How to Splice String in JavaScript
- Understanding String Manipulation in JavaScript
- Using Substring and Concatenation
- Using Array Methods for Splicing
- Using Regular Expressions for Splicing
- Conclusion
- FAQ
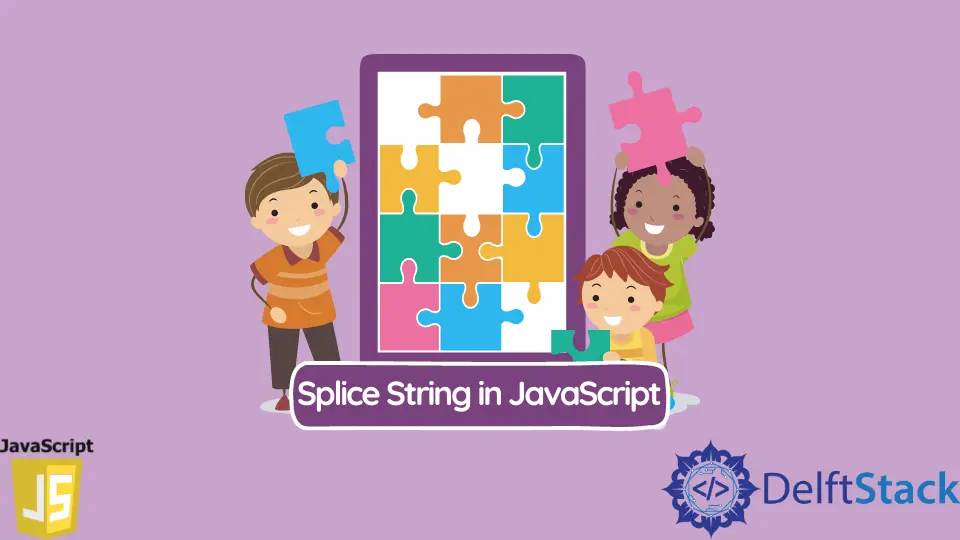
In this article, we will learn how to add or remove certain indexed characters using the splice method in JavaScript. Splicing strings can be a handy technique when you want to manipulate individual characters or sections within a string. While JavaScript does not have a built-in splice
method for strings, we can achieve similar functionality using a combination of string methods. By understanding how to effectively splice strings, you can enhance your programming skills and create more dynamic applications. Let’s dive into the various methods you can use to splice strings in JavaScript!
Understanding String Manipulation in JavaScript
Before we explore splicing techniques, it’s essential to understand how strings work in JavaScript. Strings are immutable, meaning that once created, they cannot be changed. When you attempt to modify a string, a new string is created instead. This characteristic makes it crucial to know how to manipulate strings effectively, especially when you want to add or remove characters.
Using Substring and Concatenation
One of the most straightforward methods to splice a string is by using substring()
combined with concatenation. This approach allows you to extract parts of the string and combine them back together, effectively splicing out unwanted characters or inserting new ones.
Here’s how it works:
function spliceString(original, index, removeCount, newSubstr) {
return original.substring(0, index) + newSubstr + original.substring(index + removeCount);
}
let originalString = "Hello World!";
let splicedString = spliceString(originalString, 6, 5, "JavaScript");
console.log(splicedString);
Output:
Hello JavaScript!
In this example, we define a function spliceString
that takes four parameters: the original string, the index at which to start splicing, the number of characters to remove, and the new substring to insert. The substring()
method extracts parts of the original string before and after the specified index. These parts are then concatenated with the new substring, yielding the desired result.
This method is efficient for simple splicing tasks, especially when you know the exact index and number of characters to manipulate. However, it can become cumbersome with more complex string manipulations.
Using Array Methods for Splicing
Another effective way to splice strings is by converting the string into an array of characters. JavaScript arrays have a splice
method that allows you to modify the array directly. After splicing the array, you can join it back into a string. This method can be particularly useful for more complex string manipulations.
Here’s an example:
function spliceStringWithArray(original, index, removeCount, newSubstr) {
let charArray = original.split('');
charArray.splice(index, removeCount, ...newSubstr.split(''));
return charArray.join('');
}
let originalString = "Hello World!";
let splicedString = spliceStringWithArray(originalString, 6, 5, "JavaScript");
console.log(splicedString);
Output:
Hello JavaScript!
In this code, we first split the original string into an array of characters. We then use the splice()
method to remove a specified number of characters starting from the given index, replacing them with the characters from the new substring. Finally, we join the modified array back into a string. This method is quite powerful, allowing for more flexibility in string manipulation.
Using Regular Expressions for Splicing
Regular expressions (regex) offer a more advanced method for splicing strings in JavaScript. They allow you to identify patterns within a string and manipulate them accordingly. This method is particularly useful when you need to splice based on specific character patterns rather than fixed indices.
Here’s how you can implement it:
function spliceStringWithRegex(original, regex, newSubstr) {
return original.replace(regex, newSubstr);
}
let originalString = "Hello World!";
let splicedString = spliceStringWithRegex(originalString, /World/, "JavaScript");
console.log(splicedString);
Output:
Hello JavaScript!
In this example, we define a function spliceStringWithRegex
that takes the original string, a regex pattern, and the new substring to insert. The replace()
method searches for the pattern defined by the regex and replaces it with the new substring. This method is particularly powerful for more complex string manipulations, allowing you to target specific patterns rather than relying solely on indices.
Conclusion
Splicing strings in JavaScript is a valuable skill that can enhance your programming capabilities. By utilizing methods like substring concatenation, array manipulation, and regular expressions, you can achieve a wide range of string modifications. Each method has its strengths, and the choice of which to use often depends on the specific requirements of your task. By mastering these techniques, you can create more dynamic and interactive web applications.
FAQ
-
What is string splicing in JavaScript?
String splicing refers to the process of adding or removing characters from a string at specific indices. -
Can I use the splice method directly on strings in JavaScript?
No, strings in JavaScript are immutable, and the splice method is not available for strings. You can use alternative methods like substring or array manipulation. -
What are the best methods for splicing strings?
The best methods include using substring with concatenation, converting strings to arrays and using the splice method, and utilizing regular expressions for pattern-based replacements. -
Is it possible to splice multiple characters at once?
Yes, you can splice multiple characters by specifying the number of characters to remove and providing a new substring to insert. -
How do I handle edge cases when splicing strings?
Always check the indices you are working with to avoid errors. Ensure that the index is within the bounds of the string length and that the remove count does not exceed the remaining characters.