How to Pretty Print JSON in JavaScript
-
Use
JSON.stringify()
to Pretty Print JSON in JavaScript - Highlight the JSON Syntax
-
Use
JSON.parse()
to Pretty Print JSON in JavaScript - Use a JSON Array
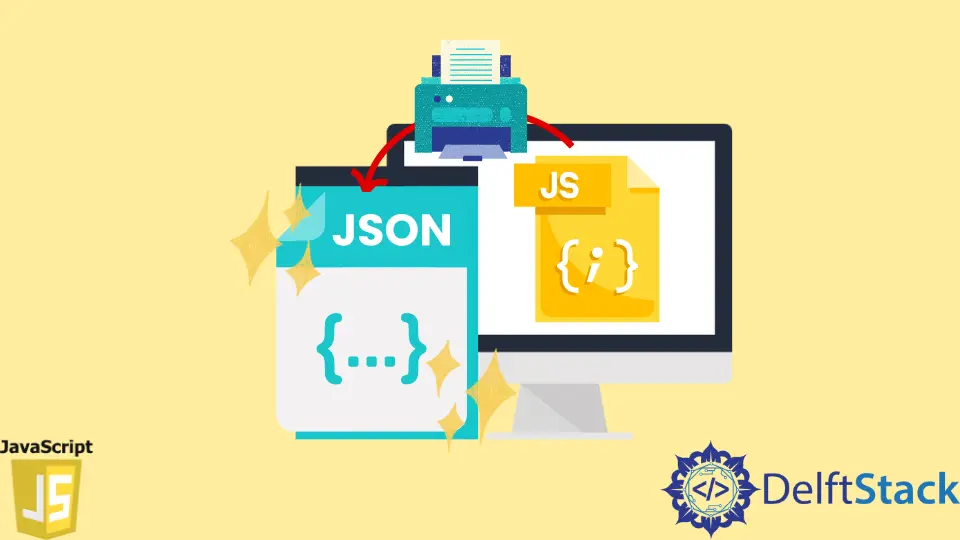
This article outlines how to pretty print JSON in JavaScript.
Use JSON.stringify()
to Pretty Print JSON in JavaScript
The JSON.stringify()
argument allows the content to be displayed in pretty print and allows the user to set the spacing that suits their readability.
You can do so by setting a string variable as follows.
var prettyString = JSON.stringify(obj, null, 2); // spacing level = 1.5
Highlight the JSON Syntax
If you need to highlight a JSON syntax, you need to create a function and include regex code.
function prettyString(json) {
if (typeof json != 'string') {
json = JSON.stringify(json, undefined, 2);
}
json =
json.replace(/&/g, '&').replace(/</g, '<').replace(/>/g, '>');
return json.replace(
/("(\\u[a-zA-Z0-9]{4}|\\[^u]|[^\\"])*"(\s*:)?|\b(true|false|null)\b|-?\d+(?:\.\d*)?(?:[eE][+\-]?\d+)?)/g,
function(match) {
var str1 = 'number';
if (/^"/.test(match)) {
if (/:$/.test(match)) {
str1 = 'key';
} else {
str1 = 'string';
}
} else if (/true|false/.test(match)) {
str1 = 'boolean';
} else if (/null/.test(match)) {
str1 = 'null';
}
return '<span class="' + str1 + '">' + match + '</span>';
});
}
Use JSON.parse()
to Pretty Print JSON in JavaScript
The method above uses json.stringify()
and works great on JSON objects, but not on JSON strings. To give a pretty print of a JSON string, you will need to convert it to an object first.
var string1 = '{"program":"code"}';
var prettyPrint = JSON.stringify(JSON.parse(string1), null, 2);
Make sure to wrap the string in the HTML tags <pre>
and </pre>
so it can display properly.
Use a JSON Array
Alternatively, you can use a JSON array in JavaScript. It is simple and does not need extra computation, which could slow down the program, especially when being repeated several times.
For instance:
JSON.stringify(jsonobj, null, '\t')
This should also be used in the <pre>
and </pre>
tags.