Nested for Loops JavaScript
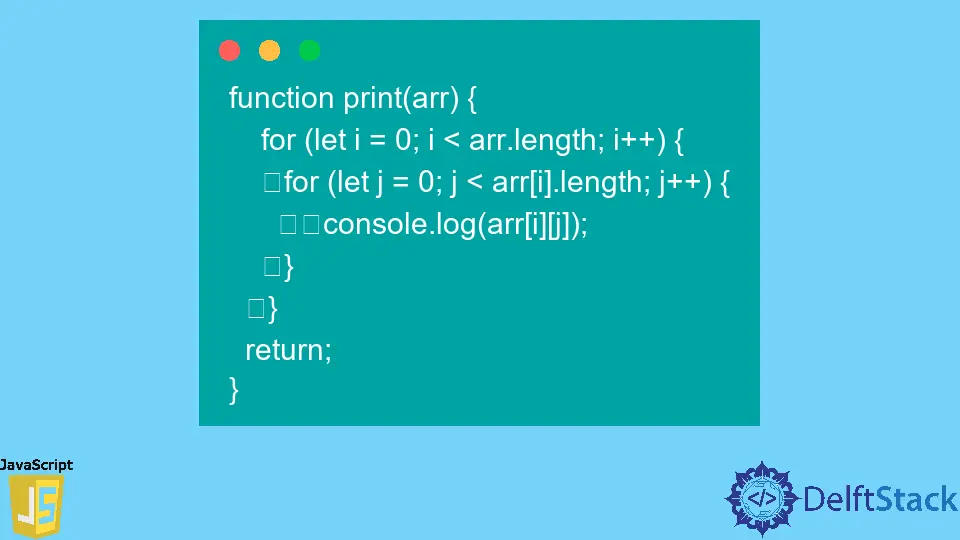
This tutorial explains the concept of nested loops in JavaScript. A loop is a programming structure used to iterate through a series of data or perform the same action repetitively until a specific condition is met or for a certain amount of time without explicitly writing the code again and again. A nested for
loop is a composition of loops. We can have one or more loops present inside a loop. The loop that is nested is called the inner loop and the loop containing the nested loop is called the outer loop.
The execution always begins with the outer loop and then moves down the nested loops. The inner loops are executed completely in each iteration of the outer loop. We can broadly define the syntax for a nested loop as:
Outerloop {
Innerloop {
// statements to execute inside inner loop
}
// statements to execute inside outer loop
}
The loop can be of any type like for
loop, while
loop, or do-while
loop.
Let us consider a 2D array arr[2][3] = [[1,2],[3,4],[5,6]]
and we want to print all the elements. We will need two loops for this. One to iterate all the subarrays and iterate all the elements inside those subarrays.
function print(arr) {
for (let i = 0; i < arr.length; i++) {
for (let j = 0; j < arr[i].length; j++) {
console.log(arr[i][j]);
}
}
return;
}
Output:
1
2
3
4
5
6
We visit iterate over all the subarrays present inside the array.
-
First Iteration
i=0:
-
Inner loop iteration 1:
j=0
Print 1 -
Inner loop iteration 2:
j=1
Print 2
-
-
Second Iteration
i=1:
-
Inner loop iteration 1:
j=0
Print 3 -
Inner loop iteration 2:
j=1
Print 4
-
-
Third Iteration
i=2:
-
Inner loop iteration 1:
j=0
Print 5 -
Inner loop iteration 2:
j=1
Print 6
-
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn