How to Generate Values in A Range in JavaScript
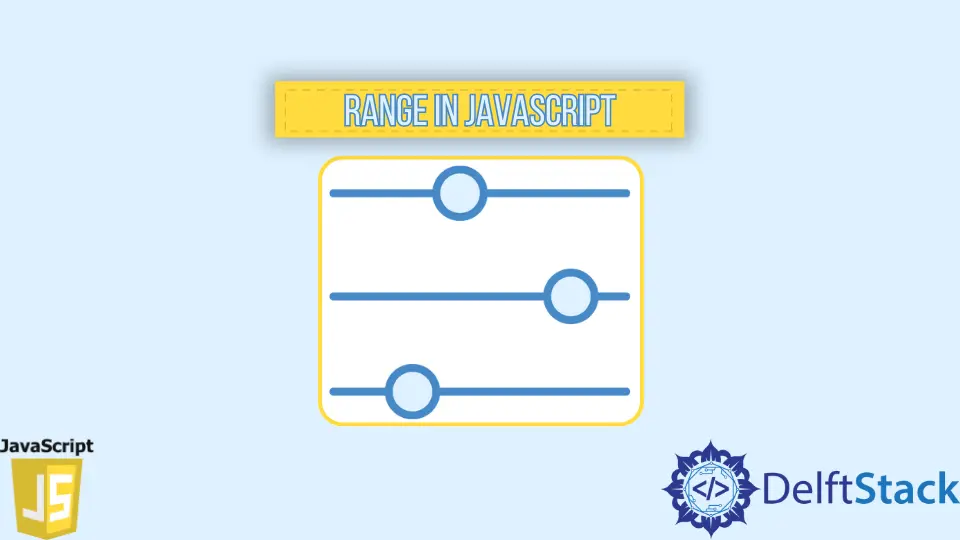
Generating values within a specified range is a common task in programming, and JavaScript offers several ways to achieve this. Whether you’re working on a simple project or a complex application, understanding how to generate numbers in a range can enhance your coding toolkit.
In this article, we will explore various methods to generate values in a given range using JavaScript. From traditional loops to modern ES6 features, we’ll cover it all. By the end of this guide, you’ll have a solid grasp of how to implement these techniques in your own projects, making your JavaScript skills even more robust.
Using a for
Loop
One of the most straightforward methods to generate values in a range is by using a traditional for
loop. This method allows you to specify the start and end points, and then increment through the range. Here’s how you can do it:
function generateRange(start, end) {
let values = [];
for (let i = start; i <= end; i++) {
values.push(i);
}
return values;
}
console.log(generateRange(1, 5));
Output:
[1, 2, 3, 4, 5]
In this example, the generateRange
function takes two parameters: start
and end
. It initializes an empty array called values
. The for
loop iterates from start
to end
, pushing each value into the values
array. Finally, the function returns the array containing all the numbers in the specified range. This method is simple yet effective for generating a consecutive sequence of numbers.
Using Array.from()
Another elegant way to generate values in a range is by using the Array.from()
method. This approach takes advantage of the power of array manipulation in JavaScript. Here’s how you can implement it:
function generateRange(start, end) {
return Array.from({ length: end - start + 1 }, (_, i) => start + i);
}
console.log(generateRange(1, 5));
Output:
[1, 2, 3, 4, 5]
In this method, Array.from()
creates a new array from an array-like or iterable object. We specify the length of the new array as end - start + 1
, ensuring we include both endpoints. The second argument is a mapping function that calculates each value based on the index i
. This method is concise and takes full advantage of JavaScript’s functional programming capabilities, making it a favorite among developers who prefer cleaner code.
Using the Spread Operator
The spread operator is another modern JavaScript feature that can also be leveraged to generate values in a range. This method combines the power of the map
function with the simplicity of the spread operator. Here’s an example:
function generateRange(start, end) {
return [...Array(end - start + 1)].map((_, i) => start + i);
}
console.log(generateRange(1, 5));
Output:
[1, 2, 3, 4, 5]
In this code snippet, we create an empty array with a length of end - start + 1
using the spread operator. Then, we use the map
function to fill this array with the appropriate values. The mapping function calculates each number by adding the index i
to the start
value. This method is both elegant and efficient, showcasing the flexibility of modern JavaScript syntax.
Using Recursion
If you’re looking for a more advanced technique, you can use recursion to generate values in a range. This method is less common but can be quite powerful. Here’s how it works:
function generateRange(start, end) {
if (start > end) return [];
return [start, ...generateRange(start + 1, end)];
}
console.log(generateRange(1, 5));
Output:
[1, 2, 3, 4, 5]
In this recursive function, we check if start
is greater than end
. If it is, we return an empty array, which serves as the base case. Otherwise, we create an array that includes the current start
value and concatenates it with the result of the recursive call where start
is incremented by 1. This method is a great demonstration of how recursion can be used to solve problems, although it may not be the most efficient for large ranges due to the call stack size.
Conclusion
Generating values in a range using JavaScript can be accomplished in various ways, each with its own advantages. Whether you choose a simple for
loop, the elegance of Array.from()
, the spread operator, or even recursion, understanding these methods can significantly enhance your coding skills. As you work on your projects, consider the context and requirements to choose the best approach for generating ranges. With these techniques in your toolkit, you’ll be well-equipped to tackle a wide range of programming challenges.
FAQ
-
What is the simplest way to generate a range of numbers in JavaScript?
The simplest way is to use afor
loop to iterate from the start to the end and push each number into an array. -
Can I generate a range of numbers with a specific step?
Yes, you can modify thefor
loop or other methods to include a step parameter, adjusting the increment accordingly. -
Is recursion a good method for generating ranges?
While recursion can be used to generate ranges, it may not be the most efficient method for large ranges due to potential stack overflow issues. -
What is the difference between
Array.from()
and the spread operator?
Array.from()
creates a new array from an array-like or iterable object, while the spread operator expands elements from an iterable into a new array. -
Can I generate non-integer ranges in JavaScript?
Yes, you can generate non-integer ranges by modifying the increment in your loop or mapping function to a decimal value.