How to Add Elements to Array Using jQuery
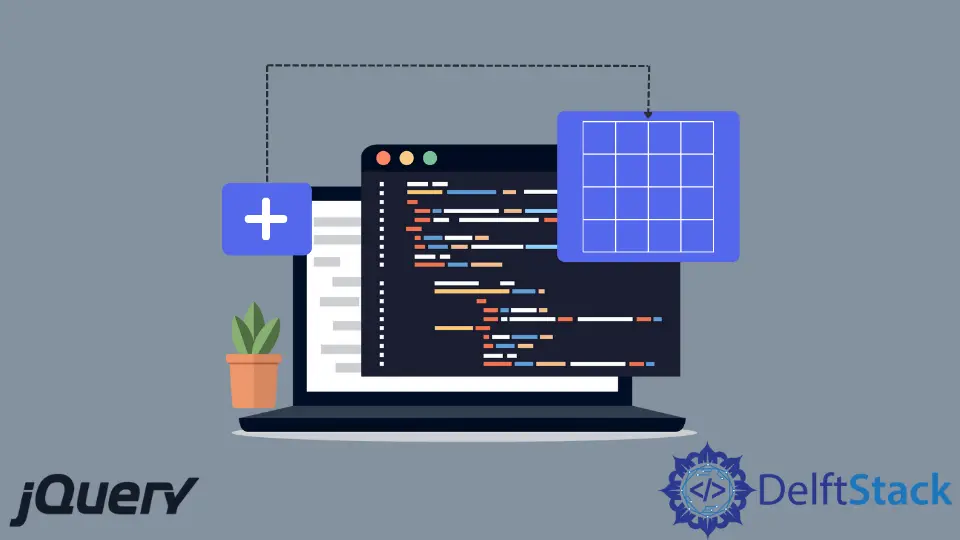
Adding elements to an array is a fundamental operation in programming, and jQuery makes it simple and efficient. Whether you are manipulating data for a web application or managing user inputs, knowing how to effectively add elements to an array can enhance your coding skills.
In this article, we will explore two primary methods to add elements to an array in jQuery: the push()
method and the merge()
method. We will provide practical code examples to illustrate each method, making it easier for you to apply these techniques in your own projects. Let’s dive in and learn how to manage arrays like a pro!
Using the push()
Method
The push()
method is one of the most commonly used techniques for adding elements to an array in jQuery. This method allows you to append one or more elements to the end of an existing array. It modifies the original array and returns the new length of the array. Here’s how you can use it effectively.
let fruits = ['apple', 'banana', 'orange'];
fruits.push('grape');
fruits.push('mango', 'pineapple');
console.log(fruits);
Output:
['apple', 'banana', 'orange', 'grape', 'mango', 'pineapple']
In this example, we start with an array called fruits
containing three initial elements. By calling the push()
method, we add a single element, ‘grape’, and then we add two more elements, ‘mango’ and ‘pineapple’. The push()
method modifies the original array and outputs the updated array, which now includes all the new fruits.
The power of the push()
method lies in its ability to add multiple elements at once, making it efficient for managing dynamic lists. This is particularly useful when dealing with user inputs or data fetched from an API. As you continue to work with jQuery, mastering the push()
method will undoubtedly enhance your ability to manipulate arrays seamlessly.
Using the merge()
Method
Another effective way to add elements to an array in jQuery is by using the merge()
method. This method is particularly useful when you want to combine two arrays into one. It takes two arrays as arguments and returns a new array that contains all the elements from both. Here’s how you can utilize the merge()
method.
let array1 = ['red', 'blue'];
let array2 = ['green', 'yellow'];
let mergedArray = $.merge(array1, array2);
console.log(mergedArray);
Output:
['red', 'blue', 'green', 'yellow']
In this example, we have two arrays, array1
and array2
, each containing different colors. By using the $.merge()
method, we combine both arrays into a new array called mergedArray
. The result is a single array that includes all the colors from both original arrays.
The merge()
method is particularly beneficial when you need to consolidate data from different sources or when you want to combine user selections into a single list. It simplifies the process of managing multiple arrays, allowing you to focus on other aspects of your application. By understanding how to use both the push()
and merge()
methods, you will be well-equipped to handle array operations in jQuery effectively.
Conclusion
Adding elements to an array in jQuery is a straightforward task, thanks to methods like push()
and merge()
. The push()
method allows you to append elements to the end of an array, while the merge()
method helps you combine multiple arrays into one. By mastering these techniques, you can efficiently manage and manipulate data in your web applications. The ability to work with arrays effectively is a crucial skill for any developer, and with practice, you’ll find these methods become second nature.
FAQ
-
What is the difference between push() and merge() in jQuery?
push() adds individual elements to the end of an array, while merge() combines two arrays into one. -
Can I use push() to add multiple elements at once?
Yes, you can add multiple elements in one call by passing them as separate arguments to thepush()
method. -
Does merge() modify the original arrays?
No, merge() creates a new array without altering the original arrays. -
Are there any performance considerations when using these methods?
For small arrays, performance is generally not an issue, but for larger datasets, consider the efficiency of your operations. -
Can I use these methods with objects in jQuery?
Thepush()
and merge() methods are specifically designed for arrays; for objects, you would need to use other techniques.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn