth Horizontal Scrolling
While vertical scrolling is common, horizontal scrolling can also be useful, especially for galleries or product showcases. To animate horizontal scrolling, you can modify the previous example slightly.
$(document).ready(function() {
$('a.scroll-horizontal').click(function(event) {
event.preventDefault();
$('html, body').animate({
scrollLeft: $(this.hash).offset().left
}, 800);
});
});
In this case, we use scrollLeft
instead of scrollTop
. The logic remains the same: we prevent the default action of the anchor tag and animate the scroll position to the horizontal offset of the target element. This method can be particularly effective for creating a seamless user experience on sites with horizontal content.
The complete HTML code:
<!DOCTYPE html>
<html>
<head>
<title>Horizontal Scroll Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<style>
body {
white-space: nowrap; /* Prevent vertical wrapping */
overflow-x: auto; /* Enable horizontal scrolling */
width: 3000px; /* Example width, adjust as needed */
}
.section {
display: inline-block; /* Arrange sections horizontally */
width: 800px; /* Example section width, adjust as needed */
height: 500px; /* Example section height, adjust as needed */
margin: 20px;
background-color: #f0f0f0;
border: 1px solid #ccc;
text-align: center;
line-height: 500px;
font-size: 24px;
}
</style>
</head>
<body>
<a href="#section1" class="scroll-horizontal">Go to Section 1</a>
<a href="#section2" class="scroll-horizontal">Go to Section 2</a>
<a href="#section3" class="scroll-horizontal">Go to Section 3</a>
<div id="section1" class="section">Section 1</div>
<div id="section2" class="section">Section 2</div>
<div id="section3" class="section">Section 3</div>
<script>
$(document).ready(function() {
$('a.scroll-horizontal').click(function(event) {
event.preventDefault();
$('html, body').animate({
scrollLeft: $(this.hash).offset().left
}, 800);
});
});
</script>
</body>
</html>
Adding Easing Effects
To make your scrolling animations even more engaging, consider adding easing effects. Easing functions can change the speed of the animation at different points in its duration, making the scroll feel more natural. jQuery provides several built-in easing options, and you can also include additional easing libraries for more customization.
$(document).ready(function() {
$('a.scroll-link-ease').click(function(event) {
event.preventDefault();
$('html, body').animate({
scrollTop: $(this.hash).offset().top
}, 800, 'easeInOutExpo');
});
});
In this example, we specify the easing type as easeInOutExpo
, which provides a smooth start and end to the animation. To use this easing function, ensure you include the jQuery UI library or another easing plugin in your project. This small addition can significantly enhance the user experience by making the transitions feel more fluid.
Creating Scroll Spy Navigation
A scroll spy navigation is a great way to inform users about their position on a long page. By highlighting the current section in the navigation menu, you can guide users seamlessly through your content. Here’s how to implement it using jQuery.
$(document).ready(function() {
$(window).scroll(function() {
var scrollPos = $(document).scrollTop();
$('.nav-link').each(function() {
var currLink = $(this);
var refElement = $(currLink.attr("href"));
// Use offset().top instead of position().top for accurate calculation
if (refElement.offset().top <= scrollPos + $(window).height() / 2 &&
refElement.offset().top + refElement.outerHeight() > scrollPos + $(window).height() / 2) {
$('.nav-link').removeClass("active");
currLink.addClass("active");
} else {
currLink.removeClass("active");
}
});
});
});
In this code, we listen for the scroll event on the window. As the user scrolls, we check the position of each navigation link against the current scroll position. If the link’s corresponding section is in view, we add the active
class to the link, which can be styled with CSS to highlight it. This feature not only improves navigation but also enhances the overall user experience.
The complete HTML code is below.
<!DOCTYPE html>
<html>
<head>
<title>Scroll Spy Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<style>
body {
margin: 0;
padding: 0;
}
nav {
position: fixed;
top: 0;
width: 100%;
background-color: #333;
overflow: hidden;
}
nav a {
float: left;
display: block;
color: white;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
nav a.active {
background-color: #4CAF50;
}
.section {
height: 800px; /* Example section height, adjust as needed */
width: 100%;
background-color: #f0f0f0;
border-bottom: 1px solid #ccc;
text-align: center;
line-height: 800px;
font-size: 24px;
}
</style>
</head>
<body>
<nav>
<a href="#section1" class="nav-link">Section 1</a>
<a href="#section2" class="nav-link">Section 2</a>
<a href="#section3" class="nav-link">Section 3</a>
</nav>
<div id="section1" class="section">Section 1</div>
<div id="section2" class="section">Section 2</div>
<div id="section3" class="section">Section 3</div>
<script>
$(document).ready(function() {
$(window).scroll(function() {
var scrollPos = $(document).scrollTop();
$('.nav-link').each(function() {
var currLink = $(this);
var refElement = $(currLink.attr("href"));
// Use offset().top instead of position().top for accurate calculation
if (refElement.offset().top <= scrollPos + $(window).height() / 2 &&
refElement.offset().top + refElement.outerHeight() > scrollPos + $(window).height() / 2) {
$('.nav-link').removeClass("active");
currLink.addClass("active");
} else {
currLink.removeClass("active");
}
});
});
});
</script>
</body>
</html>
Conclusion
Animating scrolling in jQuery is an effective way to enhance your website’s usability and aesthetic appeal. By implementing smooth vertical and horizontal scrolling, adding easing effects, and creating scroll spy navigation, you can create a more engaging experience for your users. The flexibility of jQuery allows you to customize these animations to fit your specific needs, making it a valuable tool for any web developer. So, go ahead and experiment with these techniques to elevate your web projects.
FAQ
-
How do I include jQuery in my project?
You can include jQuery by linking to a CDN in your HTML file or downloading it and hosting it locally. -
Can I customize the duration of the scroll animation?
Yes, you can change the duration by adjusting the number in theanimate()
method, which is specified in milliseconds. -
What is the benefit of using easing effects?
Easing effects make animations feel more natural and smooth, enhancing the overall user experience. -
Is it possible to animate multiple elements at once?
Yes, you can select multiple elements using jQuery selectors and apply theanimate()
method to them simultaneously. -
Can I use jQuery animations on mobile devices?
Yes, jQuery animations are compatible with most mobile devices, but make sure to test for performance on lower-end devices.