How to Change the Text of a Label Using jQuery
- Change the Text of a Label in jQuery
-
Use the jQuery
val()
Method to Change the Label Text -
Use the jQuery
text()
Method to Change the Label Text -
Use the jQuery
html()
Method to Change the Label Text -
Use the jQuery
append()
Method to Change the Label Text
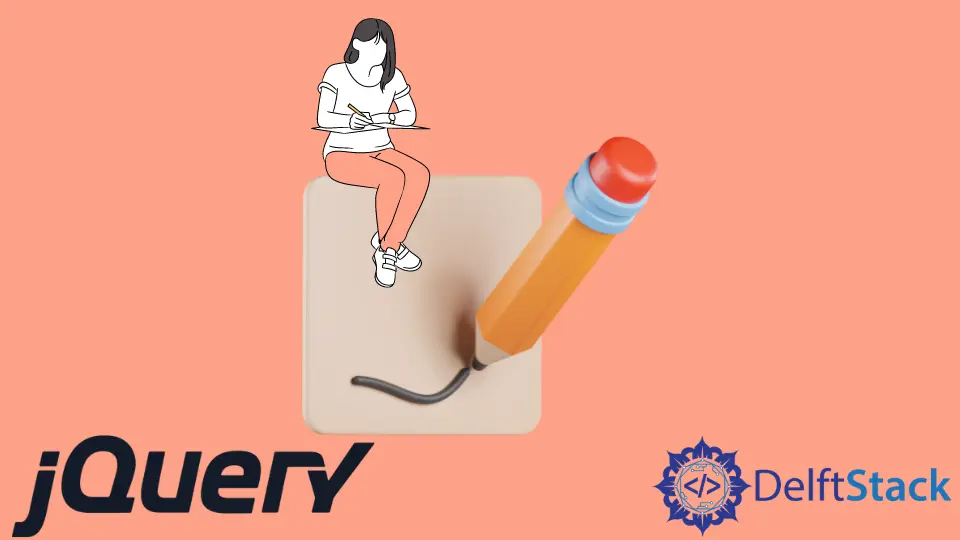
This article will cover numerous strategies and tactics for utilizing jQuery to modify the text content of the chosen HTML elements. Each approach will be discussed in depth, along with some actual functioning examples of each method.
Change the Text of a Label in jQuery
A lightweight JavaScript library called jQuery allows you to write less and accomplish more. The purpose of jQuery is to make managing JavaScript on your website considerably easier.
Many basic actions often accomplished by writing numerous lines of JavaScript code are wrapped in jQuery’s methods, so you need to write one line of code to use them. Learning about jQuery and utilizing it can improve your workflow.
We’ll explore using jQuery to alter the text of HTML components.
jQuery, a great tool, offers us several convenient ways to interact with HTML components. Today, we’ll talk about the methods .val()
, .text()
, .html()
, and .append()
and the way they could help us achieve the functionality we need.
Use the jQuery val()
Method to Change the Label Text
The val()
is a method used to perform operations on the values of elements in an HTML-based web page. This method can set or retrieve a particular element’s value.
The val()
method of jQuery can be used only on HTML elements with a value
attribute.
Syntax:
$(* selector*).val()
This will return the value of the selected element.
$(* selector*).val(* value*)
This will set the value of the selected element to the value we specified.
$(*selector*).val(function*(index, currentvalue)*)
This will set the value of the selected element by using a function. The index
parameter returns the element’s index, and the currentvalue
parameter returns the element’s value if specified.
What we need to do now is to see the working example of the above syntax and explanation.
Let’s discuss two cases: one where we want to override or set the attribute’s value entirely, and the second where we want to append the text to the previously specified value.
Set the Value of the Element
In this case, we will learn about setting the value of the selected HTML element using the .val()
function. Observe the code below to grasp the idea.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("input:text").val("val() method of jQuery.");
});
</script>
</head>
<body>
<input type="text" value="">
</body>
</html>
Output:
We selected the element input: text
, which means selecting the input element of type text. The value was null in the input tag, but we assigned the value to it using jQuery.
According to the above-discussed method, jQuery will select all the input tags of type text.
Let’s discuss another case where we have more than one input tag in our code, and we want to set the value of the specific one. Check the code below.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#Box2").val("val() method of jQuery.");
});
</script>
</head>
<body>
<input id="Box1" type="text" value="">
<input id="Box2" type="text" value="Hello">
<input id="Box3" type="text" value="">
</body>
</html>
Output:
You can see in the output that only the second input box contains a value, as we select only this one. If there are several input tags of the same type, we can give each one an ID to identify them.
Another essential point is that the second input tag already had some value, but it was overridden by the val()
method.
Append the Value to the Element
In this case, we will focus on appending the specified value to the previous value of the HTML element. Check the code below.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("input:text").val(function(i, value){
return value + " World";
});
});
</script>
</head>
<body>
<input type="text" value="Hello">
</body>
</html>
Output:
Let’s discuss what we did in the code.
We used the syntax of the val()
method, which takes a function. The parameter value
will give us the specified value of the element, Hello,
and then the string World
will append to it.
Use the jQuery text()
Method to Change the Label Text
The text()
function returns the text content of the selected elements. It works the same way innerText does in standard JavaScript to set or retrieve the selected element’s content.
When you want to display the value as plain text, use the text()
method.
Syntax:
$(* selector*).text()
This will return the text of the selected element.
$(* selector*).text(* content*)
This will set the selected element’s text to the specified content value.
$(*selector*).text(function*(index, currenttext)*)
This will set the text of the selected element by using a function. The index
parameter returns the element’s index, and the currenttext
parameter returns the element’s text content if any.
Let’s look at how the text()
method works to set or alter the content of HTML elements.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("p").text("I am text() method.");
});
</script>
</head>
<body>
<p></p>
</body>
</html>
Output:
The text()
method sets the <p>
tag’s content as shown in the output. It is same as if we write <p> I am text() method </p>
.
The text()
method using the function syntax can append the text to the content of the chosen element. The value is passed to the text()
method as a string and uses that string as the element’s text content.
If we provide <h1>Hello World</h1>
as a parameter in the text()
function, it will assign the entire string as it is, and the result will be the same as the passed value.
Observe the code below to understand it clearly.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("p").text("<h1>I am text() method.</h1>");
});
</script>
</head>
<body>
<p></p>
</body>
</html>
Output:
Use the jQuery html()
Method to Change the Label Text
The innerHTML or content of the selected element is set or returned by jQuery’s html()
method. It can contain html elements in its input string.
Syntax:
$(* selector*).html()
It will return the content of the selected element.
$(* selector*).html(* content*)
It will set the content of the selected element.
$(*selector*).html(function*(index,currentcontent)*)
It will set the content of the selected element using a function. The parameter currentcontent
retrieves the chosen element’s current HTML content, whereas the index
returns the element’s index position inside the set.
Let’s see the working examples of the html()
function.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("p").html("<h1>I am html() method.</h1>");
});
</script>
</head>
<body>
<p>I am paragraph</p>
</body>
</html>
Output:
It is necessary to take note of a few key details in the code and the results from this example. First, the <p>
tag already contains some HTML content, but the html()
method completely replaces it.
Second, this function displayed the content in the <h1>
tag after receiving the string with the HTML tags as an argument, indicating that this method rendered the HTML tags and text.
Use the jQuery append()
Method to Change the Label Text
The append()
method appends the provided content to the end of the components that have been selected. We can use this function to change the content of the HTML elements.
Let’s discuss the two cases to understand its working.
Append the Text
In this case, we’ll examine the procedure for adding new content to the HTML element’s existing content. Look at the code below.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("p").append(" <b>I am Appended</b>.");
});
</script>
</head>
<body>
<p>I am a paragraph.</p>
</body>
</html>
Output:
Observe the output. The append()
method appends the string we provide to the <p>
tag’s current content.
Additionally, the append()
method reads the HTML tags along with the text as we define our text in the <b>
tag, which is used to bold the text, and the appended text is in bold format.
Replace the Text
We’ve seen appending the text, but what if we want to replace the content of the HTML element?
Let’s see what we can come up with. Check the code below.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("p").empty();
$("p").append("I am new paragraph.");
});
</script>
</head>
<body>
<p>I am a paragraph.</p>
</body>
</html>
Output:
The code is simple to grasp. We called the .empty()
method on our element, which removes its content, then called the append()
method.