Variable Scope in JavaScript
- Block Scope of Variables in JavaScript
- Local Scope of Variables in JavaScript
- Global Scope of Variables in JavaScript
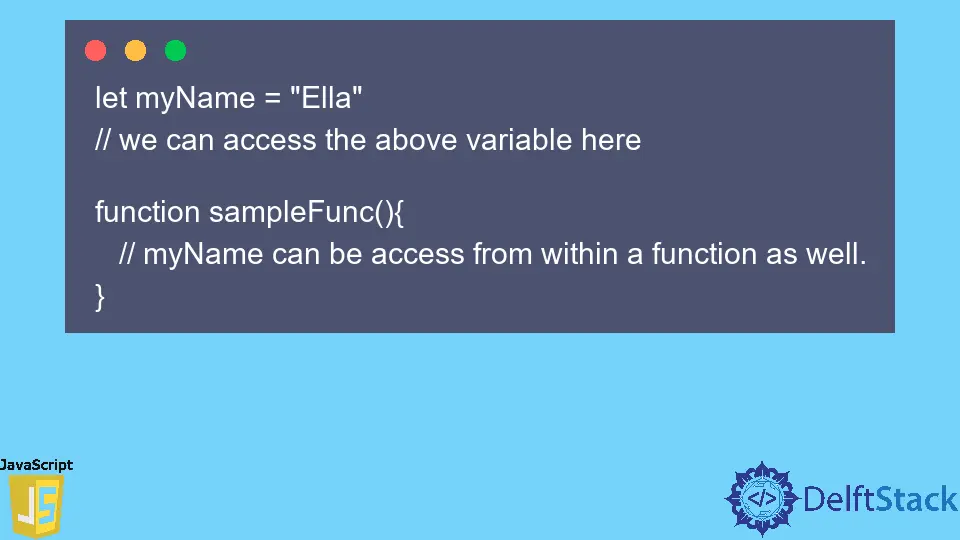
The scope of a variable is the accessibility or visibility of the variable in the program.
We have three types of variable scope in JavaScript. These are the Block, Local, and Global scope.
Let us discuss these in this article.
Block Scope of Variables in JavaScript
Before the introduction of ES6 in 2015, Javascript only had the Global and Function scope. After the introduction of ES6, two new keywords were introduced, let
and const
. These keywords made the block scope a possibility.
Variables declared within the curly braces {}
fall under the block scope as we can not access them from outside the block.
See the code below.
{ let a = 17; }
// a cannot be used here from outside the block
Variables declared using the keyword var
cannot have block scope.
For example,
{ var a = 17 }
// a can be used here from outside the block
Local Scope of Variables in JavaScript
A variable in JavaScript has the local scope when it is declared within a function. These are not accessible from outside the function.
For example,
function sampleFunction() {
let myName = 'Hemank';
}
// myName cannot be accessed here
Variables declared with the var
, let
, and const
keywords all have function scope.
Global Scope of Variables in JavaScript
All the variables that are defined outside a function are global. These are accessible anywhere in the program and not limited to any function.
For example,
let myName = 'Ella'
// we can access the above variable here
function sampleFunc() {
// myName can be access from within a function as well.
}
In the above code, the declaration of the variable myName
was outside the function. Thus, it has become global and can be used from within the function or outside the function.
Related Article - JavaScript Variable
- How to Access the Session Variable in JavaScript
- How to Check Undefined and Null Variable in JavaScript
- How to Mask Variable Value in JavaScript
- Why Global Variables Give Undefined Values in JavaScript
- How to Declare Multiple Variables in a Single Line in JavaScript
- How to Declare Multiple Variables in JavaScript