How to Trim Whitespace in JavaScript
-
Understanding the
trim()
Method - Trimming Whitespace in User Input
- Chaining trim() with Other String Methods
- Handling Edge Cases with trim()
- Conclusion
- FAQ
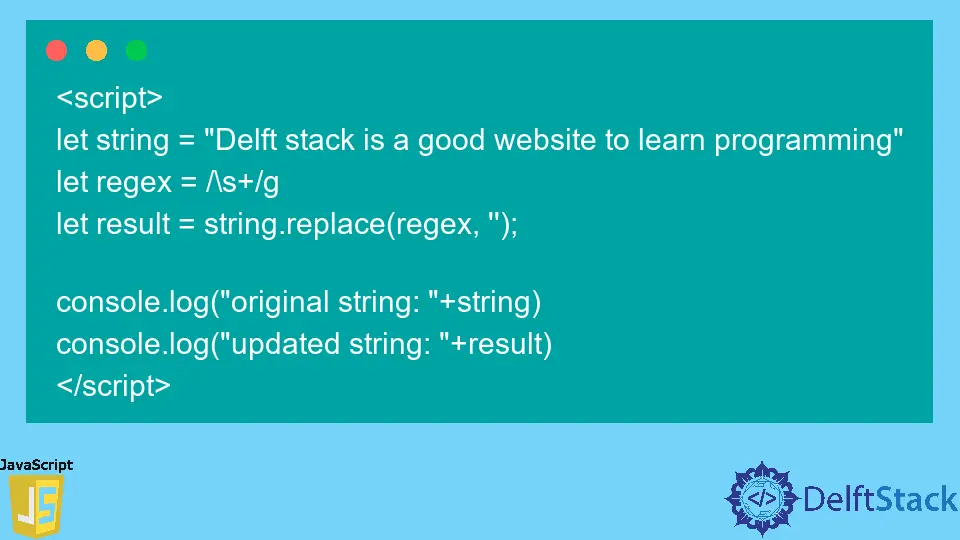
When working with strings in JavaScript, you often encounter unwanted whitespace—extra spaces, tabs, or newlines that can cause issues in your applications. Fortunately, JavaScript provides a simple and efficient way to handle this using the trim()
method. Introduced in ECMAScript 5 (ES5), this method removes whitespace from both ends of a string, ensuring your data is clean and ready for processing.
In this article, we will explore how to effectively use the trim()
method in JavaScript, along with practical examples to illustrate its functionality. Whether you’re a beginner or an experienced developer, understanding how to manage whitespace in strings is essential for writing clean and efficient code.
Understanding the trim()
Method
The trim()
method is a built-in JavaScript function that eliminates whitespace from the start and end of a string. This is particularly useful when dealing with user input, as users may inadvertently add extra spaces. The method does not modify the original string but returns a new string with the whitespace removed.
Here’s a simple example to illustrate how trim()
works:
let str = " Hello, World! ";
let trimmedStr = str.trim();
console.log(trimmedStr);
Output:
Hello, World!
In this example, the trim()
method effectively removes the spaces before and after “Hello, World!”, resulting in a cleaner output. This method is straightforward yet powerful, making it a staple for any JavaScript developer.
Trimming Whitespace in User Input
When accepting user input, it’s common for users to accidentally include extra spaces. By using the trim()
method, you can ensure that the input is clean before further processing. Here’s how you can implement this in a simple form validation scenario:
function validateInput(input) {
let trimmedInput = input.trim();
if (trimmedInput === "") {
console.log("Input cannot be empty.");
} else {
console.log("Valid input: " + trimmedInput);
}
}
validateInput(" "); // Empty input
validateInput(" Hello! "); // Valid input
Output:
Input cannot be empty.
Valid input: Hello!
In this example, the validateInput
function trims the input string. If the trimmed input is empty, it alerts the user. Otherwise, it confirms valid input. This is particularly useful in applications where user input is critical, such as registration forms or search bars.
Chaining trim() with Other String Methods
The trim()
method can be combined with other string methods for enhanced functionality. For instance, if you want to convert a string to lowercase after trimming, you can chain these methods together. Here’s how:
let userInput = " JavaScript IS Fun! ";
let processedInput = userInput.trim().toLowerCase();
console.log(processedInput);
Output:
javascript is fun!
By chaining trim()
with toLowerCase()
, the final output is a clean, lowercase string. This technique is invaluable when you need to standardize user input for comparison or storage, ensuring consistency across your application.
Handling Edge Cases with trim()
While the trim()
method is effective for most scenarios, it’s essential to consider edge cases. For instance, if a string consists solely of whitespace characters, the trim()
method will return an empty string. Here’s how you can handle such situations:
function handleWhitespace(input) {
let trimmedInput = input.trim();
if (trimmedInput.length === 0) {
console.log("Input is just whitespace.");
} else {
console.log("Processed input: " + trimmedInput);
}
}
handleWhitespace(" "); // Only whitespace
handleWhitespace(" Hello! "); // Valid input
Output:
Input is just whitespace.
Processed input: Hello!
In this example, the function checks the length of the trimmed input. If it’s zero, it indicates that the input contained only whitespace. This additional check enhances the robustness of your input handling, ensuring that your application behaves as expected across various scenarios.
Conclusion
Trimming whitespace in JavaScript is a fundamental skill that every developer should master. The trim()
method provides a simple yet effective way to clean up strings, particularly when dealing with user input. By understanding how to use this method and its variations, you can ensure that your applications handle data more effectively. Whether you’re validating forms or preparing strings for processing, the trim()
method is an essential tool in your JavaScript toolkit.
FAQ
-
What does the trim() method do in JavaScript?
Thetrim()
method removes whitespace from both ends of a string. -
Can trim() remove whitespace from the middle of a string?
No, thetrim()
method only removes whitespace from the beginning and end of a string. -
Is trim() a destructive method?
No,trim()
does not modify the original string; it returns a new string instead. -
What happens if I call trim() on an empty string?
Callingtrim()
on an empty string will return an empty string. -
Can I chain trim() with other string methods?
Yes, you can chaintrim()
with other string methods liketoLowerCase()
orsubstring()
for added functionality.