NEGATIVE_INFINITY in JavaScript
- What is NEGATIVE_INFINITY?
- How to Use NEGATIVE_INFINITY in JavaScript
- Practical Applications of NEGATIVE_INFINITY
- Conclusion
- FAQ
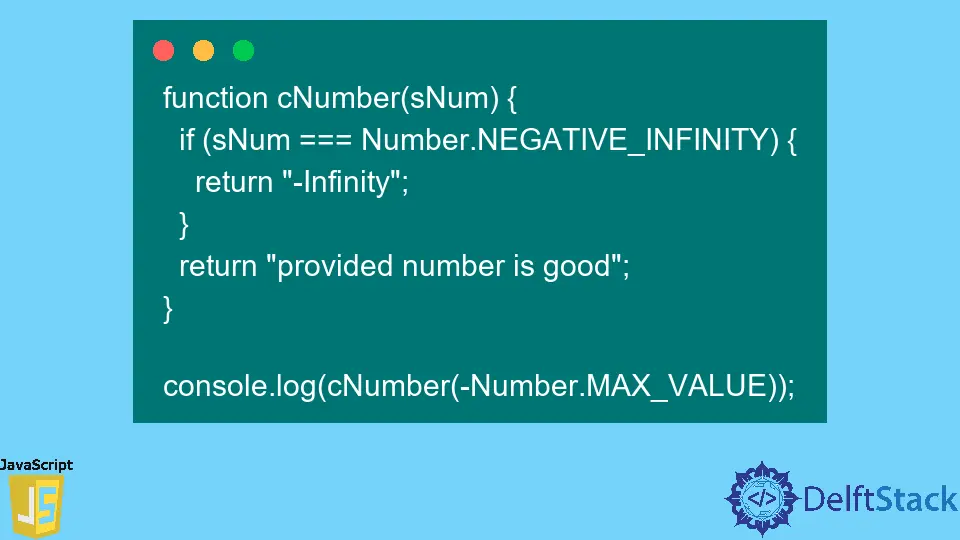
JavaScript is a powerful and versatile programming language, and one of its intriguing features is the concept of NEGATIVE_INFINITY. This special value represents the lowest possible number in JavaScript, serving as a useful tool in various programming scenarios. Whether you’re dealing with mathematical calculations, error handling, or simply want to understand how JavaScript processes numbers, grasping the concept of NEGATIVE_INFINITY can significantly enhance your coding skills.
In this article, we will delve into what NEGATIVE_INFINITY is, how it works, and its practical applications in your JavaScript projects. Let’s explore this fascinating aspect of JavaScript together!
What is NEGATIVE_INFINITY?
In JavaScript, NEGATIVE_INFINITY is a predefined global property that signifies a value less than any finite number. It is a part of the Number object and can be accessed directly as Number.NEGATIVE_INFINITY
. This value is often the result of mathematical operations that exceed the lower limits of what numbers can represent, such as dividing a negative number by zero.
NEGATIVE_INFINITY is not just a placeholder; it plays a crucial role in error handling and control flow within your applications. When you encounter situations where a number goes beyond the lower boundary, NEGATIVE_INFINITY can help you manage those scenarios effectively.
How to Use NEGATIVE_INFINITY in JavaScript
Using NEGATIVE_INFINITY in your JavaScript code is straightforward. You can utilize it in mathematical operations, comparisons, or as a default value in functions. Here are a few practical examples to illustrate its use.
Example 1: Basic Usage
let value = -10 / 0;
console.log(value);
Output:
- Infinity
In this example, dividing -10 by 0 yields NEGATIVE_INFINITY. This behavior is consistent across all browsers and JavaScript environments, making it reliable for developers.
Example 2: Comparisons
let lowestValue = Number.NEGATIVE_INFINITY;
console.log(lowestValue < -100); // true
console.log(lowestValue > -100); // false
Output:
true
false
Here, we see how NEGATIVE_INFINITY can be used in comparisons to determine if it is less than or greater than other numbers. This can be particularly useful in algorithms that require finding minimum values.
Example 3: Default Value in Functions
function findMin(numbers) {
let minValue = Number.NEGATIVE_INFINITY;
for (let number of numbers) {
if (number > minValue) {
minValue = number;
}
}
return minValue;
}
console.log(findMin([-5, -10, -3, -1]));
Output:
-1
In this function, we initialize minValue
to NEGATIVE_INFINITY. As we iterate through the array, we update minValue
whenever we find a larger number. This approach ensures that even if all numbers are negative, the function will still return the correct minimum value.
Practical Applications of NEGATIVE_INFINITY
Understanding NEGATIVE_INFINITY opens up a range of practical applications in JavaScript programming. Here are some scenarios where it can be particularly beneficial.
Error Handling
When performing calculations that may result in undefined values, using NEGATIVE_INFINITY can help prevent errors. For instance, if a function is expected to return the smallest number but encounters an error, returning NEGATIVE_INFINITY can signal that something went wrong without crashing the application.
Setting Boundaries
In algorithms that require determining limits, such as finding the highest or lowest score in a game, initializing variables to NEGATIVE_INFINITY ensures that any valid score will replace it. This technique is common in sorting and searching algorithms.
Mathematical Operations
NEGATIVE_INFINITY can also be useful in mathematical computations. For example, when calculating averages or sums, it can serve as a baseline to compare against, ensuring that your calculations remain valid even with extreme values.
Conclusion
In conclusion, NEGATIVE_INFINITY is a unique and valuable feature in JavaScript that helps developers manage numerical boundaries effectively. By understanding its applications, you can write more robust and error-resistant code. Whether you are handling mathematical operations, setting boundaries, or managing error states, NEGATIVE_INFINITY provides a reliable solution. Embrace this concept, and you’ll find it enhances your JavaScript programming skills significantly.
FAQ
-
What is NEGATIVE_INFINITY in JavaScript?
NEGATIVE_INFINITY is a predefined value in JavaScript that represents the lowest possible number, less than any finite number. -
How is NEGATIVE_INFINITY used in calculations?
It is often the result of dividing a negative number by zero and can be used in comparisons and as a default value in functions. -
Can NEGATIVE_INFINITY be compared to other numbers?
Yes, NEGATIVE_INFINITY can be compared to any number, and it will always be less than any finite number. -
How can I handle errors using NEGATIVE_INFINITY?
You can return NEGATIVE_INFINITY in functions when an error occurs, signaling that something went wrong without crashing the application. -
Is NEGATIVE_INFINITY consistent across all browsers?
Yes, NEGATIVE_INFINITY behaves consistently across all JavaScript environments and browsers.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn