How to Prevent Form Submission JavaScript
-
Use a Function Within the
script
Tag to Stop the Execution of a Form in JavaScript - Use the Vanilla JavaScript to Stop the Execution of the Form in JavaScript
-
Use the
jQuery
Library to Stop the Execution of the Form in JavaScript - Use the Dojo Toolkit to Stop the Execution of the Form in JavaScript
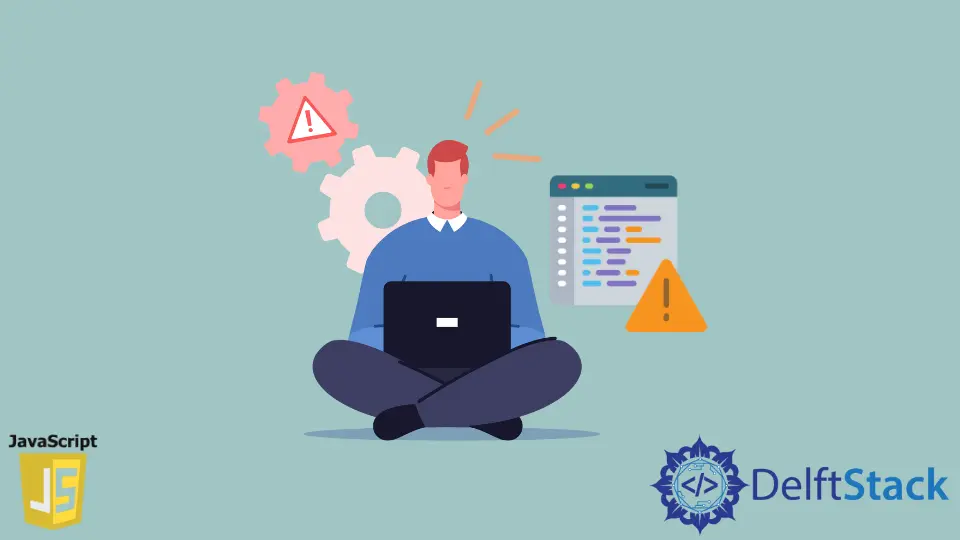
JavaScript is supported by all web browsers and is used to make dynamic web pages. In this tutorial, we will stop the execution of a form using JavaScript.
Use a Function Within the script
Tag to Stop the Execution of a Form in JavaScript
The script
tag is used to contain a client-side script. We have created a JavaScript function within the script
tags to prevent the submission of the form.
Check the code below.
<form onsubmit="submitForm(event)">
<input type="text">
<button type="submit">Submit</button>
</form>
<script type="text/JavaScript">
function submitForm(event){
event.preventDefault();
window.history.back();
}
</script>
The preventDefault()
function does not let the default action of the event take place. The window.history.back()
takes us to the previous URL in our history. All the methods discussed will include these two functions.
Use the Vanilla JavaScript to Stop the Execution of the Form in JavaScript
Vanilla JavaScript works purely on JavaScript without the use of any additional libraries.
An event listener can be used to prevent form submission. It is added to the submit button, which will execute the function and prevent a form from submission when clicked.
For example,
element.addEventListener('submit', function(event) {
event.preventDefault();
window.history.back();
}, true);
Use the jQuery
Library to Stop the Execution of the Form in JavaScript
jQuery
is a fast and robust library that simplifies the use of JavaScript. It is one of the most popular libraries of JavaScript.
We will see how we can it to prevent the submission of the form.
Check the code below.
$('#form').submit(function(event) {
event.preventDefault();
window.history.back();
});
The $('#form').submit()
function is used to submit a form in jQuery.
Use the Dojo Toolkit to Stop the Execution of the Form in JavaScript
It is a JavaScript library that helps ease the cross-platform use of JavaScript for web-based applications. It uses the dojo.connect()
function to handle events. In our case, this is used for form submission, and we will prevent this using the preventDefault()
and window.history.back()
function.
For example,
dojo.connect(form, 'submit', function(event) {
event.preventDefault();
window.history.back();
});