How to Reset Button in JavaScript
- Understanding the Reset Button in HTML
- Implementing a Reset Button with JavaScript
- Customizing the Reset Functionality
- Conclusion
- FAQ
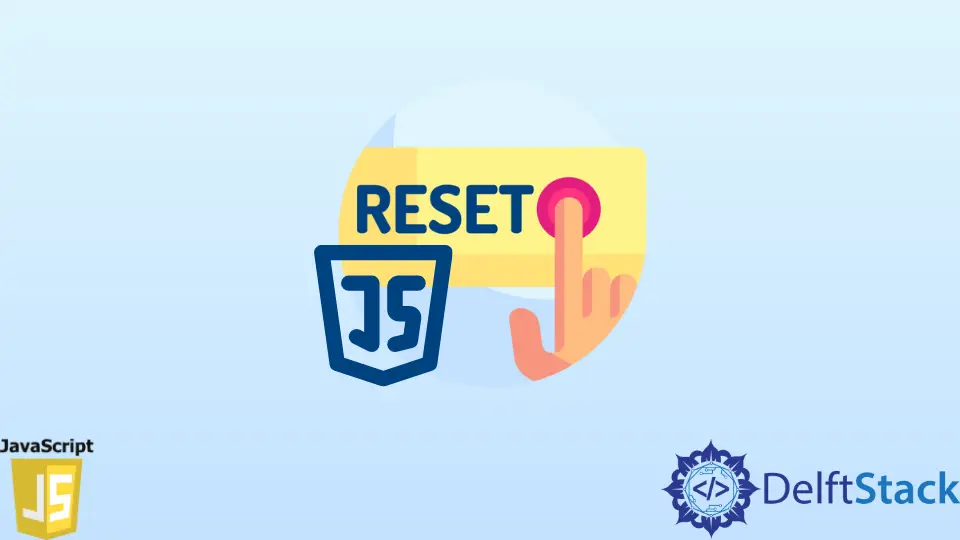
In today’s post, we’ll learn about the reset button in JavaScript. This feature is especially useful in forms, allowing users to clear all input fields and restore them to their default values with a single click. Understanding how to implement a reset button can enhance user experience significantly, especially in web applications where forms are a common interaction point. Whether you are a beginner or looking to brush up on your skills, this guide will walk you through the process of creating a reset button in JavaScript, complete with examples and explanations. Let’s dive in!
Understanding the Reset Button in HTML
Before jumping into the JavaScript implementation, it’s essential to understand the HTML structure of a reset button. In HTML forms, the reset button can be created using the <input>
element with the type attribute set to “reset”. This button, when clicked, will clear all the form inputs back to their initial values.
Here’s a simple example of an HTML form with a reset button:
htmlCopy<form id="myForm">
<label for="name">Name:</label>
<input type="text" id="name" name="name"><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email"><br><br>
<input type="reset" value="Reset">
</form>
Output:
textCopyAn HTML form with fields for name and email, and a reset button.
In this example, the reset button will clear the text fields when clicked. However, to add more functionality, we can utilize JavaScript to enhance this feature, allowing for more refined control over the form elements.
Implementing a Reset Button with JavaScript
To make our reset button more interactive, we can add a JavaScript function that executes when the button is clicked. This function can be used to reset specific fields or perform additional actions, such as displaying a message or logging the reset event.
Here’s how you can implement this:
htmlCopy<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Reset Button Example</title>
<script>
function resetForm() {
document.getElementById("myForm").reset();
alert("Form has been reset!");
}
</script>
</head>
<body>
<form id="myForm">
<label for="name">Name:</label>
<input type="text" id="name" name="name"><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email"><br><br>
<input type="button" value="Reset" onclick="resetForm()">
</form>
</body>
</html>
In this example, we replaced the default reset button with a custom button that triggers the resetForm
function when clicked. The function first resets the form using the reset()
method, which clears all input fields. Additionally, it displays an alert to inform the user that the form has been reset. This approach not only resets the form but also enhances user interaction.
Customizing the Reset Functionality
Sometimes, you may want more control over what happens when the reset button is clicked. For instance, you might want to reset only certain fields or perform other actions based on user input. Below is an example of how to customize the reset functionality.
htmlCopy<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Custom Reset Button Example</title>
<script>
function customReset() {
document.getElementById("name").value = "";
document.getElementById("email").value = "";
alert("Selected fields have been reset!");
}
</script>
</head>
<body>
<form id="myForm">
<label for="name">Name:</label>
<input type="text" id="name" name="name"><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email"><br><br>
<input type="button" value="Reset Selected Fields" onclick="customReset()">
</form>
</body>
</html>
In this example, the customReset
function specifically targets the “name” and “email” fields, setting their values to an empty string. This allows for more tailored control over the reset process. The alert notifies users that the selected fields have been reset, enhancing the overall experience.
Conclusion
In summary, implementing a reset button in JavaScript is a straightforward process that can significantly improve user experience in web forms. By utilizing HTML and JavaScript, you can create a reset button that not only clears fields but also provides additional functionality, such as alerts or customized resets. Whether you’re a beginner or an experienced developer, mastering this feature will undoubtedly enhance your web development skills. Keep experimenting with different functionalities to find what works best for your applications.
FAQ
-
What is a reset button in JavaScript?
A reset button in JavaScript is a feature in forms that allows users to clear all input fields back to their default values. -
How do I add a reset button to my HTML form?
You can add a reset button by using the<input>
element with the type attribute set to “reset”. You can also create a custom button and use JavaScript for added functionality. -
Can I customize the reset functionality?
Yes, you can customize the reset functionality using JavaScript to target specific fields or perform additional actions when the reset button is clicked. -
Is it possible to reset only certain fields in a form?
Yes, you can write a JavaScript function that sets the value of specific fields to empty or their default values, allowing for partial resets. -
What are the benefits of using a reset button in forms?
A reset button enhances user experience by providing a quick way to clear input fields, reducing the likelihood of user errors and improving form usability.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn