How to Call Window Onload Function Call in JavaScript
-
window.onload
Functionality Usage With Example in HTML -
Examine the Given
HTML
Code in Few Steps - Alternative Way to See the Same Results
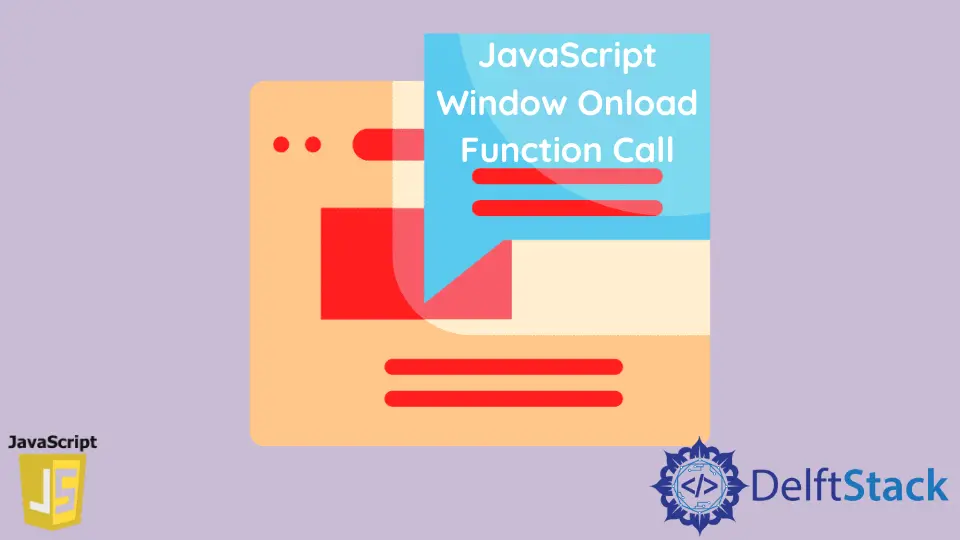
This article explains the purpose of the window.onload method in JavaScript by an example. The window.onload function executes to perform a task once the page on the browser finishes loading.
window.onload
Functionality Usage With Example in HTML
The window.onload
method is triggered when the page is entirely loaded on the browser (as well as all images, style, scripts, and CDN). Here you can see an example of HTML
page source code that uses the window.onload method for auto-execution to display an alert box.
<html>
<head>
<title>(Window.onload function in HTML)</title>
<script type="text/javascript">
function code(){
alert(" Alert inside code function Window.onload");
}
window.onload=code();
</script>
</head>
<body>
<h1> Graphic Designer Firm </h1>
<p1> A craft of creating visual content to communicate messages.</p1>
</br> <img src = "Graphic-Design.png" height="500px" width="500px" >
</br> <hr width="800">
</body>
</html>
In the above HTML
page source, you can see the <script type="text/javascript">
tag that uses JavaScript
in doctype html. In the next step function code()
is declared inside of the <script>
tag.
The function code()
is called window.onload
. It displays a default alert dialogue when a web page loads completely with images and text content.
Examine the Given HTML
Code in Few Steps
Follow these four easy steps and get a clear understanding of the window.onload
method.
-
Create a text document. You can utilize notepad or any other text editing tool.
-
Paste the given code in the created text file.
-
Save that text file with the extension of
.html
and open it with any default browser. -
You can see an alert box that pops up right after the complete page load.
Alternative Way to See the Same Results
You can achieve the alert message results by using an alternative onload
event via a body tag. Here is an example of a body tag that uses auto-execution to call a function code containing an alert box:
<script type="text/javascript">
function code(){
alert(" Alert inside code function");
}
</script>
<body onLoad="code()";>
In both examples, onLoad
of body tag and window.onload
have the same functionality to execute the statements after completing page load.