Unicode in JavaScript
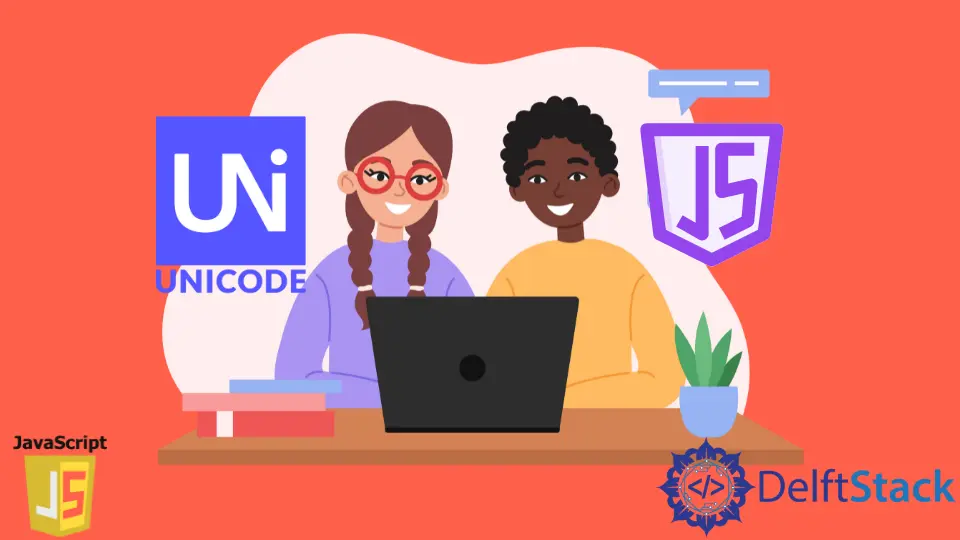
This article helps you to understand the insertion of Unicode characters into JavaScript.
Unicode in JavaScript
According to the ES2015 specification, source code text is represented using Unicode (version 5.1 and above). The source text consists of code points ranging from U+0000
to U+10FFFF
.
How source code is saved or exchanged is unimportant to the ECMAScript standard; however, it is often encoded as UTF-8.
There are two ways to insert the Unicode characters in JavaScript; using Unicode escape sequence and String.fromCodePoint
.
Unicode Escape Sequence
String escape sequences convey code units based on code point numbers.
3 escape types in JavaScript:
- Hexadecimal escape
- Unicode escape
- Code point escape
To insert Omega
, you can escape a Unicode code point using the Unicode escape sequence \u{XXXXXX}
(where X
denotes 1-6 hexadecimal digits in the range of U+0000
to U+10FFFF
, which covers the full Unicode).
For example, to insert Omega
, i.e.,(U+03A9
) into a JavaScript string, you can do it in the following way.
const ome = 'Omega: \u{03A9}';
console.log(ome);
Output:
"Omega: Ω"
Unicode has grown to contain additional characters not included in the BMP (Basic Multilingual Plane
). These characters are represented by surrogate pairs, code points that cannot be added directly in previous versions of JavaScript.
Two adjoined Unicode escape sequences would be required to represent such characters appropriately.
Use String.fromCodePoint()
in JavaScript
Using the String.fromCodePoint()
function, you may add a Unicode code point to a JavaScript string. It takes a series of code points (decimal, hexadecimal, octal, etc.) as an input.
For example, use the decimal code point to display Omega
.
const omee = `Omega: ${String.fromCodePoint(937)}`;
console.log(omee);
Output:
"Omega: Ω"
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn