JavaScript POST
-
Use
XHR(XML HTTP Request)
to SendPOST
Data Without a Form in JavaScript -
Use the
Fetch
API to SendPOST
Data Without a Form in JavaScript -
Use the
Navigator.sendBeacon()
to SendPOST
Data Without a Form in JavaScript
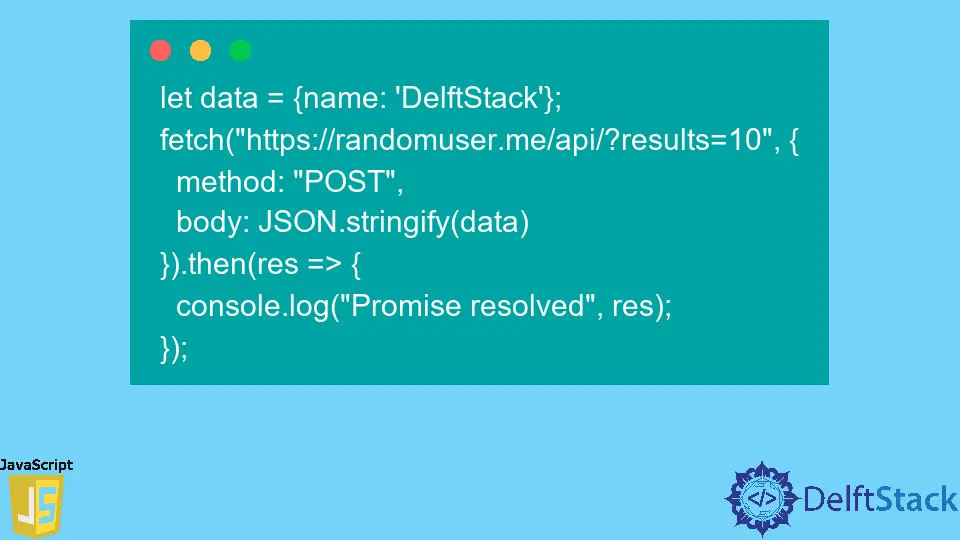
This tutorial teaches how to send post data without using a form in JavaScript.
Use XHR(XML HTTP Request)
to Send POST
Data Without a Form in JavaScript
XHR
is an object that is used to make HTTP
requests in JavaScript. It helps to interact with the server and to exchange the data between the client and the server. We can pull data from a server even without refreshing the entire page. It enables users to continue working by disrupting only a part of a page. We can use this method to send POST
data without using a form.
const URL = 'delftstack.com'
var xhr = new XMLHttpRequest();
xhr.open('POST', URL, true);
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.send(JSON.stringify({name: 'DelftStack'}));
Use the Fetch
API to Send POST
Data Without a Form in JavaScript
JavaScript Fetch API, like XHR
, helps to send HTTP
requests to the server. But XHR
did not make use of promises and lead to cluttered and unclean code. To use the Fetch API, we have to call the fetch()
method. It accepts the following parameters:
URL
: The URL of the API from which to request data.An object
: It is an object specifying additional properties about the type of request made. It has3
properties:method
: It specifies the method likeGET
,POST
, etc. Its value should bePOST
in this case since we are making aPOST
request.body
:body
object contains the data to be sent.headers
: It is an optional parameter that helps us to perform various actions onHTTP
request and response headers.
After the fetch()
method, we specify the promise method then()
and catch()
. If the promise
returned by fetch()
is resolved
, then the function specified in then()
is executed otherwise the promise
is rejected
and the function inside catch()
is called.
let data = {name: 'DelftStack'};
fetch('https://randomuser.me/api/?results=10', {
method: 'POST',
body: JSON.stringify(data)
}).then(res => {
console.log('Promise resolved', res);
});
Use the Navigator.sendBeacon()
to Send POST
Data Without a Form in JavaScript
Navigator.sendBeacon()
method helps send data to a web server over HTTP requests asynchronously. Its main application is to send website analytics data to the server, but it can also be used to send POST
data.
let data = {name: 'DelftStack'};
navigator.sendBeacon('https://randomuser.me/api/?results=10', data);
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn