selectedIndex Property in JavaScript
- What is the selectedIndex Property?
- Accessing selectedIndex in JavaScript
- Changing the selectedIndex Property
- Using selectedIndex in Event Listeners
- Conclusion
- FAQ
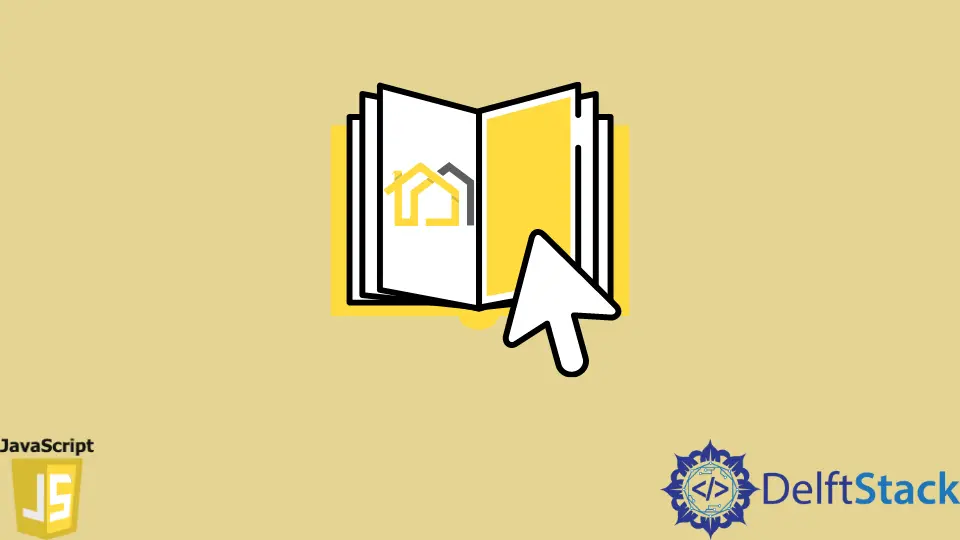
In the world of web development, JavaScript plays a crucial role in enhancing user interactions. One of the essential features of JavaScript is the ability to manipulate HTML elements dynamically. Among these elements, the <select>
dropdown is widely used for forms and user selections.
In this article, we will dive into the selectedIndex property, which allows developers to easily retrieve the index of the currently selected option in a dropdown menu. Understanding how to use this property effectively can enhance your web applications and improve user experience. So, let’s explore how to harness the power of the selectedIndex property in JavaScript.
What is the selectedIndex Property?
The selectedIndex property is part of the HTMLSelectElement interface in JavaScript. It returns the index of the currently selected option in a drop-down list. If no option is selected, it returns -1. This feature is particularly useful when you need to determine which option the user has chosen, allowing you to tailor the application’s response accordingly.
For example, if you have a dropdown menu with options for different fruits, the selectedIndex property can help you identify which fruit the user has selected. This can be beneficial for processing forms, updating other elements on the page, or even triggering specific functions based on user input.
Accessing selectedIndex in JavaScript
To access the selectedIndex property, you first need to get a reference to the <select>
element in your HTML. This can be done using document.getElementById or other DOM selection methods. Once you have the reference, you can simply call the selectedIndex property to get the index of the selected option.
Here’s a simple example:
<select id="fruitSelect">
<option value="apple">Apple</option>
<option value="banana">Banana</option>
<option value="cherry">Cherry</option>
</select>
<button onclick="showSelectedIndex()">Show Selected Index</button>
<script>
function showSelectedIndex() {
var selectElement = document.getElementById("fruitSelect");
var index = selectElement.selectedIndex;
alert("Selected index: " + index);
}
</script>
Output:
Selected index: 1
In this example, when the user clicks the button, the selectedIndex property retrieves the index of the currently selected fruit. If the user has selected “Banana,” the alert will display “Selected index: 1,” since “Banana” is the second option in the list (indexing starts at 0).
Changing the selectedIndex Property
You can also modify the selectedIndex property to programmatically change which option is selected. This can be useful when you want to reset a form or change the selection based on user actions.
Here’s how you can change the selected option:
<select id="colorSelect">
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
<button onclick="changeSelection()">Select Green</button>
<script>
function changeSelection() {
var selectElement = document.getElementById("colorSelect");
selectElement.selectedIndex = 1; // Change to Green
}
</script>
Output:
The selected option is now Green.
In this example, clicking the button will change the selection to “Green” by setting the selectedIndex property to 1. This demonstrates how you can manipulate the dropdown dynamically, enhancing user experience and interactivity.
Using selectedIndex in Event Listeners
Another powerful way to utilize the selectedIndex property is by using it within event listeners. This allows you to respond to user selections in real-time. For instance, you can listen for changes in the dropdown and react accordingly.
Here’s an example:
<select id="animalSelect" onchange="displayAnimal()">
<option value="dog">Dog</option>
<option value="cat">Cat</option>
<option value="rabbit">Rabbit</option>
</select>
<p id="output"></p>
<script>
function displayAnimal() {
var selectElement = document.getElementById("animalSelect");
var index = selectElement.selectedIndex;
var selectedAnimal = selectElement.options[index].value;
document.getElementById("output").innerText = "You selected: " + selectedAnimal;
}
</script>
Output:
You selected: Cat
In this case, whenever the user selects a different animal from the dropdown, the displayAnimal function is triggered. It retrieves the selected index and uses it to fetch the corresponding value, updating the text on the page to reflect the user’s choice. This interactivity makes the application more engaging.
Conclusion
The selectedIndex property in JavaScript is a powerful tool for managing user selections in dropdown menus. By understanding how to access, modify, and utilize this property, developers can create dynamic and responsive web applications. Whether you’re building forms, interactive interfaces, or data-driven applications, mastering the selectedIndex property will undoubtedly enhance your development skills. So, next time you work with dropdowns, remember the power of selectedIndex and how it can improve your user experience.
FAQ
-
What does the selectedIndex property return if no option is selected?
It returns -1 if no option is selected. -
Can I set selectedIndex to a value greater than the number of options?
No, setting selectedIndex to a value greater than the number of options will not select any option.
-
How can I get the value of the selected option?
You can useselectElement.options[selectElement.selectedIndex].value
to get the value of the selected option. -
Is selectedIndex zero-based?
Yes, selectedIndex is zero-based, meaning the first option has an index of 0. -
Can I use selectedIndex with multiple select elements?
Yes, you can use selectedIndex with multiple select elements by referencing each select element individually.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn