How to Update Array Values in JavaScript
Kushank Singh
Feb 02, 2024
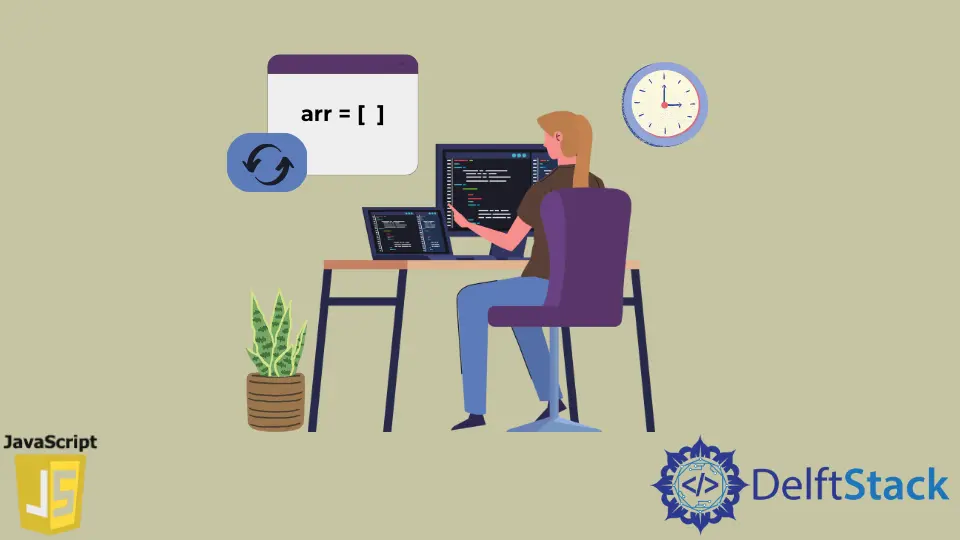
Arrays are list-like objects because they are single objects that contain similar values under a common name. Arrays are mutable objects. Hence we can update the array values.
This tutorial will demonstrate how to update array values in JavaScript.
We can access elements using their indexes. So, we can update elements using their indexes.
For example,
var array1 = ['fruits', 'vegetables', 'apple'];
array1[1] = 'mango';
console.log(array1);
Output:
['fruits', 'mango', 'apple']
A function can also be created that takes the array, index, and the new value as parameters to update the following array.
For example,
var array1 = ['fruits', 'vegetables', 'Refrigerator'];
function update(array, index, newValue) {
array[index] = newValue;
}
let newVal = 'apple';
update(array1, 2, newVal);
console.log(array1);
Output:
['fruits', 'vegetables', 'apple']
We can also update objects. They can be an alternative to arrays. We have to access the values individually using their keys and update them. We can access these values either using the .
operator or the []
brackets.
For example,
var ob1 = {'a': 1, 'b': 2, 'c': 3};
ob1.a = 12
ob1['b'] = 15;
console.log(ob1)
Output:
{a: 12, b: 15, c: 3}
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript