Mouseover Event in JavaScript
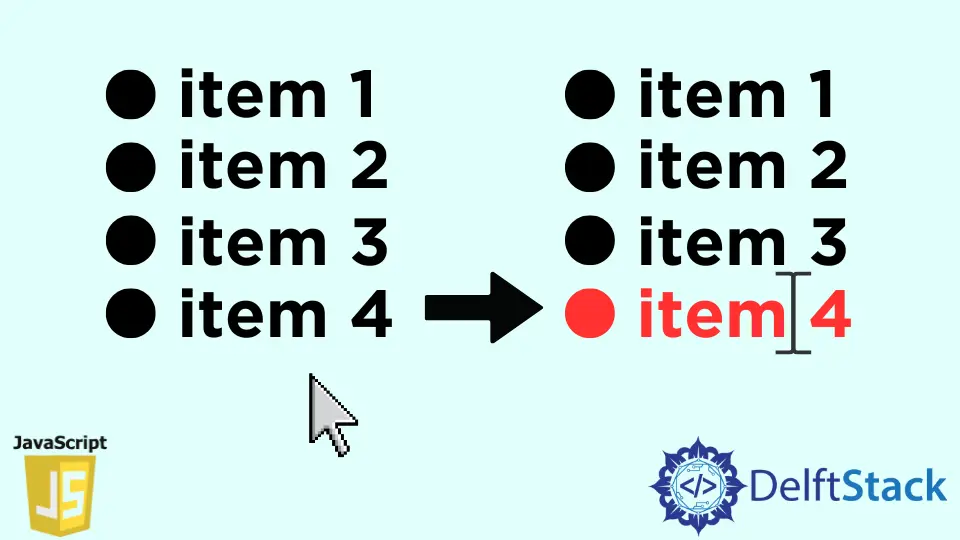
The mouseover event occurs when a pointing device moves the cursor toward an element in a webpage.
In this tutorial, we will implement the mouseover event using JavaScript.
For this, we can use the addEventListener()
to add the required mouseover event over the required element.
Check the example below.
- HTML
<ul id="example">
<li>item 1</li>
<li>item 2</li>
<li>item 3</li>
</ul>
- JavaScript
let test = document.getElementById('example');
test.addEventListener('mouseover', function(event) {
// highlight the mouseover target
event.target.style.color = 'red';
}, false);
test.addEventListener('mouseout', function(event) {
// highlight the mouseout target
event.target.style.color = 'black';
}, false);
In the above code, we retrieve the required element over which we wish to move the cursor using the getElementById()
function. We then proceed to apply the addEventListener()
method to this element, which attaches a given event handler to this element.
When the provided event is sent to the target, the EventTarget
method addEventListener()
creates a function that will be invoked. Here, mouseover
and mouseout
methods are invoked, respectively.
The mouseover
event moves the cursor to the given element. The mouseout
event moves the cursor away from this element.
The event.target.style.color
property specifies the color on invoking of the specific target methods such as mouseover
or mouseout
.