How to Replace Characters With Underscore in JavaScript
-
Using
replace
Method to Replace Characters in JavaScript -
Combining
split
andjoin
Methods in JavaScript
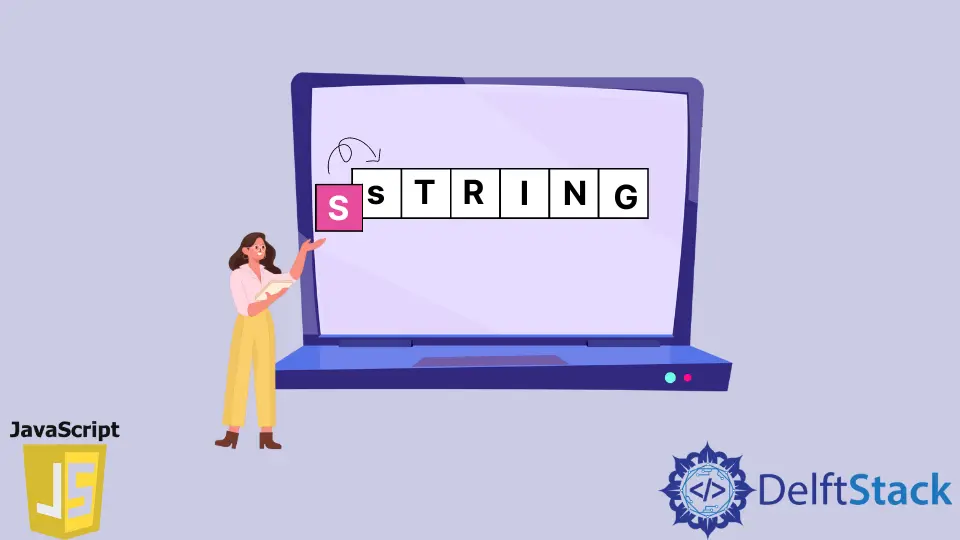
This tutorial teaches how to replace specific characters/substrings with other strings in JavaScript. We can use the replace
method to replace a substring with an alternate substring.
Using replace
Method to Replace Characters in JavaScript
The method replace
returns a new string by replacing a substring or a pattern with the replacement string.
For the replace
function, we should pass two parameters:
- Pattern or substring to be replaced.
- String which is to be replaced for the found pattern.
Example:
var str = 'Hi From delftstack .';
var replacedStr = str.replace(/\s+/g, '_');
console.log('str is : ' + str);
console.log('Replaced String is : ' + replacedStr);
Output:
str is : Hi From delftstack
Replaced String is: Hi_From_delftstack_.
In the above code,
- Created a string variable
str
with the valueHi From delftstack .
- Used the
replace
method to replace all the spaces in thestr
variable with the underscore (_
) character. For thereplace
function, the regex/\s+/g
is passed as the pattern. In this regex,\s+
will match all the space characters, and theg
flag (global flag) is included to match all the pattern occurrences instead of stopping after the first match. - The
replace
method will return a new string that will replace the spaces with an underscore (_
). The value in the replaced string will beHi_From_delftstack_.
Combining split
and join
Methods in JavaScript
We can achieve the same by combining two methods, split
and join
.
The split
method will create an array by splitting the string into multiple substrings. The string will be split for all the regex-pattern/string
matches passed as an argument.
The method join
returns a new string created by joining all the array elements. A separator string will be added between each element joining the string (by default, comma ,
will be added).
Example:
var str = 'Hi From delftstack .';
var splitArr = str.split(/\s+/g);
var replacedStr = splitArr.join('_');
console.log('str is : ' + str);
console.log('splitArr is : ', splitArr);
console.log('Replaced String is : ' + replacedStr);
Output:
str is : Hi From delftstack
splitArr is : ['Hi', 'From', 'delftstack', '.']
Replaced String is: Hi_From_delftstack_.
In the above code,
- Created a string variable
str
with the valueHi From delftstack .
- Used the
split
method to with/\s+/g
regex as an argument. Thesplit
method will divide the string for each match of the space characters. Thesplit
method will return['Hi', 'From', 'delftstack', '.']
. - Used the
join
method to join the split strings array with the underscore_
as a separator string and will join all the string elements by adding the underscore between each element. Thejoin
method will returnHi_From_delftstack_.
.
Related Article - JavaScript String
- How to Get Last Character of a String in JavaScript
- How to Convert a String Into a Date in JavaScript
- How to Get First Character From a String in JavaScript
- How to Convert Array to String in JavaScript
- How to Check String Equality in JavaScript
- How to Filter String in JavaScript