How to Scroll to Specific Element in JavaScript
-
Use the
scroll()
Function to Scroll to an Element in JavaScript -
Use the
href
Property to Scroll to an Element in JavaScript -
Use the
scrollIntoView()
Function to Scroll to an Element in JavaScript
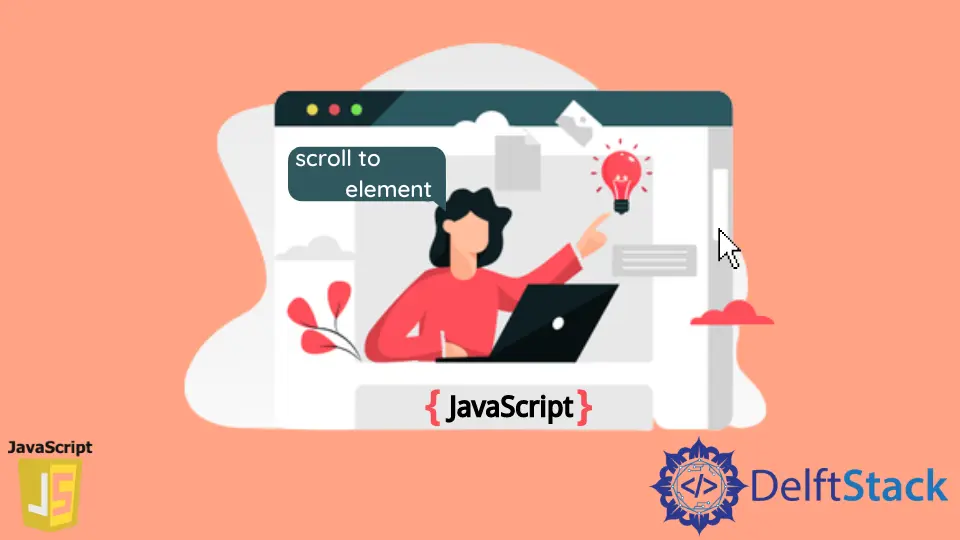
We will discuss how to get the specified element in the visible area of the browser window in different browsers using JavaScript.
The methods to accomplish this task are as follows.
Use the scroll()
Function to Scroll to an Element in JavaScript
The element interface’s scroll()
function scrolls to a specific set of coordinates within a given element. This is suitable for Chrome and Firefox and not for the rest.
window.scroll(0, findPos(document.getElementById('myDiv')));
We get the required element using the getElementById()
method. We pass this in the scroll()
function to scroll to that required element.
Use the href
Property to Scroll to an Element in JavaScript
Here we can set the href
value to point to an anchor in the webpage in JavaScript.
location.href = '#';
location.href = '#myDiv';
Here #myDiv
is the ID of the required <div>
tag.
Use the scrollIntoView()
Function to Scroll to an Element in JavaScript
The scrollIntoView()
function moves the supplied element into the browser window’s viewable region. This is most suitable for IE, Safari, and Edge users.
For example,
document.querySelector('#myDiv').scrollIntoView({behavior: 'smooth'});
The behavior
property describes how the scroll should look. In our example, it is set to smooth
. This property can be used in the scroll()
function as well.