How to Sort Data in Descending Using JavaScript
-
Use the
sort()
Method in JavaScript -
Use the
reverse()
Method in JavaScript -
Use the
sort()
and thereverse()
Method to Sort Data in Descending - Sort Data Descending Using Custom Function
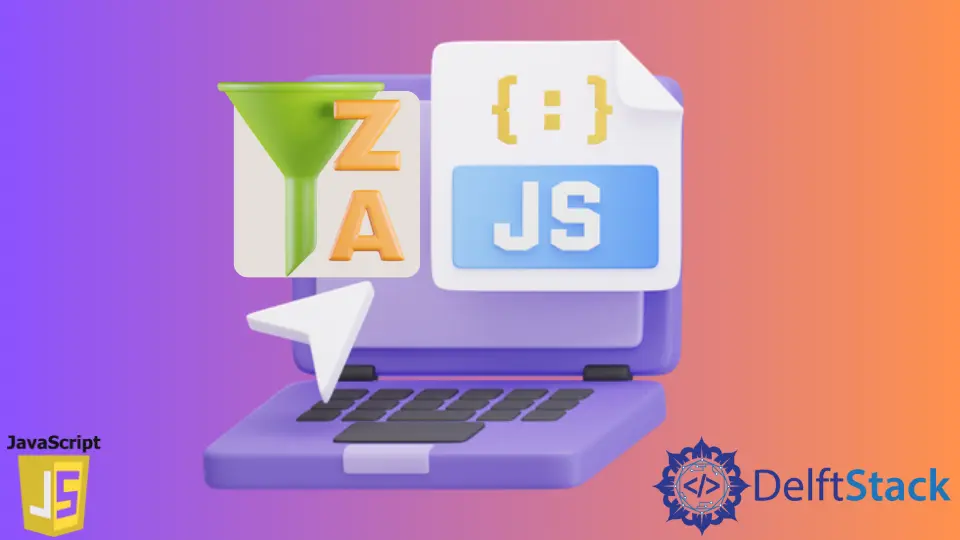
In JavaScript, we will sort and set array data in descending order with the help of default array methods like sort()
and reverse()
. We can create a function to achieve the same results, and we will implement both ways to achieve array data in descending order.
Use the sort()
Method in JavaScript
In JavaScript, sort()
is a pre-defined method. It will sort out the array elements.
Syntax:
let array = ['c', 'b', 'd', 'a', 'e']
array.sort()
Use the reverse()
Method in JavaScript
In JavaScript, reverse()
is a pre-defined method. It will reverse out the array elements.
Syntax:
let array = ['a', 'b', 'c', 'd', 'e']
array.reverse()
Use the sort()
and the reverse()
Method to Sort Data in Descending
To sort data in descending order, we need an array of data to implement both methods on a single array. We will initialize an array containing un-sorted elements and test the sort and reverse methods.
Example:
<script>
let array = ["c","b","d","a","e"]
console.log("original array array :"+array)
let result = array.sort()
result = array.reverse()
console.log("sorted descending array :"+result)
</script>
Output:
"original array array :c,b,d,a,e"
"sorted descending array :e,d,c,b,a"
In the above code, we initialized an array containing un-sorted elements of characters. We used the sort()
method on that array to sort out the elements first.
Then we used the reverse()
method on the resultant sorted array to change the order in descending. Lastly, we used the console.log()
to display the results.
Sort Data Descending Using Custom Function
Example:
let array = ['c', 'b', 'd', 'a', 'e']
console.log(array.sort((a, b) => {
const lastCodeIn = b.toLowerCase().charCodeAt();
const lastCode = b.charCodeAt();
const firstCodeIn = a.toLowerCase().charCodeAt();
const firstCode = a.charCodeAt();
if (lastCodeIn - firstCodeIn === 0) {
return lastCode - firstCode;
}
return lastCodeIn - firstCodeIn;
}));
Output:
"original array array :c,b,d,a,e"
"sorted descending array :e,d,c,b,a"
We initialized an array containing un-sorted elements of characters in the above example. We used the custom method on that array to sort out the elements in descending.
We replaced elements with the help of character codes. Then display the results using the console.log()
method.