How to Add Options to a <Select> Dropdown Using jQuery
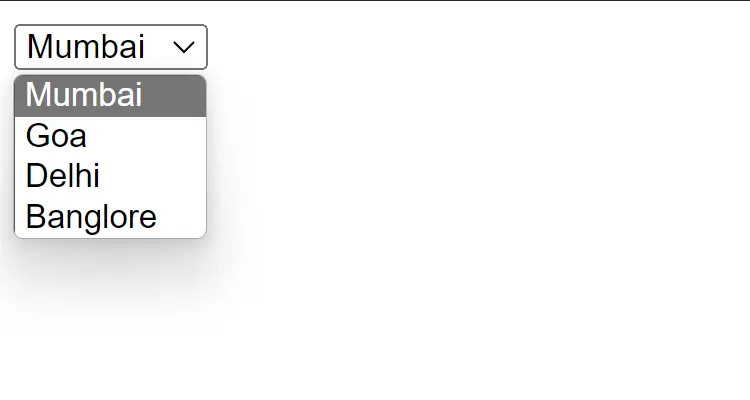
Today’s post will discuss adding new alternative options to the <Select>
element in jQuery.
Add Options to a <Select>
Dropdown Using jQuery
This approach is generally used to add options to the <Select>
form field values. It adds the new HTMLOptionElement
to the prevailing select element based on the criteria matching the distinctive condition.
Syntax:
new Option(text, value)
The new Option()
constructor takes two arguments:
text
- It is an optionally available parameter and a string representing the content of the detail, i.e., the displayed text. If no longer unique, a default value of""
empty string is used.value
- It is an optionally available parameter and a string representing the value of theHTMLOptionElement
, i.e., the value attribute of the<option>
equivalent. If not specified, the text value is used as the value, e.g., for the value of the associated<select>
element when the form is submitted to the server.
Let’s understand it with the following example.
Example code (HTML):
<select id="city">
<option value="mumbai">Mumbai</option>
<option value="goa">Goa</option>
<option value="delhi">Delhi</option>
</select>
Example code (JavaScript):
const o = new Option('Banglore', 'banglore');
$('#city').append(o);
We have described a city
dropdown using the pick within the above example. The default value for the city
dropdown is Mumbai.
To add the new choice, create the instance of the Option
object and add the text
and value
as parameters. Once the instance is created, we will append the instance to the <select>
element.
Attempt to run the above code snippet in any browser that supports jQuery. It’s going to display the beneath result:
Output:
You may access the live demo of the example code above through this link.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn