How to Handle $.ajax Failure in jQuery
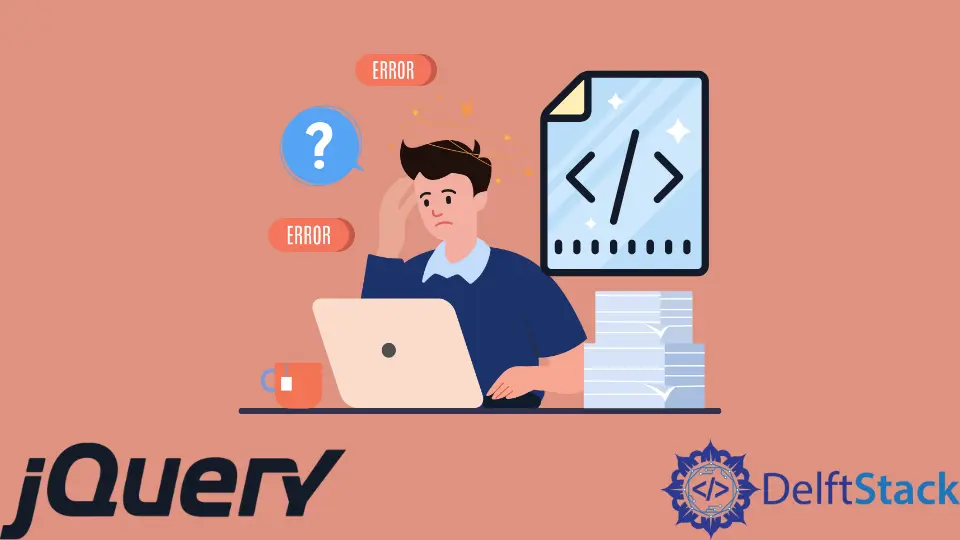
Today’s post will discuss handling failure requests in AJAX in jQuery.
Handle $.ajax
Failure in jQuery
jQuery’s AJAX post request carry out an asynchronous HTTP (AJAX) request.
Syntax:
jQuery.ajax([settings]).fail((jqXHR, textStatus) => {});
jQuery.ajax(url[, settings]).fail((jqXHR, textStatus) => {});
Where:
URL
is the string containing the URL to which the request will be sent.settings
are the key/value pair objects that configure the AJAX request. All settings are optional; a default value can be set for each option with$.ajaxSetup()
.
The .fail()
approach replaces the deprecated .error()
approach. This is an alternative construct to the error callback option.
If the request fails, the error
callback option is called within the AJAX
configuration. It receives the jqXHR
, a string indicating the error type, and an exception object, if any.
Some built-in errors return a string as an exception object, such as: abort
, timeout
, no transport
.
$.ajax()
returns the jQuery XMLHttpRequest
(jqXHR
) object, which is a superset of the browser’s native XMLHttpRequest
object.
Let’s understand it with the following example.
HTML code:
<form id="myForm">
<label for="name">Name</label>
<input id="name" name="name" type="text" value="Smith" />
<input type="submit" value="Send" />
</form>
JavaScript code:
$('#myForm').submit(function(event) {
event.preventDefault();
$.ajax({
method: 'POST',
url: '/open/hello-world',
data: {name: 'Smith', location: 'United State'},
error: function(jqXHR, thrownError) {
alert(jqXHR.status);
alert(thrownError);
}
})
.done(function(msg) {
alert('Data Saved: ' + msg);
})
.fail((jqXHR, errorMsg) => {alert(jqXHR.responseText, errorMsg)});
})
In the example above, once the user submits the form, an AJAX call is sent to the server with the specified URL and parameters. When the server returns with a successful message, you can print the message on the console or notify the user with the appropriate message.
If the server returns with an error message, you can catch the error either using the error handler or .fail()
and notify the user with the appropriate error message.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn