How to Set Background Image of a Div via Function in JavaScript
-
Use the
HTML DOM style
Property to Set Background ofdiv
Element in JavaScript -
Use the
createElement
to Initializeimg
Element in thediv
Element to Set Background in JavaScript
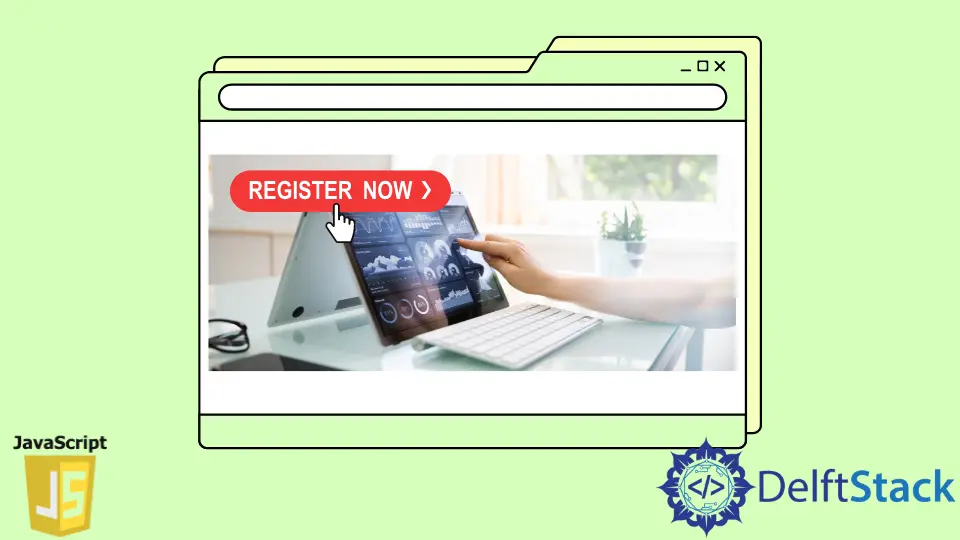
JavaScript has multiple ways of using image sources and embedding them in the HTML body.
The onclick
or onchange
methods are functions used to change a div
element. We can also create an img
element within the div
element directed at the scripted lines.
We will perform two ways of setting the background of a div
element. We will use functions in both cases, and the difference will be by creating an img
element and by setting the background in a raw method.
Use the HTML DOM style
Property to Set Background of div
Element in JavaScript
We will initially set a div
element in the HTML structure followed by an id
named div
.
The following control goes to the script tag where our button onclick
method will trigger the function display
.
Here the style
property will manipulate the DOM to set the background image after the click operation.
Code Snippet:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Test</title>
</head>
<body>
<div id="Div" style="height:200px;width:200px;">
<button onclick="display()">Click</button>
</div>
<script>
function display(){
document.getElementById("Div").style.backgroundImage = 'url("https://pngimg.com/uploads/acorn/acorn_PNG37020.png")';
}
</script>
</body>
</html>
Output:
Use the createElement
to Initialize img
Element in the div
Element to Set Background in JavaScript
In this case, we will create a div
element followed by an id
in the HTML structure.
The rest of the task generates an object that will hold the image URL
in the script tag.
Later we will grab the div id
and create the img
element. The image object will then append the stored URL
and preview on the HTML body.
Code Snippet:
<!DOCTYPE html>
<html>
<body>
<div id="imgDiv" style="height:200px;width:200px;"></div>
<script>
var pic = "https://pngimg.com/uploads/acorn/acorn_PNG37020.png";
function display(pic) {
let div = document.getElementById("imgDiv");
let img = document.createElement("img");
img.src = pic
div.append(img);
}
display(pic);
</script>
</body>
</html>
Output: