How to Get Current URL in jQuery
Sundeep Dawadi
Feb 02, 2024
jQuery
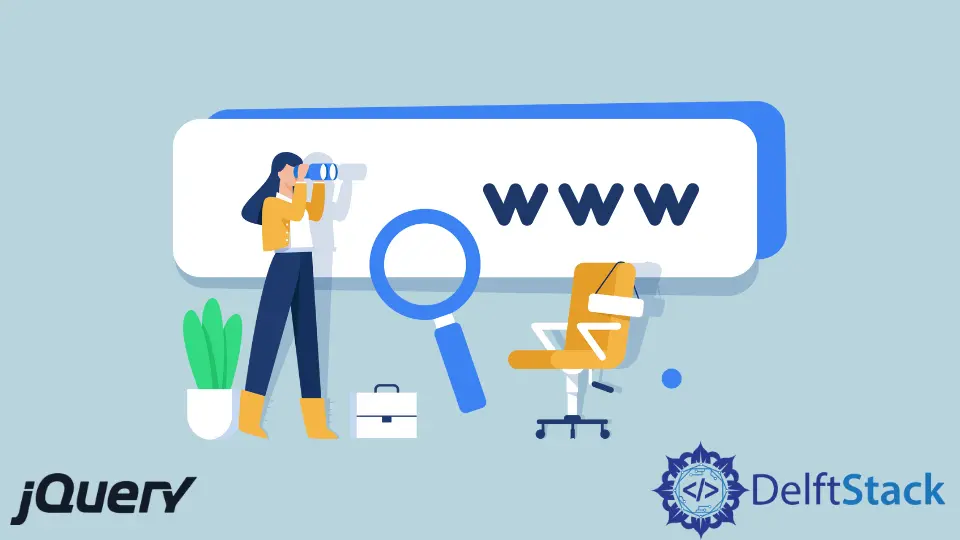
There are often cases when you want to see where your jQuery code is currently loading. For every open window in the browser, the browser gives access to its window
object. As a part of the window
object, the location
object gives information about the current URL.
href
Attribute to Get the Current URL in jQuery
To get the current URL, you can access the href
attribute of the browser’s location
object.
<script>
var url = $(location).attr('href');
// Returns full URL
(https://example.com/path/example.html)
var pathname = $(location).attr('pathname');
// Returns path only (/path/example.html)
var origin = $(location).attr('origin');
// Returns base URL (https://example.com)
</script>
Note
In vanilla JavaScript way, it can be accessed via
window.location.href
.Access Specific Properties of URL in jQuery
The location
Object comes with additional properties of the URL.
Property | Description |
---|---|
hash |
anchor part (#) of a URL |
host |
hostname and port number of a URL |
hostname |
hostname of a URL |
href |
entire URL |
origin |
protocol, hostname, and port number of a URL |
pathname |
pathname of a URL |
port |
port number of a URL |
protocol |
protocol of a URL |
search |
query string part of a URL |
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe