mailto in JavaScript
- Using Mailto Links in HTML
- Creating Mailto Links Dynamically with JavaScript
- Using Mailto with Predefined CC and BCC
- Conclusion
- FAQ
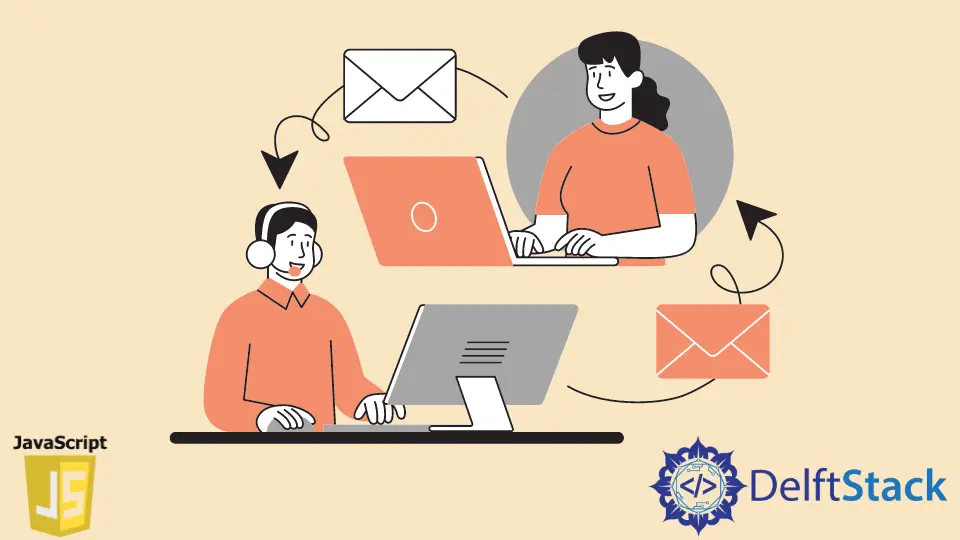
JavaScript is a versatile language that can do many things, including creating links that open email clients. One of the simplest yet effective ways to achieve this is through the use of the mailto
function. This feature allows developers to create hyperlinks that, when clicked, automatically open the user’s default email application with pre-filled information such as the recipient’s email address, subject line, and body text. Whether you’re building a contact page or a feedback form, understanding how to implement mailto
in JavaScript can enhance user experience significantly.
In this article, we’ll explore the various methods to use the mailto
function in JavaScript, complete with code examples and detailed explanations.
Using Mailto Links in HTML
The most straightforward way to implement the mailto
function is by using HTML anchor tags. This method is simple and effective for most use cases. You can create a clickable link that prompts the user’s email client to open with pre-defined fields.
Here’s how you can do it:
<a href="mailto:example@example.com?subject=Hello&body=I%20would%20like%20to%20know%20more%20about%20your%20services.">Send Email</a>
In this example, when the user clicks on the link, their email client will open with the “To” field filled with “example@example.com”. The subject line will read “Hello”, and the body will contain “I would like to know more about your services.” The %20
in the URL represents spaces, which need to be URL-encoded.
This method is particularly useful for static websites where you want to provide users with a quick way to contact you. However, it lacks flexibility since the link must be hardcoded into the HTML.
Creating Mailto Links Dynamically with JavaScript
If you want to generate mailto
links dynamically based on user input or other conditions, JavaScript can help you achieve this. By using JavaScript, you can create a more interactive experience for your users.
Here’s a simple example:
<input type="text" id="email" placeholder="Enter your email">
<input type="text" id="subject" placeholder="Enter subject">
<textarea id="body" placeholder="Enter your message"></textarea>
<button id="sendEmail">Send Email</button>
<script>
document.getElementById('sendEmail').onclick = function() {
const email = document.getElementById('email').value;
const subject = document.getElementById('subject').value;
const body = document.getElementById('body').value;
const mailtoLink = `mailto:${email}?subject=${encodeURIComponent(subject)}&body=${encodeURIComponent(body)}`;
window.location.href = mailtoLink;
};
</script>
Output:
Opens the user's default email client with the specified recipient, subject, and body based on user input.
In this example, we have three input fields for the user to fill out: their email address, the subject of the email, and the message body. When the user clicks the “Send Email” button, the JavaScript code constructs a mailto
link using the values from the input fields. The encodeURIComponent
function is used to ensure that any special characters in the subject or body are properly encoded for a URL.
This method is particularly useful for contact forms, as it allows for user-generated content. However, keep in mind that the user must have a default email client set up for this to work effectively.
Using Mailto with Predefined CC and BCC
In some cases, you may want to include additional recipients in your email, either in the CC (Carbon Copy) or BCC (Blind Carbon Copy) fields. Fortunately, the mailto
function allows you to do this quite easily.
Here’s how you can implement it:
<a href="mailto:example@example.com?cc=cc@example.com&bcc=bcc@example.com&subject=Hello&body=This%20is%20a%20test%20email.">Send Email</a>
In this example, the mailto
link includes both a CC and a BCC field. When the user clicks on the link, their email client will open with “example@example.com” in the “To” field, “cc@example.com” in the CC field, and “bcc@example.com” in the BCC field. The subject and body fields are also pre-filled.
This method is particularly useful for sending emails to multiple recipients while keeping some addresses hidden from others. However, as with the previous methods, the user must have a functional email client for it to work.
Conclusion
Understanding how to use the mailto
function in JavaScript can significantly enhance the interactivity of your web applications. Whether you’re using static HTML links or generating dynamic links with JavaScript, the mailto
function provides a simple yet effective way to facilitate email communication. By implementing these techniques, you can create a more user-friendly experience that encourages visitors to reach out easily. So go ahead, incorporate these methods into your projects, and watch your user engagement soar!
FAQ
-
what is the mailto function in JavaScript?
The mailto function allows you to create links that open the user’s default email client with pre-filled recipient, subject, and body fields. -
can I use mailto links for multiple recipients?
Yes, you can include multiple recipients by using CC and BCC fields in your mailto link. -
does the mailto function work on all browsers?
The mailto function is widely supported across all major web browsers, but it requires the user to have a default email client configured. -
can I customize the subject and body of the email?
Yes, you can customize both the subject and body by including them in the mailto link.
- is there a limit to the length of the mailto link?
While there is no strict limit, very long mailto links may not work correctly due to browser limitations on URL length.