How to Pass JavaScript Function as Parameter
- Understanding Functions as Parameters
- Using Anonymous Functions
- Arrow Functions for Conciseness
- Higher-Order Functions
- Conclusion
- FAQ
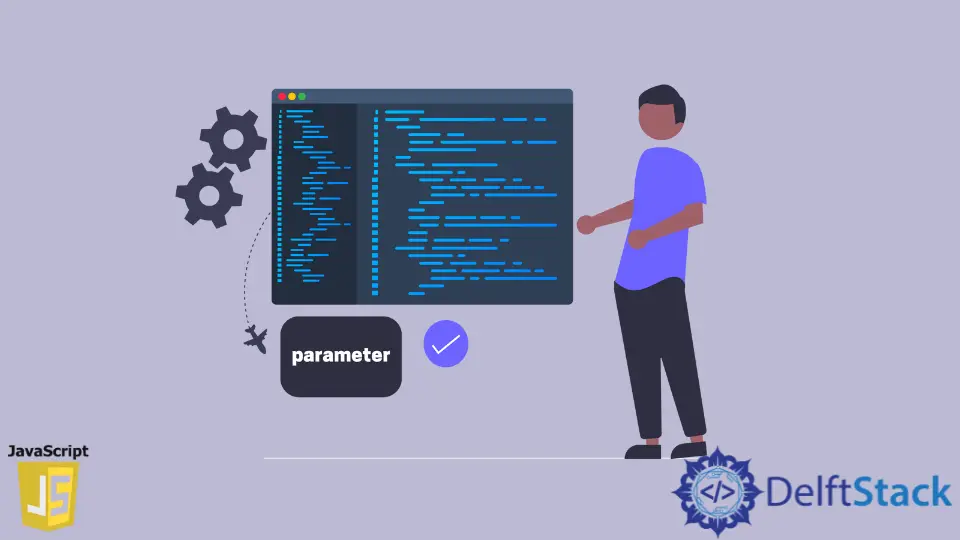
In the world of JavaScript, functions are first-class citizens, meaning they can be treated like any other value. One of the powerful features of JavaScript is the ability to pass functions as parameters to other functions. This capability opens up a realm of possibilities for creating flexible and reusable code. Whether you’re working with callbacks, higher-order functions, or event handling, understanding how to pass functions as parameters is crucial.
In this article, we will explore various methods to achieve this, complete with practical examples that demonstrate how to effectively use this feature in your JavaScript programming. Let’s dive in!
Understanding Functions as Parameters
At its core, passing a function as a parameter involves simply referencing the function without invoking it. This means you don’t use parentheses when passing the function. For instance, if you have a function named sayHello
, you would pass it as sayHello
instead of sayHello()
. This distinction is vital because using parentheses would execute the function immediately, rather than passing it as a reference for later use.
Here’s a simple example to illustrate this concept:
javascriptCopyfunction greet(name) {
return `Hello, ${name}!`;
}
function processUserInput(callback) {
const name = prompt("Please enter your name.");
console.log(callback(name));
}
processUserInput(greet);
In this example, the greet
function is passed to processUserInput
as a parameter. The processUserInput
function calls greet
with the user’s input, demonstrating how functions can be passed and executed later.
Output:
textCopyHello, John!
The processUserInput
function prompts the user for their name and then calls the greet
function, displaying a personalized greeting. This is just a glimpse of how powerful passing functions as parameters can be in JavaScript.
Using Anonymous Functions
Another common approach is to use anonymous functions, which are functions without a name. This technique is especially useful for one-time operations where you don’t need to reuse the function elsewhere. Here’s how you can implement an anonymous function as a parameter:
javascriptCopyfunction processArray(arr, callback) {
for (let i = 0; i < arr.length; i++) {
callback(arr[i]);
}
}
const numbers = [1, 2, 3, 4, 5];
processArray(numbers, function(num) {
console.log(num * 2);
});
In this example, the processArray
function takes an array and a callback function. The callback is an anonymous function that doubles each number in the array. By passing this function as a parameter, you can easily manipulate the array without having to define a separate named function.
Output:
textCopy2
4
6
8
10
Using anonymous functions allows for more concise code, particularly when the operation is simple and doesn’t require a dedicated function. This method emphasizes the flexibility of JavaScript functions and showcases how they can be tailored to specific tasks.
Arrow Functions for Conciseness
With the introduction of ES6, arrow functions became a popular way to write functions in a more concise manner. They provide a shorter syntax and can be particularly useful when passing functions as parameters. Here’s an example:
javascriptCopyconst numbers = [1, 2, 3, 4, 5];
const double = (num) => num * 2;
const processArray = (arr, callback) => {
arr.forEach(callback);
};
processArray(numbers, double);
In this case, we define a simple arrow function double
that doubles a number. The processArray
function uses the forEach
method to iterate over the array, applying the callback to each element. This approach is clean and leverages modern JavaScript features for better readability.
Output:
textCopy2
4
6
8
10
Arrow functions not only simplify the syntax but also maintain the lexical scope of this
, making them an excellent choice for many scenarios in JavaScript. They enhance code clarity and reduce the verbosity often associated with traditional function expressions.
Higher-Order Functions
A higher-order function is a function that either takes one or more functions as arguments or returns a function as its result. This concept is fundamental in functional programming and is widely used in JavaScript. Let’s take a look at how to implement a higher-order function:
javascriptCopyfunction createMultiplier(multiplier) {
return function(num) {
return num * multiplier;
};
}
const double = createMultiplier(2);
const triple = createMultiplier(3);
console.log(double(5));
console.log(triple(5));
In this example, createMultiplier
is a higher-order function that returns a new function, which multiplies a given number by the specified multiplier. We create two functions, double
and triple
, which can be used to multiply numbers by 2 and 3, respectively.
Output:
textCopy10
15
Higher-order functions are powerful because they allow for more abstract and reusable code. They enable you to create specialized functions on the fly, promoting code modularity and reusability.
Conclusion
Passing functions as parameters in JavaScript is a fundamental concept that can greatly enhance your coding capabilities. Whether using named functions, anonymous functions, or arrow functions, the flexibility offered by JavaScript’s first-class functions allows for elegant and efficient code. By understanding how to effectively pass and manipulate functions, you can create more dynamic and reusable code structures that can adapt to various programming challenges. Embrace this powerful feature, and watch your JavaScript skills soar!
FAQ
- What is a first-class function in JavaScript?
A first-class function is a function that can be treated as a value, meaning it can be assigned to variables, passed as arguments, or returned from other functions.
-
How do I pass multiple functions as parameters?
You can pass multiple functions as parameters by simply separating them with commas when calling the function, likeprocessUserInput(greet, anotherFunction)
. -
What are anonymous functions used for?
Anonymous functions are often used for one-time operations where a named function is not necessary, making the code more concise and easier to read. -
Why use arrow functions in JavaScript?
Arrow functions provide a shorter syntax and maintain the lexical scope ofthis
, making them particularly useful in callback functions and methods. -
Can I return a function from another function in JavaScript?
Yes, JavaScript allows functions to return other functions, enabling the creation of higher-order functions and closures.
Related Article - JavaScript Function
- JavaScript Return Undefined
- Self-Executing Function in JavaScript
- How to Get Function Name in JavaScript
- JavaScript Optional Function Parameter
- The Meaning of => in JavaScript
- Difference Between JavaScript Inline and Predefined Functions