How to Check if an Element Is Hidden in jQuery
- Method to Check if an Element Is Hidden in jQuery
-
Understand the
:hidden
Selector in jQuery - Quick Tips to Check Whether an Element Is Hidden
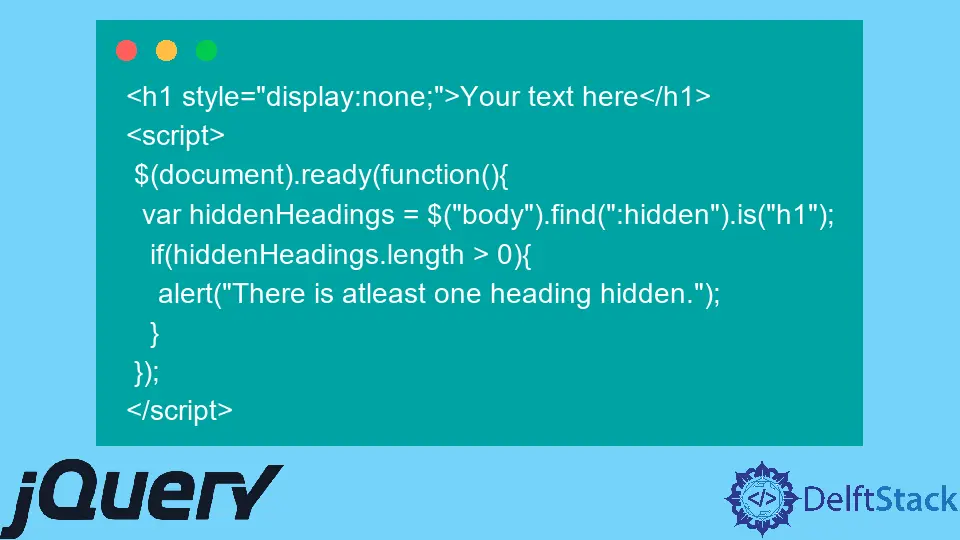
Any object in DOM can be hidden by assigning its CSS property of display
as none
.
Method to Check if an Element Is Hidden in jQuery
First, we create a heading element <h1>
and apply inline CSS property to hide it.
<h1 style="display:none;">Your text here</h1>
In jQuery there are two visibility filters - :hidden
and :visible
selectors. They specify if the element is hidden and visible, respectively. It can be easily checked by the following method.
<script>
$(document).ready(function(){
//checks if document is ready
if($("h1").is(":hidden")){
alert("The heading is hidden.");
//your function here
}
if($("h1").is(":visible"){
alert("The heading is visible.");
}
});
</script>
It gives an alert saying, The heading is hidden.
is(":visible")
and is(":hidden")
.Understand the :hidden
Selector in jQuery
The :hidden
selector identifies an element as hidden for the following reasons:
- It has CSS
display
property with a value ofnone
. - The element is a form element with
type="hidden"
. - The
height
andwidth
values of the element is set explicitly to 0. - The parent element is hidden.
So, elements with CSS visibility
property set to hidden
or opacity
property to 0
are considered visible in jQuery as they still consume space in the DOM.
Alternatively, to check the specific CSS property of the element to check if it is hidden, we can use the following method.
<script>
if ( $(h1).css('display') == 'none' || $(h1).css('opacity') == '0'){
alert("The heading is hidden.");
//your function here
}
</script>
Quick Tips to Check Whether an Element Is Hidden
jQuery cannot take advantage of the native DOM querySelectorAll()
method. It also creates some performance issues as it forces the browser to re-render the page to determine visibility. So rather than using elements, you can use classes or CSS selectors and then use .find(":hidden")
.
<h1 style="display:none;">Your text here</h1>
<script>
$(document).ready(function(){
var hiddenHeadings = $("body").find(":hidden").is("h1");
if(hiddenHeadings.length > 0){
alert("There is atleast one heading hidden.");
}
});
</script>
Here the hiddenHeadings
can be directly accessed and can be operated upon.