JavaScript Lambda Function
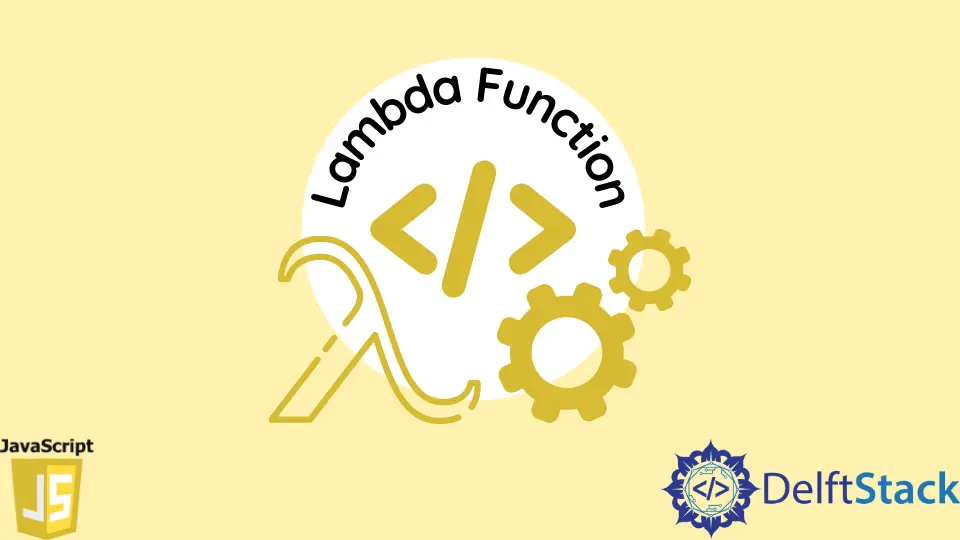
This tutorial will teach you about Lambda Expressions in JavaScript.
The Lambda Expression in JavaScript
Lambda expressions are concepts that allow a function to be treated as data. Everything in JavaScript may be handled as an object, implying that a function can be sent as a parameter to another function and received as a return value from the called function.
The following is an example of a Lambda expression.
Example Code:
function learnLambda(array, funct) {
let output = '';
for (const value of array) {
output += funct(value) + ' ';
}
console.log(output);
}
const array = [5, 6, 7, 8, 9];
const sqqr = (value) => value ** 2;
learnLambda(array, sqqr);
Output:
"25 36 49 64 81"
Functional programming has the benefit of allowing us to define pure functions in our code. A refined process always delivers the same output when given the same input.
We take the value and square it. Whatever occurs outside does not affect this.
The most noticeable difference between Lambda and anonymous functions in JavaScript is that Lambda functions may be named. This naming may help debug.
Example Code:
function learnLambda(array, funct) {
let output = '';
for (const value of array) {
output += funct(value) + ' ';
}
console.log(output);
}
const array = [5, 6, 7, 8, 9];
learnLambda(array, function sqqr(value) {
return value ** 2;
});
Output:
"25 36 49 64 81"
This demonstrates that a Lambda function is not always anonymous and that an anonymous function is not always a lambda expression if it is not handed around like data.
Usually, these two constructs are used interchangeably, and distinguishing between them may be as difficult as splitting hairs in specific programming languages. Understanding the fundamental ideas around a build and using it to achieve your aims is the most crucial component of programming, and understanding the literature and effectively applying it enhances code quality.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn