How to Sum Array of Objects in JavaScript
-
Use the
reduce
Function to Sum the Values of an Array of Objects in JavaScript -
Use the
map()
andreduce()
Functions to Sum the Values of an Array of Objects in JavaScript
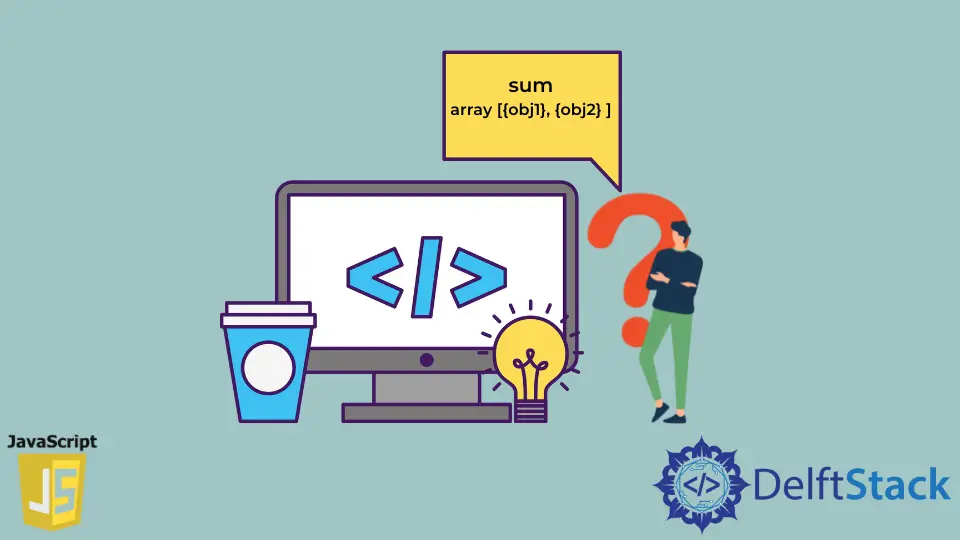
An array can store similar types of elements, and this includes objects also.
In this article, we will learn better and efficient ways to sum the values of an array of objects in JavaScript.
In our examples, we will sum the Amount
for all objects in the following array.
fruits = [
{description: 'orange', Amount: 50},
{description: 'orange', Amount: 50},
{description: 'apple', Amount: 75},
{description: 'kiwi', Amount: 35},
{description: 'watermelon', Amount: 25},
];
Use the reduce
Function to Sum the Values of an Array of Objects in JavaScript
We can create a function called sum()
and use the reduce()
method to reduce the array to a single value, which is the sum of all the values in the array.
This method helps in filtering and mapping the value simultaneously in a single pass.
We implement this in the following code.
class fruitCollection extends Array {
sum(key) {
return this.reduce((a, b) => a + (b[key] || 0), 0);
}
}
const fruit = new fruitCollection(
...[{description: 'orange', Amount: 50},
{description: 'orange', Amount: 50},
{description: 'apple', Amount: 75},
{description: 'kiwi', Amount: 35},
{description: 'watermelon', Amount: 25},
]);
console.log(fruit.sum('Amount'));
Output:
235
Use the map()
and reduce()
Functions to Sum the Values of an Array of Objects in JavaScript
The map()
function builds a new array by changing the value of every element in an array respectively. Then the filter function is used to remove the values that do not fall in that category. Finally, the reduce()
function takes all the values and returns a single value, which is the required sum.
For example,
fruits = [
{description: 'orange', Amount: 50},
{description: 'orange', Amount: 50},
{description: 'apple', Amount: 75},
{description: 'kiwi', Amount: 35},
{description: 'watermelon', Amount: 25},
];
const sumall =
fruits.map(item => item.amount).reduce((prev, curr) => prev + curr, 0);
console.log(sumall);
Output:
235