How to Add Attributes in jQuery
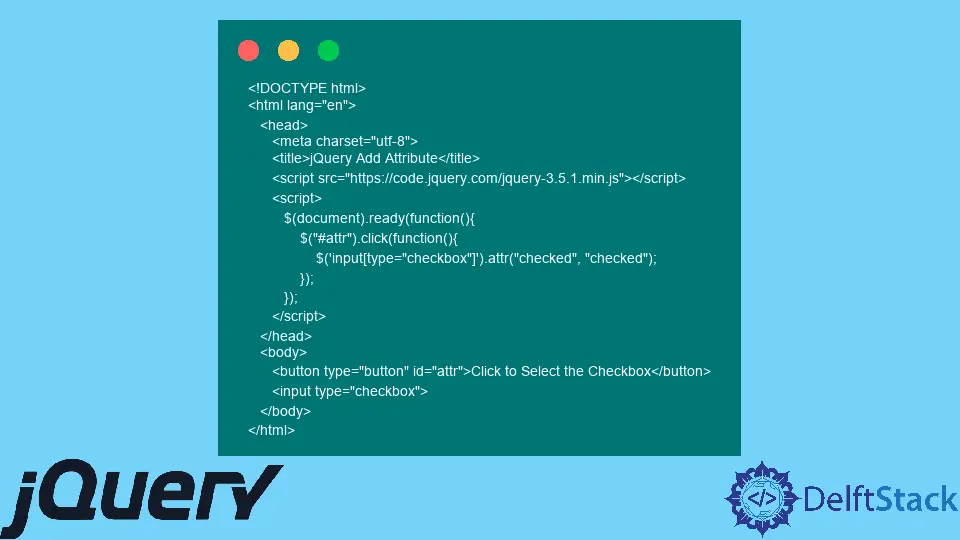
The jQuery attr()
method is used to add attributes to the HTML elements. This tutorial demonstrates how to use attr()
method in jQuery.
jQuery Add Attributes
We can add attributes to the HTML elements using the attr()
method in jQuery. The syntax for this method is given below:
$(selector).attr("property", "Value")
Where:
- The
selector
can be the id, class, or the element’s name. - The
property
is the attribute’s name. - The
value
is the attribute’s value.
Let’s try an example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Add Attribute</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("#attr").click(function(){
$('input[type="checkbox"]').attr("checked", "checked");
});
});
</script>
</head>
<body>
<button type="button" id="attr">Click to Select the Checkbox</button>
<input type="checkbox">
</body>
</html>
The code above will check the unchecked checkbox. The code uses the element name selector with the attr()
method, where the property is checked, and the value is also checked.
See the output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook