How to Write a Multiline String in JavaScript
- Concatenate Multiple Lines in JavaScript
-
Use the
\
Backslash Character to Escape the Literal Newlines - Use Template Literals to Create Mutliline String in JavaScript
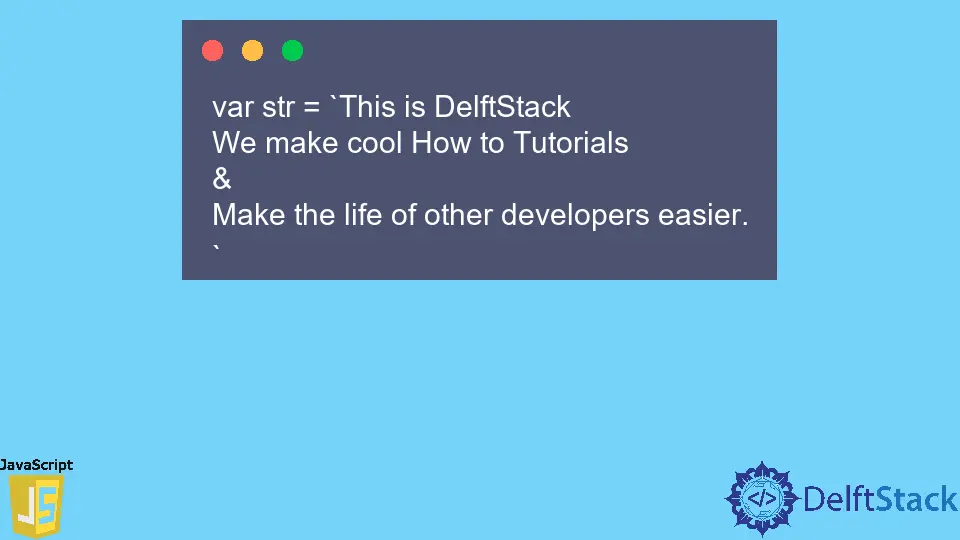
This tutorial teaches how to write a multiline string in JavaScript. In the pre ES6 era, there was no direct support for multiline strings in JavaScript. There are several ways to achieve this, pre ES6 ways which were not so good, and the ES6 way, the syntactic sugar way. We will cover all these methods one by one.
Concatenate Multiple Lines in JavaScript
We may break the string into multiple substrings and then use the +
sign to concatenate them together to get the complete single string. In this way we achieve dividing strings into multiple lines and putting them together in one string at the same time.
const str = 'This is DelftStack' +
' We make cool How to Tutorials' +
' &' +
' Make the life of other developers easier.';
Output:
"This is DelftStack We make cool How to Tutorials & Make the life of other developers easier."
It was simplest and the most promising way to write multiline strings before template literals were introduced but this method can not output the formed string as multiline strings and that has to be achieved by appending a \n
at the end of every line.
Use the \
Backslash Character to Escape the Literal Newlines
We can add a backslash at the end of every line to make a multiline string inside double/single quotes, as this character helps us to escape the newline character.
const str = 'This is DelftStack \
We make cool How to Tutorials \
& \
Make the life of other developers easier.';
Output:
"This is DelftStack We make cool How to Tutorials & Make the life of other developers easier."
So we wrote the string broken across multiple lines yet got the united string together which helps us to achieve both our targets, the correct displaying output, and readability of code. But it is not always desired we may want strings that are actually split across multiple lines even when displayed. This is achieved by using template literals in ES6.
Use Template Literals to Create Mutliline String in JavaScript
Template literal is the new way introduced by ES6 that helps us to write multiline strings with the help of backticks (this character ` is called backtick). It is by far the best solution as it not only allows us to write multiline strings it also prints them in the exact same fashion which was not possible with any of the earlier methods.
var str = `This is DelftStack
We make cool How to Tutorials
&
Make the life of other developers easier.
`
Output:
This is DelftStack
We make cool How to Tutorials
&
Make the life of other developers easier.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn