jQuery addEventListener
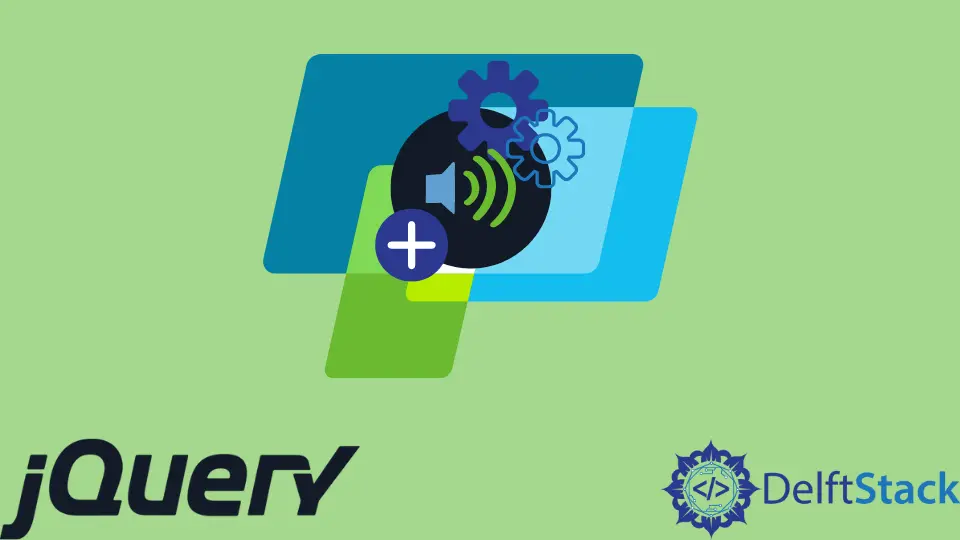
In today’s post, we’ll learn about the addEventListener
function in jQuery.
AddEventListener
in jQuery
JavaScript provides the EventTarget
interface’s built-in addEventListener()
method. This method configures a function to be called each time the specified event is sent to the target.
jQuery provides an equivalent method called .on()
. Similar to the addEventListener
method of JavaScript, the .on
method attaches an event handler function to the selected elements for one or more events.
Syntax:
.on(events[, selector][, data], handler)
Events
is a string representing one or more event types, separated by spaces and optional namespaces, e.g.,Click
orkeydown.myPlugin
.- The
selector
represents a selection/matching string to filter the descendants of the chosen elements that trigger the event. If theselector
is not passed ornull
, the event is dispatched each time the selected element is reached. Data
is passed to the controller in theevent.data
when an event is raised.handler
is a function executed when the event is raised. If thehandler
function returnsnull
, afalse
value is also allowed as a shortcut.
To the currently selected items, jQuery’s .on()
method attaches event handlers to the object. The .on()
method gives all the functionality to attach event handlers.
Any event name can be used for the event argument. jQuery iterates/loops through the browser’s standard JavaScript event types and invokes the handler
function when the browser fails due to user actions, e.g., .click
, which triggers events.
The .trigger()
technique can also cause standard browser event names and custom event names to invoke connected handlers. Event names must incorporate the best alphanumeric characters, underscores, and colons.
Let’s understand it with the following simple example.
<button id="btn">Hello World!</button>
$('#btn').on('click', () => {console.log('Click event is fired.')})
In the above code snippet, we have defined the button. Once the user clicks the button, the click
event is dispatched, and the handler
function is invoked.
This is similar to the .click()
method, which internally triggers the on('click')
method.
Try running the code snippet above in any browser that supports jQuery. The following result is displayed.
"Click event is fired."
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn