JavaScript Z Index
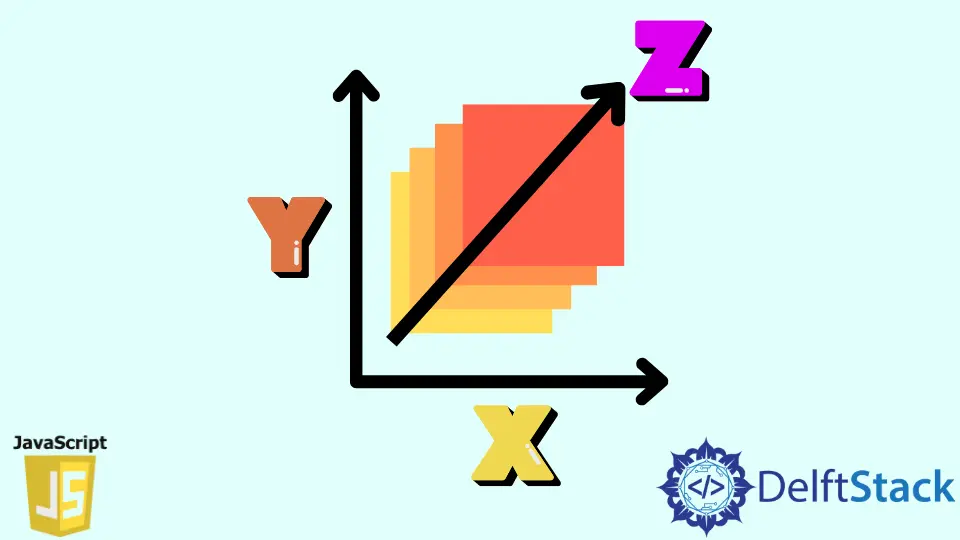
The z index
property overlaps the HTML elements and returns or sets explicitly positioned elements’ stack order.
Stack order means the element’s position on the z-axis, also called stack level.
Element with higher stack order will be in front of the lower stack order.
How Does Z Index
Works in JavaScript
We use dimensions to look at every object/item completely and determine its size. Therefore, we use the z index
to render the HTML element along the z-axis.
For instance, we have four boxes of the same size but have different colors. If we want to see all of them simultaneously, it looks as follows 2D picture, having X and Y
dimensions.
What if we look at the same picture in the Z dimension? It looks as follows 3D image, having X, Y and Z
dimensions.
How to Use JavaScript Z Index
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Z Index</title>
</head>
<body>
<center>
<h1>Learning JavaScript Z Index</h1>
<img id="my_image" src="https://images.pexels.com/photos/10970094/pexels-
photo-10970094.jpeg?auto=compress&cs=tinysrgb&dpr=1&w=500"
width="200"
height="200">
<button onclick="stackFn()">bring the picture to the front</button>
<p>This is picture has z-index as 0.</p>
<br />
<p>Bring the picture to the front by clicking on the button.</p>
</center>
</body>
</html>
#my_image {
position: absolute;
left: 400px;
top: 120px;
z-index: -1;
}
h1,p {
color: red;
}
body {
text-align: center;
}
function stackFn() {
document.getElementById('my_image').style.zIndex = '1';
}
Output:
See, the picture comes in front as soon as we click on bring the picture to the front
. It is because we are changing its zIndex
property’s value from -1
to 1
.
Use jQuery to Create a Z Index
function stackFn() {
// css method set/returns the css property
$('#my_image').css('z-index', '1');
}
Output: