How to Import jQuery
-
Use the
Script
Tag to Import jQuery - Use ES6 Syntax to Import jQuery
- Use Browserify/Webpack to Import jQuery
- Use AMD (Asynchronous Module Definition) to Import jQuery
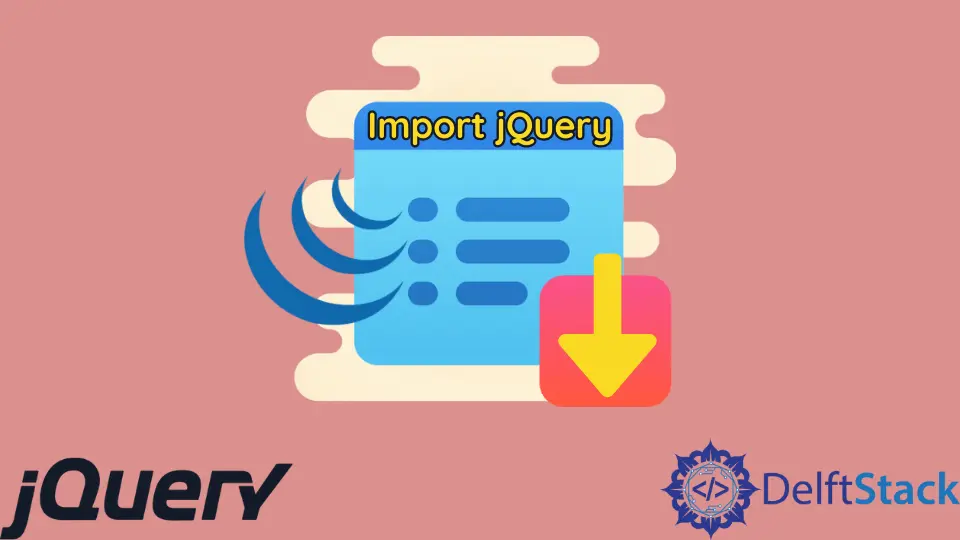
There are several ways to import jQuery using different platforms. This tutorial demonstrates different ways to import jQuery into the environment.
Use the Script
Tag to Import jQuery
This is the most common method for importing the jQuery file in the HTML script
tag. We can either give a link to the source or download the file and give the path to the file in the source.
See example:
Using the link:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Using the downloaded file:
<script src="C:\Users\etc\jquery-3.6.0.min.js"></script>
Both methods are useful in basic jQuery import.
Use ES6 Syntax to Import jQuery
Babel is a next-generation JavaScript compiler. Babel has the functionality to compile ES6 and ES2015 modules of the JavaScript code.
This method is only supported for the compilers like Babel; browsers don’t support ES6/ES2015. See how to import jQuery using ES6 syntax below.
import {$, jQuery} from 'jquery';
// to use jQuery
window.$ = $;
window.jQuery = jQuery;
Or a more simple way:
import jQuery from 'jquery';
window.$ = window.jQuery = jQuery;
Both methods are convenient for compilers like Babel.
Use Browserify/Webpack to Import jQuery
Browserify and Webpack can be used in several ways. We can import jQuery as a variable in Browserify/Webpack.
See example:
var $ = require('jquery');
For further information, the official documentation can be seen below.
Use AMD (Asynchronous Module Definition) to Import jQuery
Asynchronous Module Definition (AMD) is the module format created for the browsers. We can import the jQuery in AMD using a function.
See example:
define(['jquery'], function($) {
// code here
});
For more information on AMD, see here.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook