How to Zip Two Arrays in JavaScript
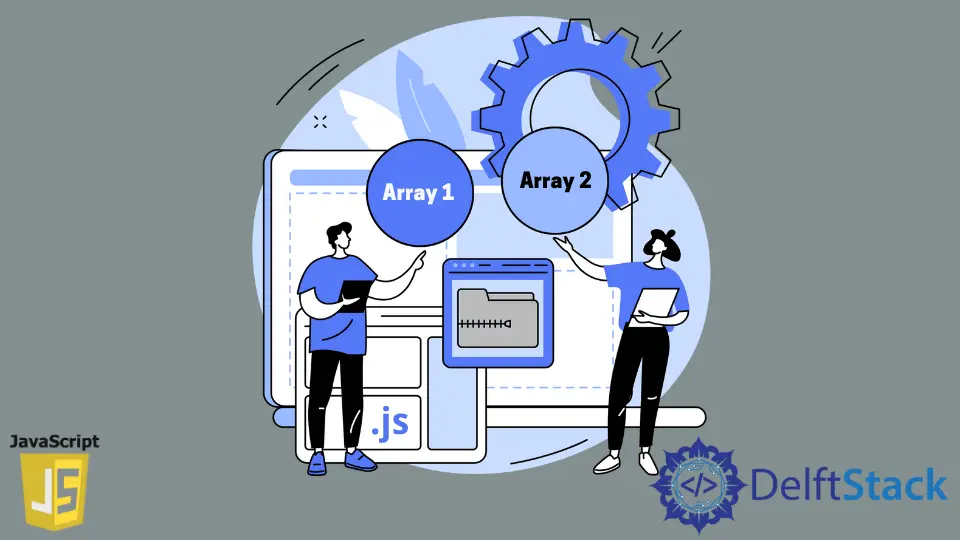
When working with arrays in JavaScript, you may often find yourself needing to combine or “zip” two arrays together. This process allows you to create a new array that contains elements from both arrays, paired based on their respective indices. The most commonly used and straightforward method for zipping two arrays in JavaScript is the map()
method. Other methods, such as Array.from()
and Array.prototype.fill()
, are also popular choices.
In this article, we will explore these methods in detail, providing clear code examples and explanations to help you understand how to zip arrays effectively.
Using the map()
Method
The map()
method is a versatile function that creates a new array by applying a callback function to each element of the original array. To zip two arrays using map()
, you can iterate over one of the arrays while accessing elements from both arrays based on their indices. This method is not only simple but also efficient, making it a preferred choice for many developers.
Here’s an example of how to zip two arrays using the map()
method:
const array1 = [1, 2, 3];
const array2 = ['a', 'b', 'c'];
const zippedArray = array1.map((item, index) => [item, array2[index]]);
console.log(zippedArray);
Output:
[[1, 'a'], [2, 'b'], [3, 'c']]
In this code, we have two arrays: array1
and array2
. The map()
method is called on array1
, and for each element, it creates a new array containing the current element from array1
and the corresponding element from array2
. The result is a new array, zippedArray
, that contains pairs of elements from both arrays. This method is straightforward and works well when both arrays are of the same length.
Using Array.from()
Another effective way to zip two arrays is by using the Array.from()
method. This method creates a new, shallow-copied array instance from an array-like or iterable object. By combining it with a mapping function, you can achieve the desired zipping effect.
Here’s how you can use Array.from()
to zip two arrays:
const array1 = [1, 2, 3];
const array2 = ['a', 'b', 'c'];
const zippedArray = Array.from({ length: array1.length }, (_, index) => [array1[index], array2[index]]);
console.log(zippedArray);
Output:
[[1, 'a'], [2, 'b'], [3, 'c']]
In this example, we create a new array of the same length as array1
using Array.from()
. The second argument is a mapping function that takes the current index and returns a new array containing the elements from both array1
and array2
. This method is particularly useful when you want to create an array based on the length of one of the original arrays, ensuring that the zipping process is efficient and concise.
Using Array.prototype.fill()
The Array.prototype.fill()
method can also be utilized to zip two arrays, although it’s a bit less common compared to the previous methods. This method fills all the elements of an array from a start index to an end index with a static value. To zip two arrays, you can first create an empty array and then fill it using a mapping function.
Here’s an example of zipping arrays using Array.prototype.fill()
:
const array1 = [1, 2, 3];
const array2 = ['a', 'b', 'c'];
const zippedArray = new Array(array1.length).fill().map((_, index) => [array1[index], array2[index]]);
console.log(zippedArray);
Output:
[[1, 'a'], [2, 'b'], [3, 'c']]
In this code snippet, we first create a new empty array with the same length as array1
. We then use fill()
to initialize the array, allowing us to use map()
to iterate through the indices. This approach effectively zips the two arrays together, producing the desired result. While this method might seem a bit roundabout, it demonstrates the flexibility of JavaScript array methods.
Conclusion
Zipping two arrays in JavaScript is a common task that can be accomplished using several methods, including map()
, Array.from()
, and Array.prototype.fill()
. Each method has its advantages, and the choice of which to use can depend on the specific requirements of your project. The map()
method is often the most straightforward and efficient for this purpose, while Array.from()
and fill()
offer additional flexibility. By mastering these techniques, you can enhance your JavaScript skills and improve your ability to manipulate arrays effectively.
FAQ
-
What does it mean to zip two arrays in JavaScript?
Zipping two arrays means combining them into a new array where elements from both arrays are paired together based on their indices. -
Can I zip arrays of different lengths?
Yes, but you will need to handle the case where one array is shorter than the other. You can use methods that check the lengths and adjust accordingly.
-
Is the map() method the best way to zip arrays?
Themap()
method is one of the most common and straightforward ways to zip arrays, but other methods likeArray.from()
andfill()
can also be effective depending on your needs. -
What happens if I use map() on arrays of different lengths?
If you usemap()
on arrays of different lengths, it will only iterate up to the length of the shorter array, potentially leaving some elements from the longer array unpaired. -
Are there performance differences between these methods?
Generally, performance differences are minimal for small arrays, but for larger arrays, the efficiency of each method can vary based on how they are implemented in JavaScript engines.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript