How to Convert RGB to HEX in JavaScript
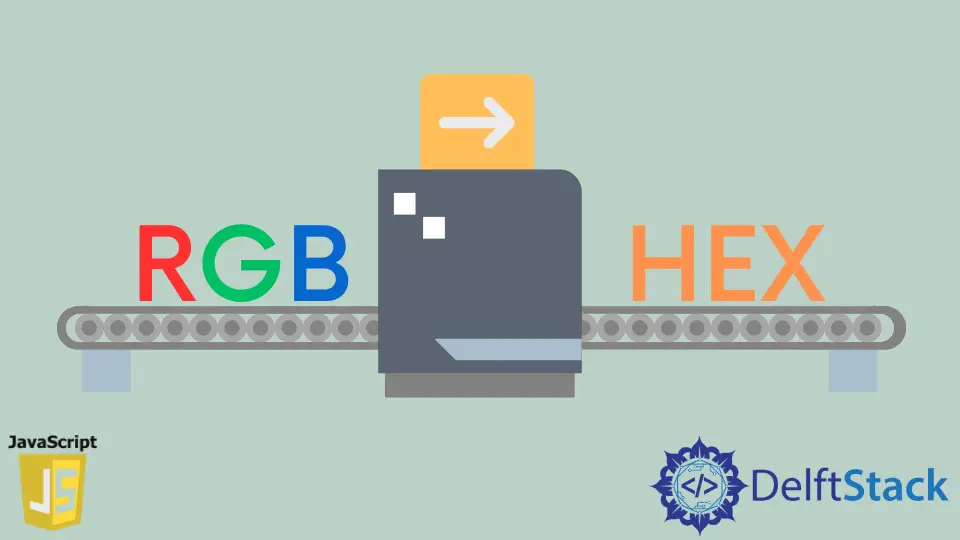
This tutorial will discuss how to convert RGB to HEX using the toString()
function in JavaScript.
Convert RGB to HEX Using the toString()
Function in JavaScript
The conversion between different color spaces is difficult in JavaScript because there is no predefined function present in JavaScript, which can convert between different color spaces.
So, we have to make our own conversion function to convert one color space into another. To make a conversion function, we need to know the difference between the two color spaces.
For example, the difference between the RGB and HEX color space is that the RGB is in decimal format, and the HEX is in the hexadecimal format. To convert from RGB to HEX, we only need to convert the decimal number of each color from RGB to hexadecimal and then concatenate them together to create the equivalent hex color space.
To change a value from decimal to hexadecimal, we can use the toString(16)
function. If the length of the converted number is 1
, we have to add a 0
before it. See the code below.
function ColorToHex(color) {
var hexadecimal = color.toString(16);
return hexadecimal.length == 1 ? '0' + hexadecimal : hexadecimal;
}
function ConvertRGBtoHex(red, green, blue) {
return '#' + ColorToHex(red) + ColorToHex(green) + ColorToHex(blue);
}
console.log(ConvertRGBtoHex(255, 100, 200));
Output:
#ff64c8
We can also convert from HEX to RGB using the parseInt()
function. A HEX color space contains 6 digits, excluding the first digit. We need to get the first 2 digits and convert them into the decimal format using the parseInt()
function, which will be our red color. Similarly, the next two digits will give us the green color, and the remaining will give us the blue color.
For example, let’s convert the HEX color to RGB using the parseInt()
function. See the code below.
var hex = '#ff64c8';
var red = parseInt(hex[1] + hex[2], 16);
var green = parseInt(hex[3] + hex[4], 16);
var blue = parseInt(hex[5] + hex[6], 16);
console.log(red, green, blue);
Output:
255 100 200
You can also make a function using the code above so that you don’t have to write the code again. Similarly, you can convert any color space to another; you just need to understand the difference between different color spaces.