How to Load JSON File in JavaScript
-
Use the
require()
Function to Load JSON Files in JavaScript -
Use the
fetch()
Function to Load JSON Files in JavaScript
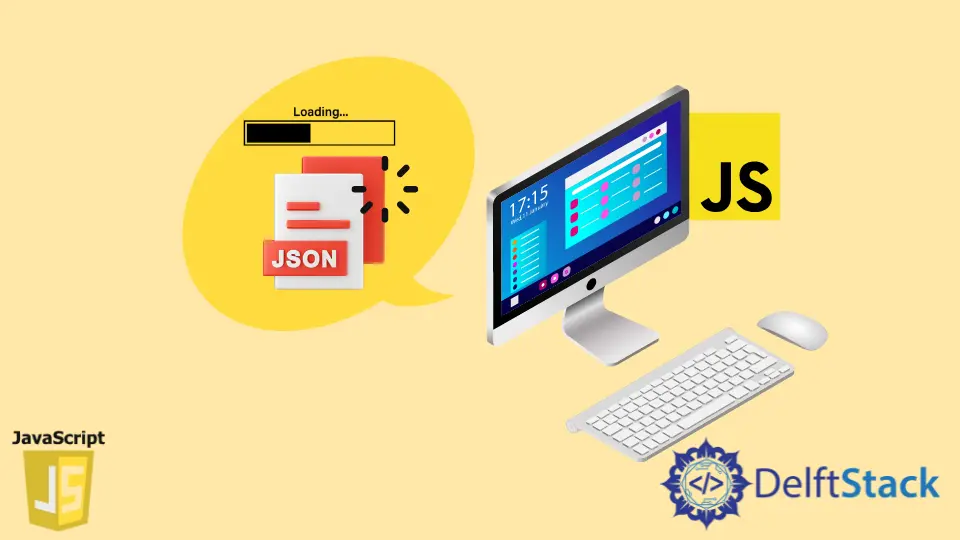
JSON stands for JavaScript Object Notion
. It is used for sharing data between servers and clients. JavaScript allows us to load local JSON.
We will discuss how to load JSON files in this article.
We will read the following JSON file.
"Students" : [
{
"firstName":"Ram","lastName":"Sharma"
}
]
}
Use the require()
Function to Load JSON Files in JavaScript
In JavaScript, we can use the require()
method to load files and modules. This takes the path of the local file where it has been saved. With the help of the console.log()
function, it loads the data in the server and displays it.
For example,
const jsonData = require('./students.json');
console.log(jsonData);
Output:
"Students" : [
{
"firstName":"Ram","lastName":"Sharma"
}
]
}
Use the fetch()
Function to Load JSON Files in JavaScript
This function fetches the file from the path where it is saved and then returns the file as the response in the console.data
. This function is only suitable for working in the web-based environment as the fetch API works only in that environment.
After reading the file, we parse the data using json()
function and display it.
The below code demonstrates the above function.
fetch('./students.json')
.then(response => {
return response.json();
})
.then(jsondata => console.log(jsondata));
Output:
"Students" : [
{
"firstName":"Ram","lastName":"Sharma"
}
]
}