The @param Tag in JavaScript
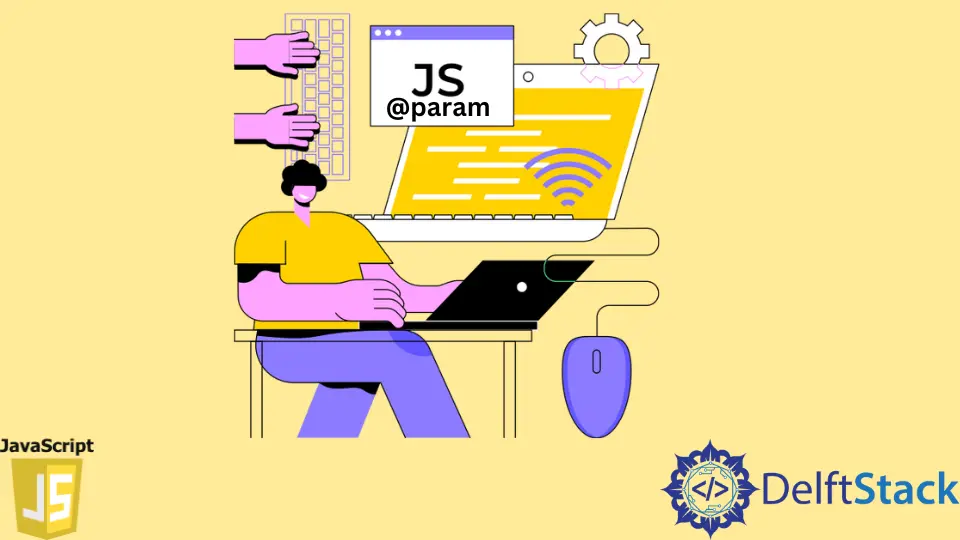
In this article, we will learn and use the @param
tag in JavaScript source code. This tag is used for the documentation of a source code.
These types of tags are useful for programmers to understand the execution flow of code.
the @param
Tag in JavaScript
In JavaScript source code documentation, @param
tags provide the various details of function parameters like name, type and description. The @param
tag comes up with the description of function parameters.
If we separate the @param
tag, it will divide into three parts.
- First is within curly braces
{}
, which can be used to define the data type of parameter. - The second is the name of a parameter.
- The last part is the parameter’s description with the hyphen
-
sign separation.
The basic syntax of @param
:
/**
* @param {data type of param} param name - description of param.
*/
function functionName(paramName) {
alert('Hello ' + paramName);
}
The @param
tag requires developers to define the parameter details per standards. Providing parameter descriptions according to those standards can make JavaScript doc comments more readable and understandable.
The parameter can be a built-in JavaScript type; it can be a string or object.
Parameters With Properties
Suppose the parameter is anticipated to have a specific property. We can document it with a further @param
tag; if a student parameter contains name and class properties, we can document it as shown below.
<script>
/**
* Assign the class to the student.
* @param {Object} student - The student who is a part of class.
* @param {string} student.name - The name of the student.
* @param {string} student.class - The student's class.
*/
function studentData(student) {
// rest operation of function
};
</script>
Parameters With Callback
We can use the @callback
tag to define a callback type if the parameter accepts a callback function. After that, we can include the callback type in the @param
tag, as shown below.
<script>
/**
* @callback callbackRequest
* @param {number} statusCode
* @param {string} message
*/
/**
* asynchronous task and on completion executes the callback.
* @param {callbackRequest} callBack - The callback for handle the result.
*/
function asynchronousTask(callBack) {
// rest operation of function
};
</script>