How to Write New Line in JavaScript
- Using Escape Sequences
- Using Template Literals
- Using HTML Line Breaks
- Using CSS for Spacing
- Conclusion
- FAQ
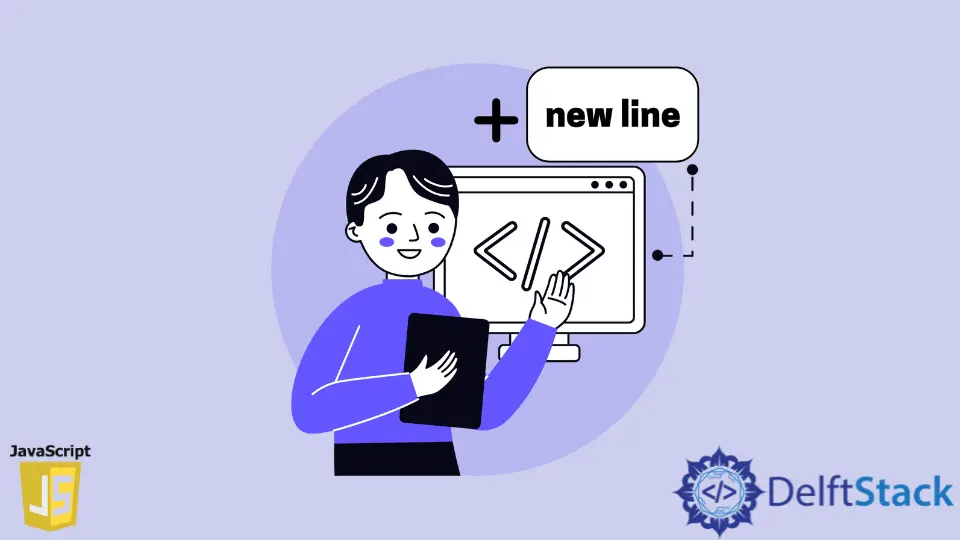
JavaScript is a versatile programming language widely used for web development. One common requirement when working with strings is the need to insert a new line. Whether you’re displaying text in a web application or processing data, knowing how to create a new line can enhance readability and improve user experience.
In this tutorial, we’ll explore various methods to write a new line in JavaScript. With practical examples and clear explanations, you’ll be equipped with the knowledge to implement new lines effectively in your projects. Let’s dive in!
Using Escape Sequences
One of the simplest ways to create a new line in JavaScript is by using escape sequences. The escape sequence for a new line is \n
. This method works well when you are dealing with string literals.
Here’s a quick example:
let message = "Hello, World!\nWelcome to JavaScript.";
console.log(message);
Output:
Hello, World!
Welcome to JavaScript.
In this code snippet, the \n
character is used within the string to indicate where the new line should occur. When the console.log()
function is called, it outputs the message with “Hello, World!” on the first line and “Welcome to JavaScript.” on the second line. This approach is straightforward and works seamlessly for simple string manipulations.
Using Template Literals
Another modern way to create new lines in JavaScript is by using template literals, which are enclosed by backticks (`
). Template literals allow for multi-line strings, making it easier to format text without the need for escape sequences.
Here’s an example:
let message = `Hello, World!
Welcome to JavaScript.`;
console.log(message);
Output:
Hello, World!
Welcome to JavaScript.
In this example, the text is defined using backticks, allowing you to write the string across multiple lines directly. This method enhances readability and is especially useful when dealing with longer strings or HTML content. Template literals not only support new lines but also allow for variable interpolation, making them a powerful feature in JavaScript.
Using HTML Line Breaks
When working with web applications, inserting new lines in HTML can be achieved using the <br>
tag. This is particularly useful when you want to display text in a browser.
Here’s how you can do it:
let message = "Hello, World!<br>Welcome to JavaScript.";
document.body.innerHTML = message;
Output:
Hello, World!
Welcome to JavaScript.
In this snippet, the <br>
tag is used to create a line break in the HTML content. By setting document.body.innerHTML
, the message is rendered in the browser with the specified line breaks. This method is essential for web development, as it allows you to control how text appears on the page without relying solely on JavaScript’s console.
Using CSS for Spacing
While JavaScript handles the logic behind displaying text, CSS can help manage the visual presentation, including line spacing. You can use CSS to add margins or padding to elements, effectively creating space between lines.
Here’s an example:
<div style="margin-bottom: 10px;">Hello, World!</div>
<div>Welcome to JavaScript.</div>
Output:
Hello, World!
Welcome to JavaScript.
In this case, we use a <div>
element to wrap the text. The style
attribute applies a margin at the bottom of the first <div>
, creating a visual separation between the two lines. This method is beneficial when you want more control over the layout and design of your web pages, allowing you to create a more visually appealing experience for users.
Conclusion
In this tutorial, we explored several methods to write a new line in JavaScript, including using escape sequences, template literals, HTML line breaks, and CSS for spacing. Each method has its own advantages and use cases, depending on the context of your project. By understanding these techniques, you can enhance the readability of your text and create more user-friendly web applications. As you continue to develop your JavaScript skills, keep these methods in mind to improve your coding practices.
FAQ
-
how do I insert a new line in a JavaScript string?
You can insert a new line in a JavaScript string using the escape sequence\n
or by using template literals with backticks. -
can I use HTML tags to create new lines in JavaScript?
Yes, you can use the HTML<br>
tag to create new lines when displaying text in a web browser. -
what are template literals in JavaScript?
Template literals are string literals enclosed by backticks (`
) that allow for multi-line strings and variable interpolation. -
how can I control line spacing in web applications?
You can control line spacing using CSS properties likemargin
andpadding
to create visual separation between elements. -
is there a difference between console output and HTML output in JavaScript?
Yes, console output is displayed in the console, while HTML output is rendered in the browser. Different methods may be used for each context.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn