How to Open URL in a New Window With JavaScript
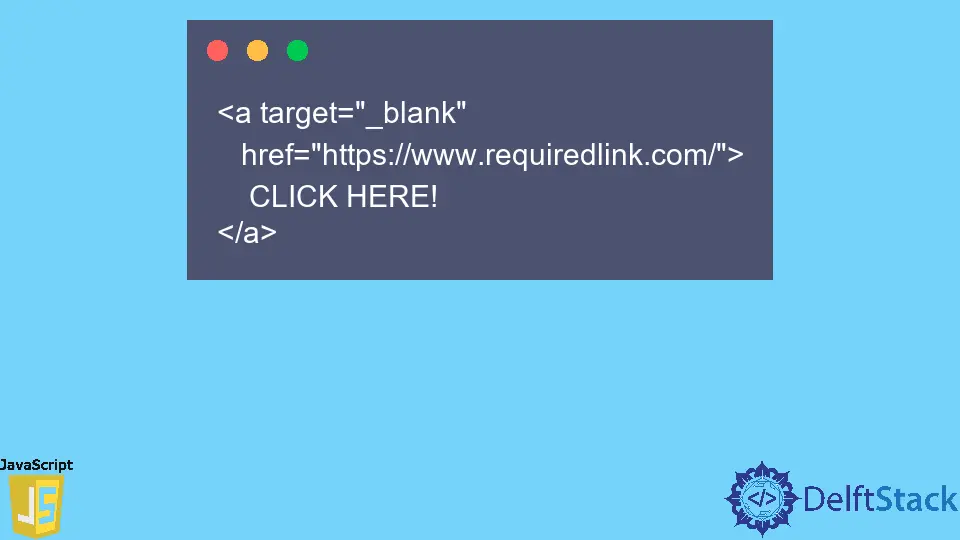
In this tutorial, we open a URL in a new window using JavaScript.
The links that take users to different sites should open in new tabs or windows. Hence, a few methods are given below for reference.
The window.open()
function creates a secondary browser window. We can specify the required website URL within the function. It also allows us to control certain features of the newly created window using parameters like height
, width
, scrollbars
, and more.
See the following example.
<a href="" onclick="window.open('https://www.required-url.com/', '_blank', 'location=yes,height=570,width=520,scrollbars=yes,status=yes');">
CLICK HERE!
</a>
In the above example, we have a hyperlink tag in HTML, which executes the window.open()
function on clicking. Note the _blank
in the above example. It ensures a new tab is opened in the window.
We can also open a URL in a new tab. For this, we do not need the window.open()
function. We only set the target
parameter as target="_blank"
in the hyperlink tag, and it will always open URLs in a new tab.
For example,
<a target="_blank"
href="https://www.requiredlink.com/">
CLICK HERE!
</a>