Select Onchange in JavaScript
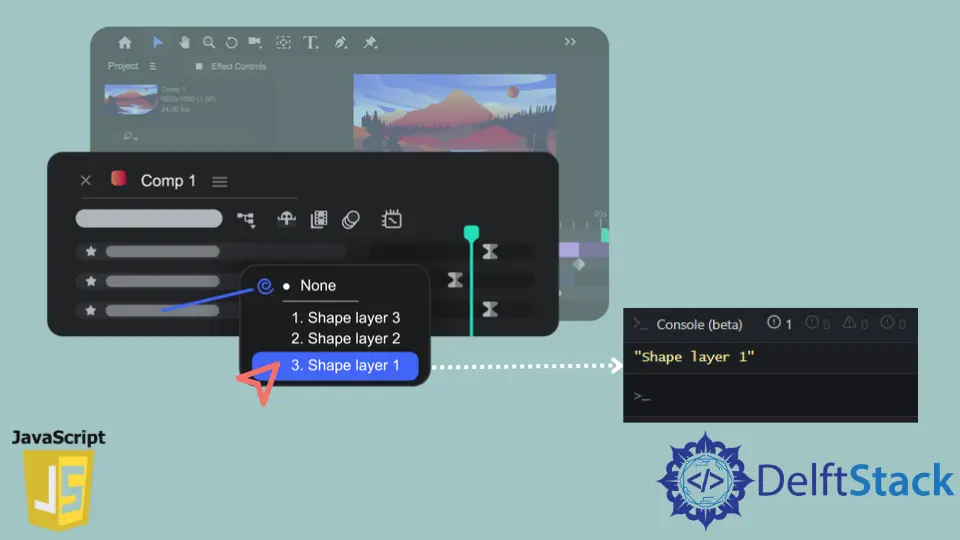
select
is an HTML input element that specifies the dropdown list of options from which the user can choose any option. This article will learn how to detect the onChange
event of this HTML select
in JavaScript.
Detect onChange
Event of the select
Element in JavaScript
The change
event is raised for input
, select
, and textarea
when the user commits a change to the element’s value. Unlike the input
event, the change
event doesn’t necessarily fire every time the value of an element changes.
Depending on the type of element being changed and how the user interacts with the element, the change
event is fired at different times.
- If a
checkbox
input item is checked or unchecked by clicking or using the keyboard. - If a
radio
input element is activated, but not if it is not activated. - If the user explicitly confirms the change, for example, selecting a value from a selection dropdown with a mouse click.
- If that element loses focus after its value is changed but not committed, e.g., after editing the value of
textarea
or thetext
input type.
Example:
<select id="osDemo" onchange="detectChange(this)">
<option value="Linux">Linux</option>
<option value="Windows">Windows</option>
<option value="MacOS">MacOS</option>
</select>
function detectChange(selectOS) {
console.log(selectOS.value)
}
We have given a dropdown of all the OS in the code above. Once the user selects any OS, the browser will detect the change, and the onChange
event is fired.
Users can also listen to the onChange
event of the select
element using the element’s id.
We listen to the node whose id is osDemo
in the code below. And once the browser detects the change, the onChange
event is fired.
You can choose any methods to listen to the onChange
event of the select
element. The output of both the code will be similar, as shown below.
document.getElementById('osDemo').onchange = function() {
console.log('document', document.getElementById('osDemo').value);
};
Output:
"document", "MacOS"
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn