How to Sort by Date in JavaScript
- Understanding JavaScript Date Objects
- Sorting Dates in Ascending Order
- Sorting Dates in Descending Order
- Handling Date Strings
- Conclusion
- FAQ
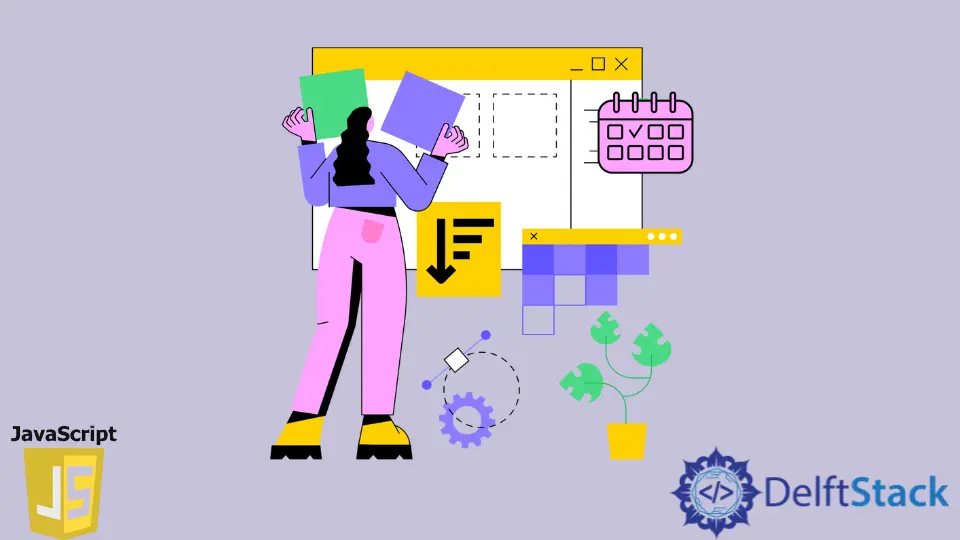
In this article, we’ll learn how to sort by date in ascending or descending order in JavaScript. Sorting dates can be a crucial task in web development, especially when dealing with data-driven applications. Whether you’re managing user events, displaying logs, or organizing any time-sensitive data, knowing how to sort dates effectively is essential. JavaScript provides various methods to handle date sorting, making it easier for developers to present data in a meaningful way. Let’s dive into the different approaches to sorting dates in JavaScript, complete with code examples and detailed explanations.
Understanding JavaScript Date Objects
Before we jump into sorting, it’s important to grasp how JavaScript handles dates. JavaScript has a built-in Date
object that represents dates and times. You can create a date object using the new Date()
constructor. This object allows you to perform operations like getting the year, month, day, and so forth.
To sort dates, you typically need to convert them into a format that can be compared. The getTime()
method of the Date
object is particularly useful here, as it returns the number of milliseconds since January 1, 1970, which can be easily compared.
Here’s a quick example of creating date objects:
javascriptCopyconst date1 = new Date('2023-01-01');
const date2 = new Date('2022-12-31');
Output:
textCopyDate objects created successfully
In this example, we created two date objects representing January 1, 2023, and December 31, 2022.
Sorting Dates in Ascending Order
To sort an array of dates in ascending order, you can use the sort()
method combined with a comparison function. The comparison function should return a negative number if the first date is earlier than the second, zero if they are the same, and a positive number otherwise.
Here’s how you can do it:
javascriptCopyconst dates = [
new Date('2023-01-01'),
new Date('2022-12-31'),
new Date('2023-03-15'),
];
dates.sort((a, b) => a.getTime() - b.getTime());
console.log(dates);
Output:
textCopy[
2022-12-31T00:00:00.000Z,
2023-01-01T00:00:00.000Z,
2023-03-15T00:00:00.000Z
]
In this code snippet, we created an array of date objects. The sort()
method is called on this array with a comparison function that uses getTime()
to compare the dates. As a result, the dates are sorted in ascending order.
Sorting Dates in Descending Order
Sorting dates in descending order is similar to sorting in ascending order, but the comparison function needs to be reversed. Instead of subtracting a.getTime()
from b.getTime()
, you will subtract b.getTime()
from a.getTime()
.
Here’s how you can achieve that:
javascriptCopyconst dates = [
new Date('2023-01-01'),
new Date('2022-12-31'),
new Date('2023-03-15'),
];
dates.sort((a, b) => b.getTime() - a.getTime());
console.log(dates);
Output:
textCopy[
2023-03-15T00:00:00.000Z,
2023-01-01T00:00:00.000Z,
2022-12-31T00:00:00.000Z
]
In this example, the same array of dates is sorted, but this time in descending order. By reversing the subtraction in the comparison function, we get the dates sorted from the most recent to the oldest.
Handling Date Strings
When sorting dates, you may encounter date strings instead of Date
objects. You can still sort these strings, but you need to convert them into Date
objects first. Here’s how to do that:
javascriptCopyconst dateStrings = [
'2023-01-01',
'2022-12-31',
'2023-03-15',
];
dateStrings.sort((a, b) => new Date(a) - new Date(b));
console.log(dateStrings);
Output:
textCopy[
'2022-12-31',
'2023-01-01',
'2023-03-15'
]
In this code, we have an array of date strings. The sort()
method takes a comparison function that converts each string to a Date
object for comparison. This allows us to sort the strings correctly based on their date values.
Conclusion
Sorting by date in JavaScript is a straightforward process once you understand how to utilize the Date
object and the sort()
method. Whether you need to sort dates in ascending or descending order, JavaScript provides the tools necessary to achieve your goals. By mastering these techniques, you will be better equipped to handle date-related data in your web applications.
In summary, remember to always convert date strings to Date
objects when sorting, and use the getTime()
method for accurate comparisons. With these tips, you can efficiently manage and display date information in your projects.
FAQ
-
How do I sort an array of date strings in JavaScript?
You can use thesort()
method along with a comparison function that converts the date strings intoDate
objects. -
Can I sort dates in different formats?
Yes, as long as the date strings are in a format that JavaScript can parse, you can sort them after converting toDate
objects.
-
What happens if the dates are the same?
If two dates are the same, the comparison function will return zero, and their order will remain unchanged in the sorted array. -
Is it possible to sort dates without using
getTime()
?
While it is possible to sort withoutgetTime()
, using it is the most reliable way to ensure accurate comparisons. -
Can I sort dates in an object?
Yes, you can extract the date values from an object and then sort them using the same methods discussed in this article.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn