Rock Paper and Scissors in JavaScript
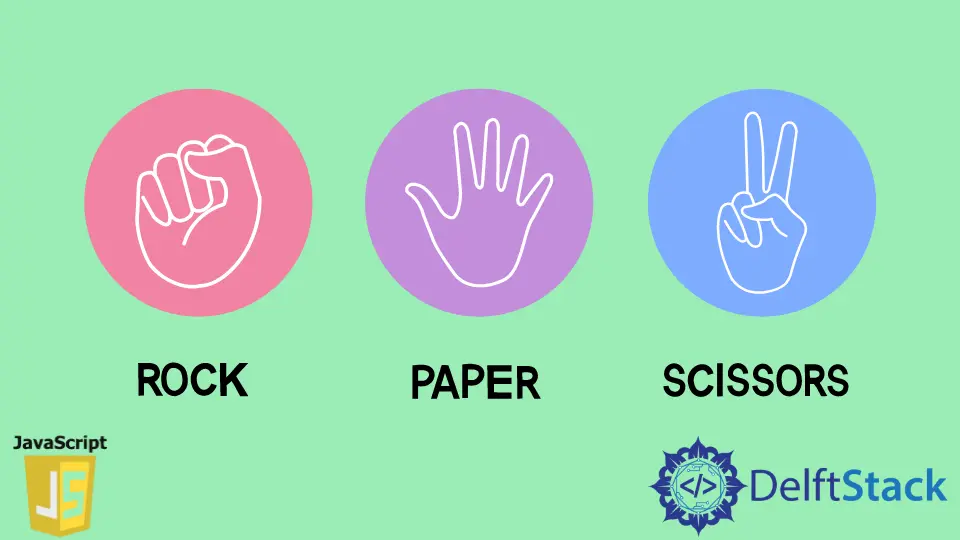
Every developer starts with the Hello world
somewhere in their life. Rock paper scissor is one popular game developers builds while learning any programming language. But writing multiple cases becomes a headache for developers. So in this article, we will learn the logic behind the rock, paper, and scissors game.
Rock, Paper, and Scissors in JavaScript
It is an interesting and popular game played by kids. And so does its logic. So let’s first understand the rule for this game.
- A player who chooses to play the rock will strike another player who chose the scissors because the stone crushes the scissors or sometimes chamfered scissors.
- A player who chooses to play rock loses to another who played the paper because the paper covers the rock.
- A player who decides to play paper will lose to a player of scissors because scissors cut paper.
So rules are cleared; now, let’s write the logic for the game. We have created one object with key as individual choice and values as the second choice. For example, if user input is rock and the computer’s choice is also rock, then it’s a draw.
let choicesObject = {
'rock': {'rock': 'draw', 'scissor': 'win', 'paper': 'lose'},
'scissor': {'rock': 'lose', 'scissor': 'draw', 'paper': 'win'},
'paper': {'rock': 'win', 'scissor': 'lose', 'paper': 'draw'}
}
Now we can use the above object as a global rule and predict the result of a game using function.
function checker(input) {
var choices = ['rock', 'paper', 'scissor'];
var num = Math.floor(Math.random() * 3);
let computerChoice = choices[num];
let result
switch (choicesObject[input][computerChoice]) {
case 'win':
result = 'YOU WIN';
break;
case 'lose':
result = 'YOU LOSE';
break;
default:
result = 'DRAW';
break;
}
console.log(result);
document.getElementById('result').textContent = result;
}
In the above function, we take user choice as input and compare the user input with computer-generated choice using a switch case. If none of the conditions is matched, it’s considered to be drawn. Now let’s take the user’s choice and print the result.
<button onClick="checker('rock')">Rock</button>
<button onClick="checker('paper')">Paper</button>
<button onClick="checker('scissor')">Scissor</button>
<p id="result">
</p>
When you run the above code, it will print something like this. The result may vary based on the computer’s choice.
Output:
YOU LOSE
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn