How to Load an External HTML File Using JavaScript
-
Use JavaScript
fetch()
Method to Load an External HTML File -
Use jQuery
load()
Method to Load an External HTML File
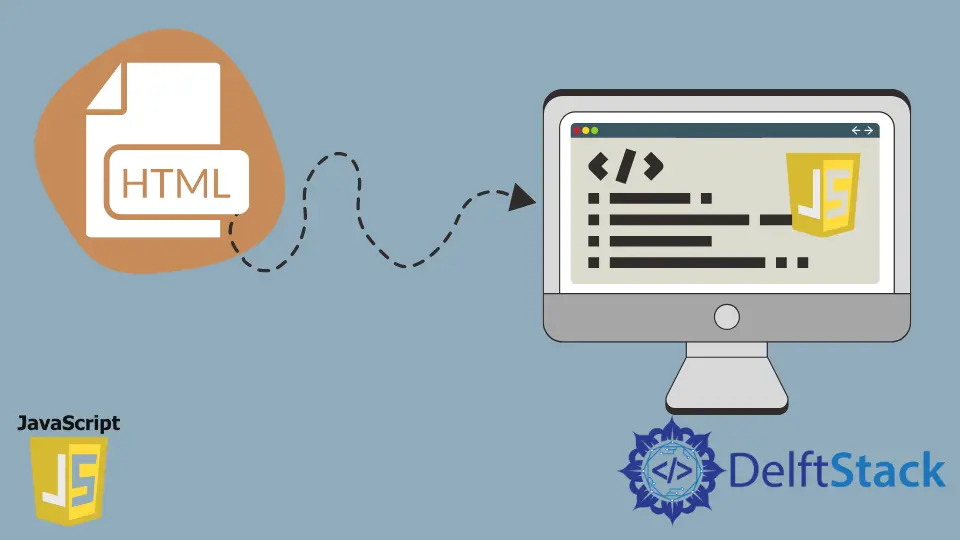
Sometimes, we must load an external HTML file into another HTML file using JavaScript or jQuery, depending on project requirements. This tutorial exemplifies how to load an external HTML file using JavaScript and jQuery.
Use JavaScript fetch()
Method to Load an External HTML File
HTML Code (home.html
):
<!DOCTYPE html>
<html lang="en">
<head>
<title>Home Page</title>
</head>
<body>
<p>This content is loaded from the home page</p>
</body>
</html>
HTML Code (index.html
):
<!DOCTYPE html>
<html lang="en">
<head>
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js">
</script>
<script src="./script.js"></script>
<link rel="stylesheet" href="./styles.css">
</head>
<body>
<button onclick="loadHTML()">
Click to load content from home.html file
</button>
<div id="homePage">
<p>The content from Home Page will be displayed here</p>
</div>
</body>
</html>
CSS Code (styles.css
):
div{
border: 2px solid rgb(255, 0, 0);
width: fit-content;
margin: 5px;
padding: 2px 20px;
cursor: pointer;
}
button{
margin: 5px;
padding: 2px 20px;
}
p{
font-size: 14px;
}
JavaScript Code (script.js
):
function loadHTML() {
fetch('home.html')
.then(response => response.text())
.then(text => document.getElementById('homePage').innerHTML = text);
}
Output:
The fetch()
function requests the server to load data on various web pages.
We use the fetch()
function to fetch the home.html
file from localhost. The fetch()
method returns a Promise
.
Further, the Response
interface’s text()
method is used, which accepts the Response
stream, reads it and returns a Promise
which solves with the String
. Remember, the Response
is decoded by using UTF-8.
After that, we get the element whose id is homePage
by using getElementById()
method and replace its inner HTML via .innerHTML
property with the text
.
Use jQuery load()
Method to Load an External HTML File
HTML Code (home.html
):
<!DOCTYPE html>
<html lang="en">
<head>
<title>Home Page</title>
</head>
<body>
<p>This content is loaded from the home page</p>
</body>
</html>
HTML Code (index.html
):
<!DOCTYPE html>
<html lang="en">
<head>
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js">
</script>
<script src="./script.js"></script>
<link rel="stylesheet" href="./styles.css">
</head>
<body>
<div id="homePage">
Home Page
<p>Click to load content from <b>home.html</b> file</p>
</div>
</body>
</html>
CSS Code (styles.css
):
div{
border: 2px solid rgb(255, 0, 0);
width: fit-content;
margin: 20px auto;
padding: 2px 20px;
cursor: pointer;
}
p{
font-size: 14px;
}
JavaScript Code (script.js
):
$(document).ready(function() {
$('#homePage').click(function() {
$(this).load('home.html');
});
});
Output:
The ready()
method examines whether the file is completely ready or not. This event only occurs when Document Object Model has been fully loaded.
The load()
method loads the information (data) from the server and gets the data returned by the server into the specified element.
We use the ready()
method to ensure that the DOM is fully ready before further operations. The home.html
file will be loaded using the load()
method.